Filter pandas DataFrame by substring criteria
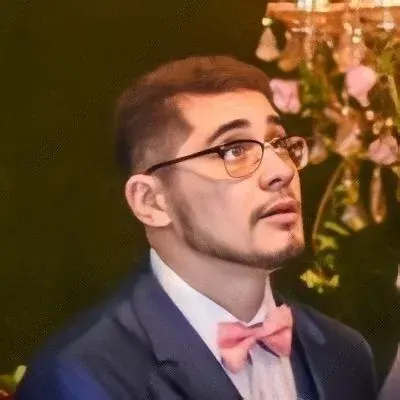
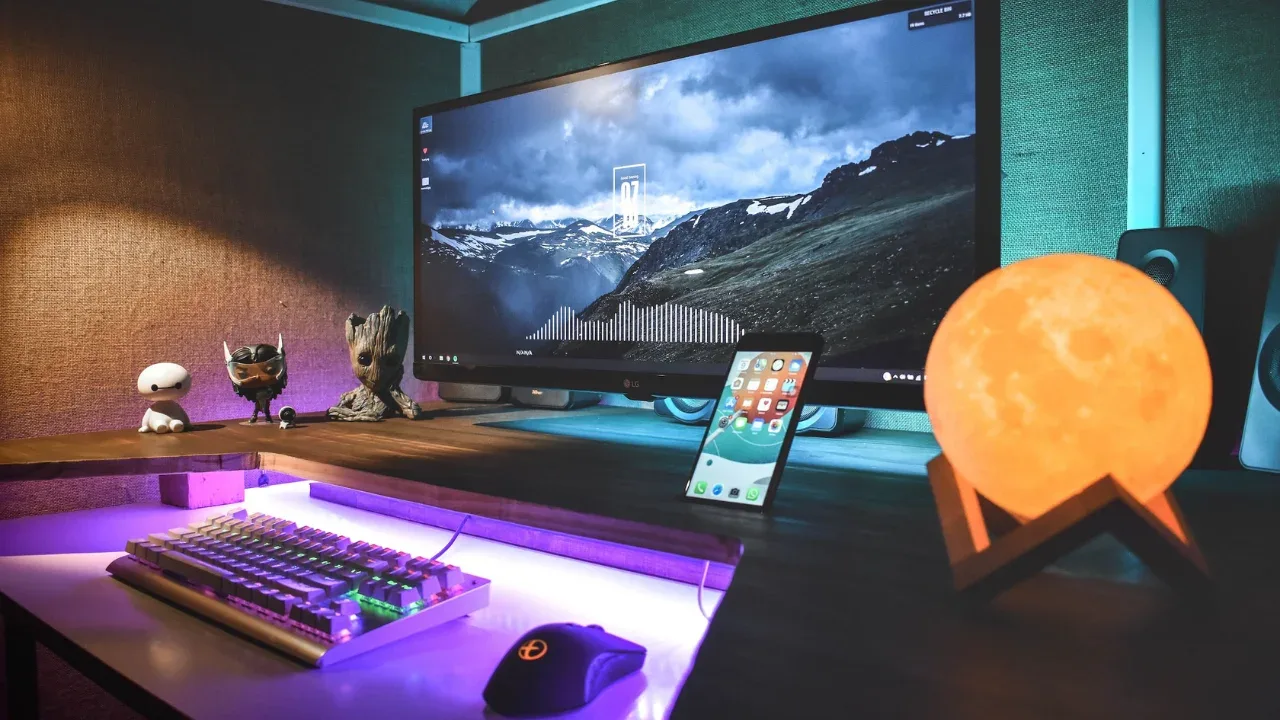
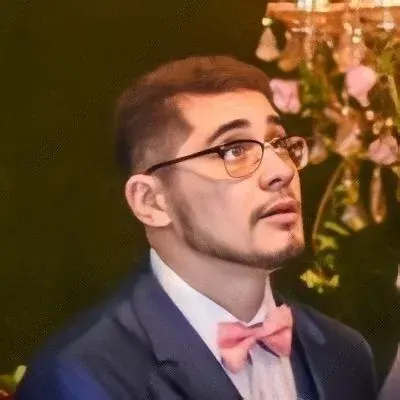
Filtering Pandas DataFrame by Substring Criteria
š Welcome to our tech blog! In this post, we will address a common issue that many Python pandas users face when trying to filter a DataFrame based on partial string matches. We understand that the syntax for exact string matching, such as df[df['A'] == "hello world"]
, is well-known, but partial string matching, like 'hello'
, can be a bit tricky. Don't worry, we've got you covered with easy solutions and practical examples!
The Problem: Selecting Rows Based on Partial String Matches
Let's say you have a pandas DataFrame with a column containing string values, and you need to select specific rows that match a particular substring. For example, you want to filter all rows where the string in the column contains the word "hello."
The Solution: Using the str.contains()
Method
To achieve this, you can use the str.contains()
method provided by pandas. This method allows you to perform partial string matching on a DataFrame column.
Here's how you can use the str.contains()
method to filter rows based on a substring match:
df[df['column_name'].str.contains('substring')]
Replace 'column_name'
with the actual name of the column you want to filter, and 'substring'
with the desired substring you want to match.
Example: Filtering a DataFrame based on a Substring Match
Let's illustrate this solution with an example. Assume we have the following DataFrame:
import pandas as pd
data = {'Name': ['John Doe', 'Alice Smith', 'Bob Johnson', 'Charlie Brown'],
'Age': [25, 30, 35, 40]}
df = pd.DataFrame(data)
Suppose we want to filter all rows where the Name
column contains the substring "John." We can achieve this by using the following code:
filtered_df = df[df['Name'].str.contains('John')]
After executing this code, filtered_df
will contain the rows that match the specified substring criteria, in this case, the row with 'John Doe'
.
Call-to-Action: Engage and Share Your Experience!
Filtering DataFrame by substring criteria can be a powerful technique to extract useful information from your data. Have you encountered any challenges when trying to perform partial string matching? Let us know in the comments below!
Share this blog post with your fellow pandas enthusiasts and help them overcome this common obstacle. š
Thank you for reading! Stay tuned for more insightful tech tips and tutorials. Happy coding! š»š„