Filtering Pandas DataFrames on dates
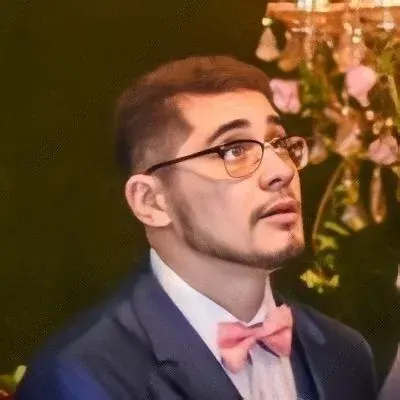
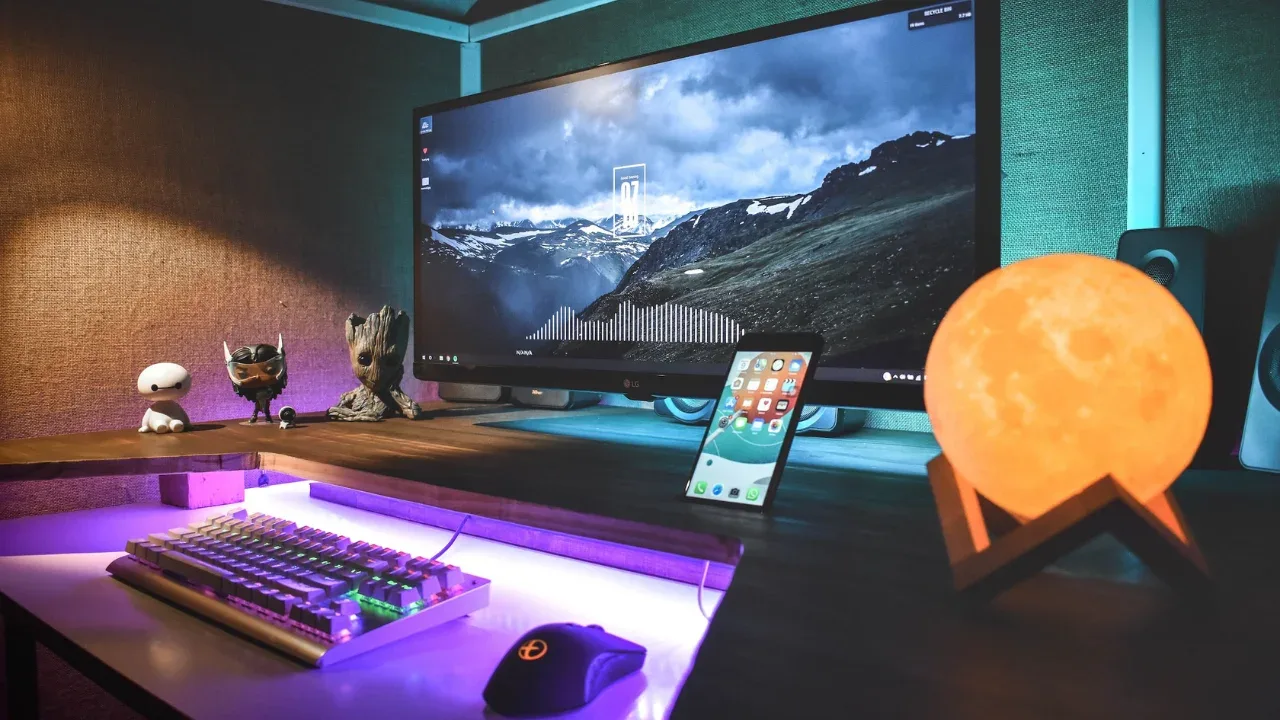
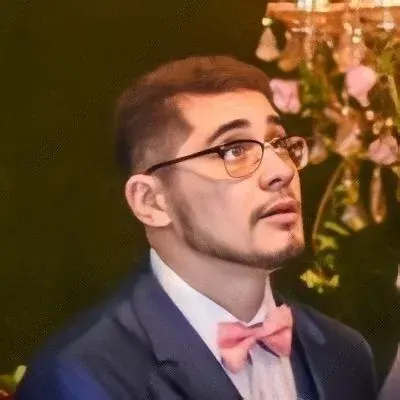
Filtering Pandas DataFrames on Dates: Retaining the Rows Within the Next Two Months π
Are you struggling to filter out rows in a Pandas DataFrame that fall outside of a specific date range? Don't worry β we've got you covered! In this blog post, we'll explore the best way to filter Pandas DataFrames based on dates, specifically retaining only the rows within the upcoming two months. Let's dive in and find the perfect solution! πͺπ
The Context π
Let's set the scene β you have a Pandas DataFrame, and one of the columns stores dates. Your goal is to filter out all the rows that have dates falling outside of the next two months. The ultimate aim is to narrow down your DataFrame solely to the rows that lie within this time frame. Seems like a tricky problem, right? Luckily, we have some neat solutions to make your life easier! ππ
Solution 1: Using Pandas to Filter by Date πΌποΈ
The most straightforward solution involves using built-in Pandas functionalities, such as pd.to_datetime
, to convert the 'date' column into a Pandas datetime
type. Once the column is in the correct format, filtering it based on the next two months becomes a breeze. Here's some code to help you get started:
import pandas as pd
# Assuming your DataFrame is called 'df'
df['date'] = pd.to_datetime(df['date']) # Convert 'date' column to datetime type
today = pd.Timestamp.today() # Get today's date
next_two_months = today + pd.DateOffset(months=2) # Get the date two months from today
filtered_df = df[df['date'] < next_two_months] # Filter out the rows outside the two-month range
In the code above, we first convert the 'date' column to the datetime type using the pd.to_datetime
function. Then, we use pd.Timestamp.today()
to retrieve today's date and pd.DateOffset(months=2)
to calculate the date two months from now. Finally, we apply the filter by creating a new DataFrame called filtered_df
that retains only the rows with dates falling within the specified range. πβ¨
Solution 2: Utilizing Boolean Indexing π‘π
As an alternative approach, we can also use Boolean indexing to filter out the rows we don't need. This involves creating a Boolean mask by comparing each date in the 'date' column against our date range criteria. Here's an example:
# Assuming your DataFrame is called 'df'
df['date'] = pd.to_datetime(df['date']) # Convert 'date' column to datetime type
today = pd.Timestamp.today() # Get today's date
next_two_months = today + pd.DateOffset(months=2) # Get the date two months from today
mask = (df['date'] < next_two_months) # Create a Boolean mask based on the specified date range
filtered_df = df.loc[mask] # Apply the mask to the original DataFrame
In this solution, we create a Boolean mask called mask
where each element represents whether the corresponding row fulfills our date range condition. By using this mask with df.loc
, we extract only the rows that meet the criteria, resulting in the desired filtered DataFrame. ππ
Time to Put Your Knowledge to the Test! β³π‘
Now that you have learned two effective ways to filter Pandas DataFrames based on dates, it's time to give it a go with your own data! Feel free to experiment and adapt these solutions to suit your specific requirements. If you encounter any issues or you have alternative solutions, we'd love to hear about them in the comments below. Let's dig into your data and make the most of it! ππ
Was this blog post helpful? Don't forget to share it with fellow data enthusiasts and join the conversation below. Happy coding! ππ©βπ»π¨βπ»