Favorite Django Tips & Features?
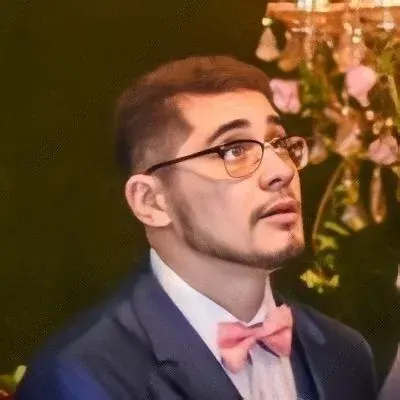
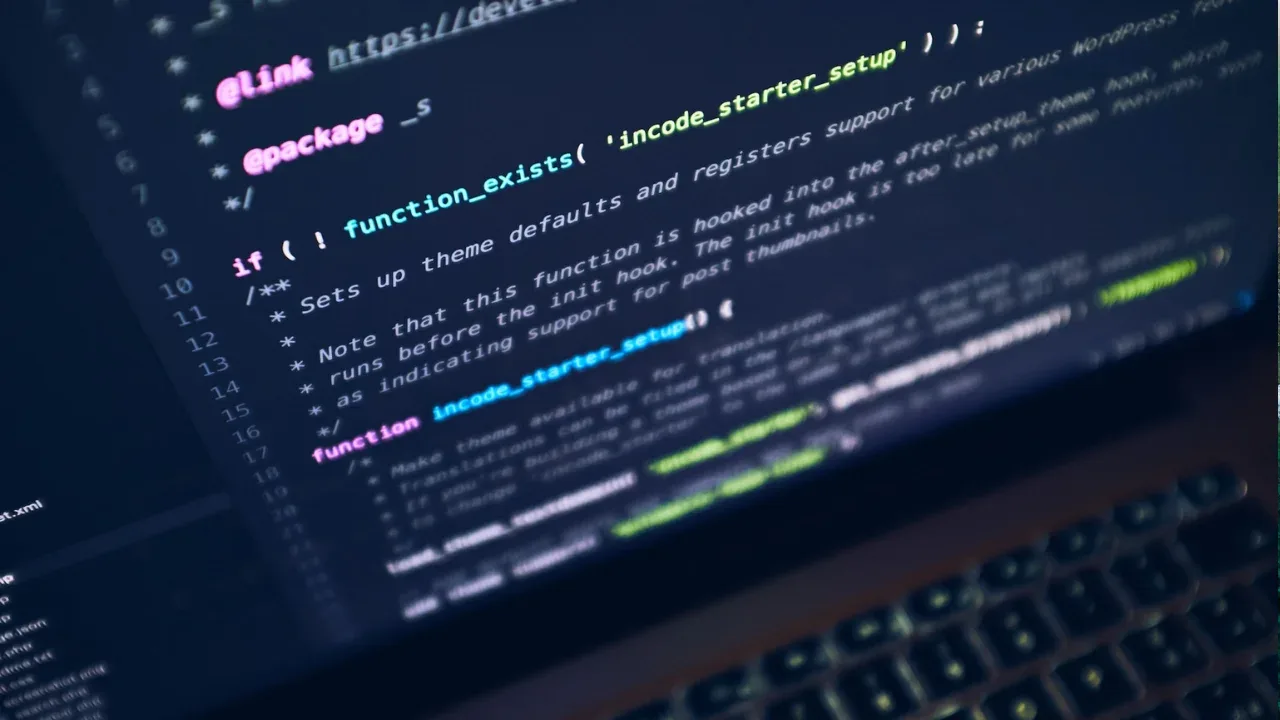
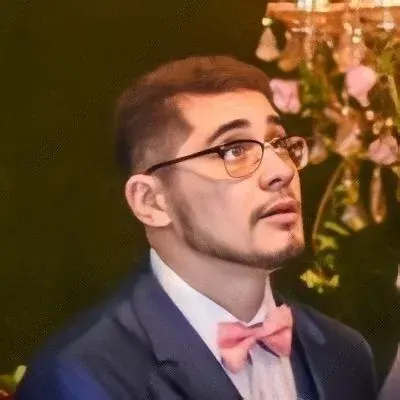
10 Amazing Django Tips and Features You Must Know! 🚀
Are you a Django developer looking for some hidden gems in this powerful web framework? Look no further! In this blog post, we will explore some of the coolest Django tips and lesser-known features that will make your development tasks easier and more enjoyable. So let's dive right in! 💻
Tip #1: Use Django Shell Plus
One of the most useful extensions for your Django project is Django Shell Plus. This tool provides an enhanced interactive shell with auto-importing of models, making it easier to work with your project's data. To install it, simply add 'django_extensions'
to your INSTALLED_APPS
and run:
python manage.py shell_plus
Now you can explore and manipulate your data with all the power of Django at your fingertips! 💪
Tip #2: Speed Up Template Development with {% include %}
If you find yourself writing repetitive blocks of code in your templates, Django's {% include %}
template tag comes to the rescue. It allows you to include code from another template, reducing duplication and making your templates cleaner and more maintainable. For example:
{% include 'partials/navbar.html' %}
{% include 'partials/footer.html' %}
Tip #3: Use Django's @property
decorator for Efficient Model Methods
In Django models, you can use the @property
decorator to create computed fields or perform complex calculations. By using this decorator, you can access these methods without needing to call them as functions. It makes your code more readable and saves you from adding unnecessary parentheses. Here's an example:
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=10, decimal_places=2)
@property
def discount_price(self):
return self.price * 0.9
Now you can access the discount price of a product directly as product.discount_price
. 🔥
Tip #4: Simplify Model Querying with get_object_or_404()
Django provides a useful shortcut method called get_object_or_404()
that saves you from writing repetitive code whenever you need to get an object from the database. If the object doesn't exist, it raises a 404 exception. Here's how you can use it:
from django.shortcuts import get_object_or_404
def product_detail(request, product_id):
product = get_object_or_404(Product, id=product_id)
return render(request, 'product_detail.html', {'product': product})
Tip #5: Boost Performance with Django's Caching Framework
If your application deals with frequently accessed data, you can significantly improve its performance by using Django's built-in caching framework. By caching database queries, rendered templates, or any other expensive computations, you can reduce the load on your server and provide a faster user experience. Check out Django's cache framework documentation to take advantage of this powerful feature. ⚡
