Find object in list that has attribute equal to some value (that meets any condition)
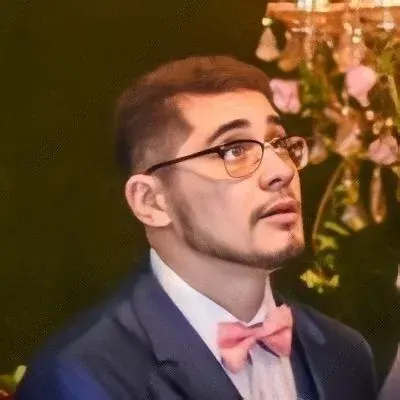
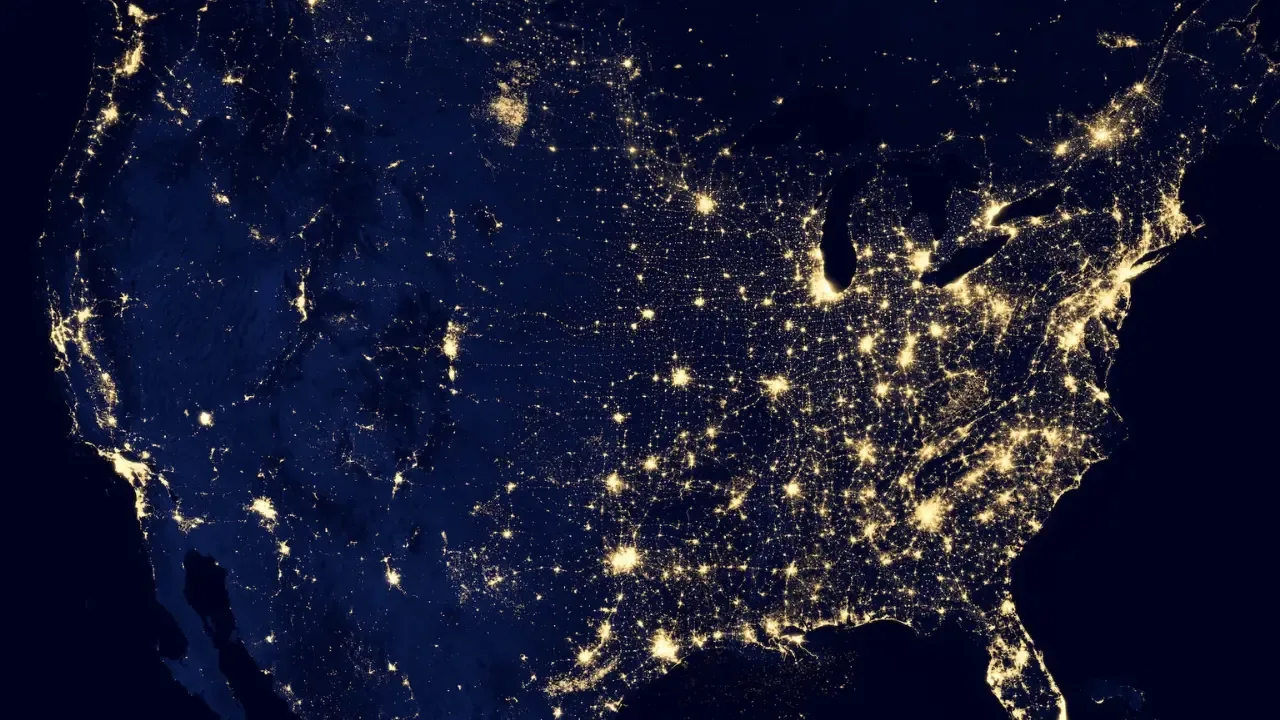
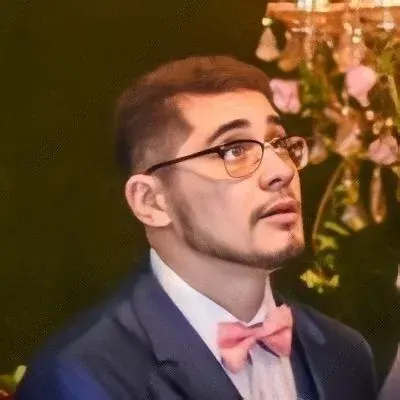
đ How to Find an Object in a List with a Specific Attribute Value đ¯
Are you tired of manually iterating through a list to find an object that matches a certain attribute value? đ Look no further! In this blog post, we will explore the best way to efficiently find that needle in the haystack. đĄ
The Problem đ¤
Let's say you have a list of objects, and you want to find the first object that has an attribute value equal to a given value. You might be tempted to iterate through the list using a for
loop, checking each object's attribute one by one. ⊠However, this approach may not be the most optimal one, especially when dealing with large lists.
class Test:
def __init__(self, value):
self.value = value
import random
value = 5
test_list = [Test(random.randint(0,100)) for x in range(1000)]
# Finding the object using a loop
for x in test_list:
if x.value == value:
print("I found it!")
break
The Solution đĄ
To tackle this problem more elegantly, we can make use of Python's powerful filter()
function, combined with lambda expressions. đ
# Finding the object using filter() and lambda
found_object = next(filter(lambda x: x.value == value, test_list), None)
if found_object:
print("I found it!")
else:
print("It's not here.")
Here's what's happening:
We use the
filter()
function to create an iterator that filters thetest_list
based on a lambda expression.The lambda expression checks if the value of the current object's attribute is equal to the given value.
Since
filter()
returns an iterator, we usenext()
to get the first object that matches the condition. We can also provide a default value (in this case,None
) if no object is found.Finally, we check if an object was found and print the appropriate message.
This solution saves us from manually iterating through the list, and it stops as soon as it finds the first matching object. âšī¸
Handling any Condition â
In the example given, we were looking for an object with an attribute equal to a specific value. However, you can easily modify the lambda expression to match any condition you desire. đ
# Example: Finding an object with an even attribute value
found_object = next(filter(lambda x: x.value % 2 == 0, test_list), None)
Feel free to adjust the lambda expression to suit your needs. The possibilities are endless! đ
Conclusion and Call-to-Action đ
Next time you need to find an object in a list based on a specific attribute value, remember to leverage Python's filter()
function with lambda expressions. It's a concise and efficient way to save time and make your code more readable. đĒ
Now, it's your turn! Have you ever encountered a situation where finding an object in a list was proving to be a headache? Share your experience and any alternative solutions you might have tried. Let's learn from each other in the comments section below! đ