Find column whose name contains a specific string
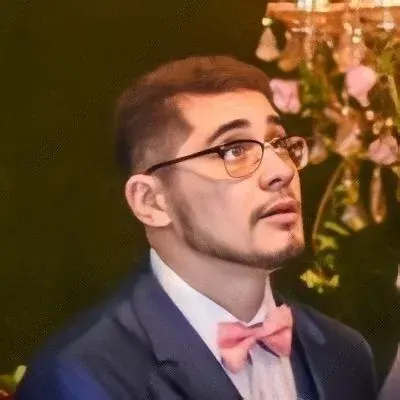
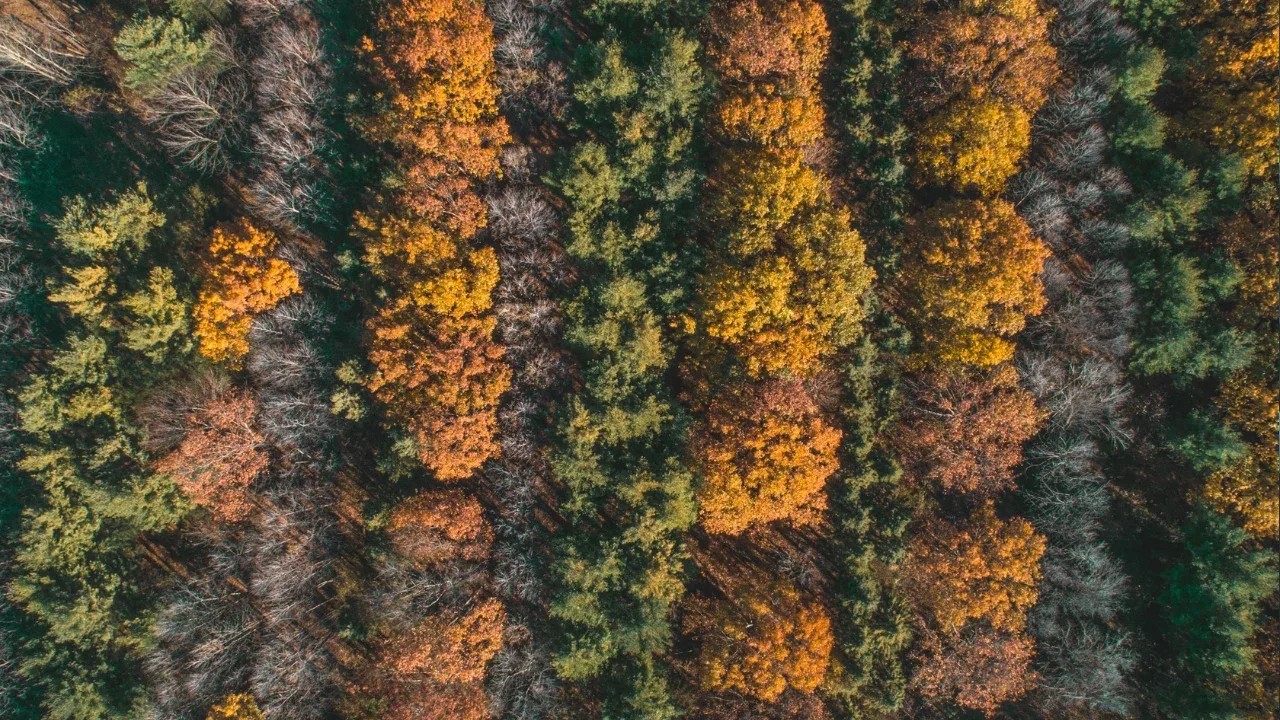
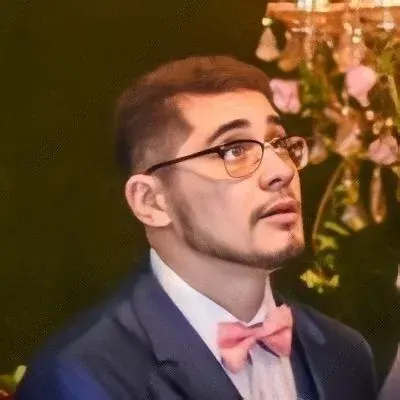
📝 Tech Tips: How to Find a Column Name containing a Specific String in Python DataFrame
Are you stuck in finding a column in your Python DataFrame that contains a specific string? Don't worry! In this guide, I will show you easy solutions to this common problem.
💡 Problem Description
Let's say you have a DataFrame with multiple columns, and you need to find the column that contains a certain string. However, the column name may not exactly match the string. For example, you are searching for the string 'spike'
, but the column names could be like 'spike-2'
, 'hey spike'
, or 'spiked-in'
. The only requirement is that the string 'spike'
is continuous within the column name.
Additionally, you want the column name to be returned as a string or a variable, so you can access it later using df['name']
or df[name]
as you normally would.
🛠️ Solution
To solve this problem, you can use Python's built-in string manipulation functions combined with the DataFrame's columns
property. Here's a step-by-step solution:
Import the necessary modules:
import pandas as pd import re
Create your DataFrame (assuming you already have one):
df = pd.DataFrame({'spike-2': [1, 2, 3], 'hey spike': [4, 5, 6], 'spiked-in': [7, 8, 9]})
Use regular expressions (regex) to find the column name containing the desired string:
string_to_find = 'spike' pattern = re.compile(f'.*{string_to_find}.*') matched_columns = [col for col in df.columns if pattern.match(col)]
Print the matched column names:
for col in matched_columns: print(col)
This will output:
spike-2 hey spike spiked-in
Store the matched column name as a string or a variable for later use:
# As a string matched_column_str = matched_columns[0] # Or as a variable matched_column_var = matched_columns[0]
You can now access the matched column using
df[matched_column_str]
ordf[matched_column_var]
.
📣 Call-to-Action
Now that you know how to find a column name containing a specific string in a Python DataFrame, go ahead and try it out in your own projects. If you have any questions or alternative solutions, leave a comment below and let's discuss!
Don't forget to share this article with your fellow Python enthusiasts who might find it helpful. Happy coding! 😄🐍