Tip #6: Take Advantage of Django's Built-in Middleware
Django's middleware functionality allows you to modify and process requests and responses at the HTTP layer. It provides a great way to add common functionalities to your application, such as authentication, CORS handling, and request/response preprocessing. By writing custom middleware, you can seamlessly integrate these features without cluttering your views or templates.
Tip #7: Customize the Django Admin Interface
Django's admin interface is already a powerful tool, but did you know you can customize it to fit your project's needs? By making use of the ModelAdmin
class and overriding its methods, you can add filters, define custom actions, and create custom views to extend the functionality of the admin interface. Take control of your admin panel and make it truly your own!
Tip #8: Simplify Model Form Creation with Django's ModelForm
Django's ModelForm
makes it a breeze to create forms for your models. It automatically generates form fields based on the model's fields, handles validation, and provides an easy way to handle form submissions. This can save you a lot of time and effort in form handling. Here's a simple example:
from django import forms
from .models import Product
class ProductForm(forms.ModelForm):
class Meta:
model = Product
fields = ['name', 'price']
With this form, you can easily create, display, and validate product data without writing a lot of boilerplate code.
Tip #9: Leverage Django's Signals for Decoupled Applications
Django's signals framework enables you to decouple different parts of your application and respond to specific actions or events. By using signals, you can trigger actions whenever certain events occur, such as saving a model instance or deleting an object. This allows you to keep your code modular and maintainable. Learn more about signals in the Django documentation.
Tip #10: Stay Up to Date with Django Releases
Django is constantly evolving, and new features and improvements are regularly added. Stay up to date with Django releases by subscribing to the Django blog or following official Django social media channels. Keeping up with the latest updates ensures you're making the most of Django's capabilities and taking advantage of the newest features and performance improvements.
So there you have it – 10 amazing Django tips and features that will level up your development game! Which tip was your favorite or most helpful? Share your thoughts and other Django tips in the comments below! Let's learn from each other and create even better Django applications together. 🌟