Filter dataframe rows if value in column is in a set list of values
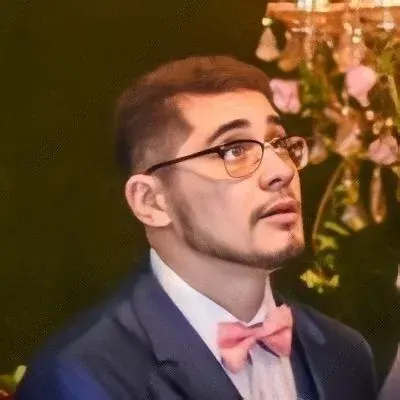
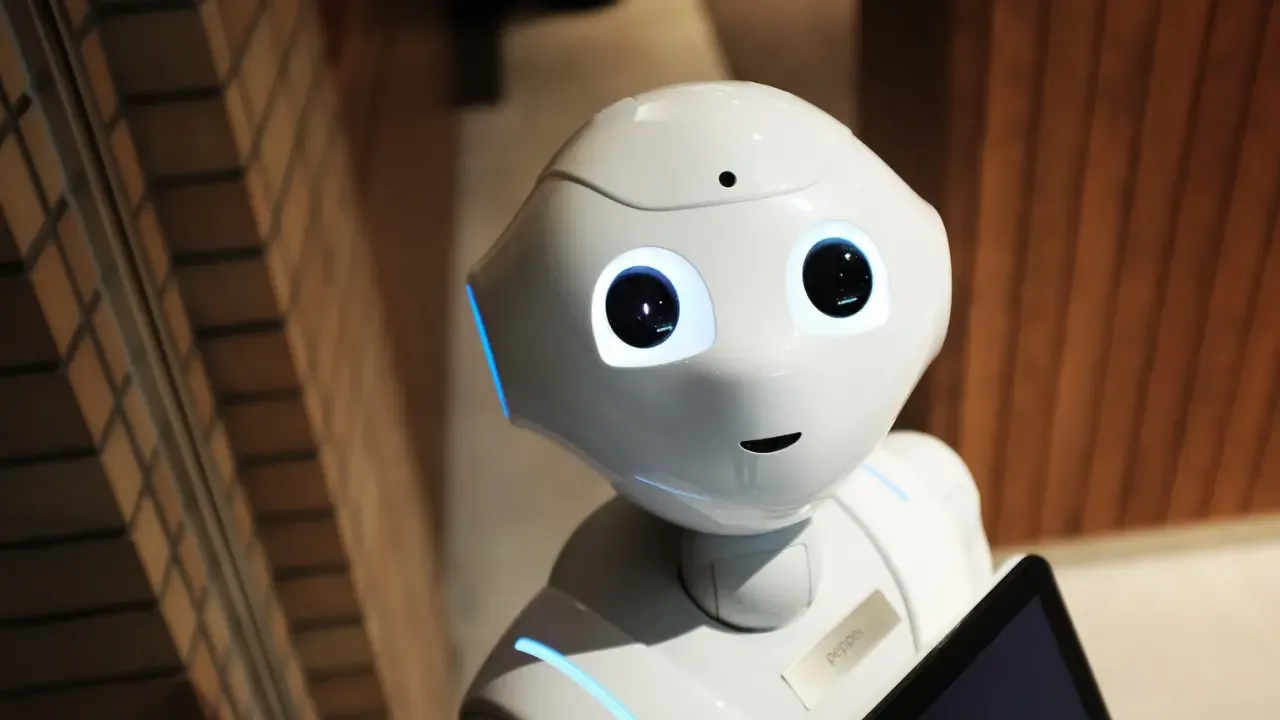
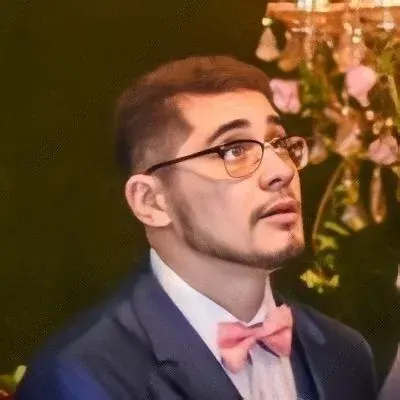
Filtering DataFrame Rows Using a List of Values in a Column
So, you have a pandas DataFrame and you want to filter the rows based on whether the values in a specific column are in a given list of values. The problem arises when trying to use the in
operator within the DataFrame filtering syntax, as it does not work in pandas. But fear not, because we have some easy and efficient solutions to your rescue! π
The Problem
Let's take a look at a simplified example. Say we have a DataFrame called rpt
, which contains information about stocks:
rpt
<class 'pandas.core.frame.DataFrame'>
MultiIndex: 47518 entries, ('000002', '20120331') to ('603366', '20091231')
Data columns:
STK_ID 47518 non-null values
STK_Name 47518 non-null values
RPT_Date 47518 non-null values
sales 47518 non-null values
You want to filter the rows in rpt
based on the values in the 'STK_ID'
column. For example, let's say you want to get all the rows where the stock ID is '600809'
. You might try doing something like this:
rpt[rpt['STK_ID'] == '600809']
And you will get a filtered DataFrame:
<class 'pandas.core.frame.DataFrame'>
MultiIndex: 25 entries, ('600809', '20120331') to ('600809', '20060331')
Data columns:
STK_ID 25 non-null values
STK_Name 25 non-null values
RPT_Date 25 non-null values
sales 25 non-null values
The Solution
Now comes the part where you want to get all the rows of multiple stocks together. Let's say you have a list of stock IDs, like ['600809', '600141', '600329']
, and you want to filter the DataFrame to only include rows with stock IDs from this list.
If you try something like this:
stk_list = ['600809', '600141', '600329']
rst = rpt[rpt['STK_ID'] in stk_list] # This doesn't work in pandas
You will encounter an error because pandas does not support using the in
operator in the DataFrame filtering syntax. But don't worry, there are a couple of simple solutions to achieve the desired result!
Solution 1: Using the isin()
Method
The isin()
method in pandas allows you to check if each element in a DataFrame column belongs to a list of values. Here's how you can use it to filter the DataFrame:
stk_list = ['600809', '600141', '600329']
rst = rpt[rpt['STK_ID'].isin(stk_list)]
Voila! π This will give you a filtered DataFrame containing only the rows with stock IDs present in the stk_list
.
Solution 2: Using the query()
Method
Another handy method in pandas is query()
, which allows you to write expressions similar to SQL queries to filter your DataFrame. Here's how you can use it:
stk_list = ['600809', '600141', '600329']
rst = rpt.query('STK_ID in @stk_list')
In this case, @stk_list
is used as a reference to the stk_list
variable defined outside the query. This way, you can efficiently achieve the desired filtering.
Calling All Stock Enthusiasts! πΌπ
Now that you have learned how to filter DataFrame rows based on a list of values in a column, it's time to put your newfound knowledge into action! Try applying these solutions to your own real-world data or explore different ways of leveraging the power of pandas. Share your experiences or any other cool tricks you come across with the pandas community. Engage with us by leaving a comment below or sharing this blog post with your fellow stock enthusiasts. Happy filtering! π