Fastest way to get the first object from a queryset in django?
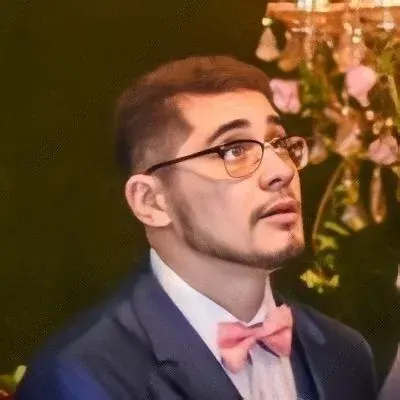
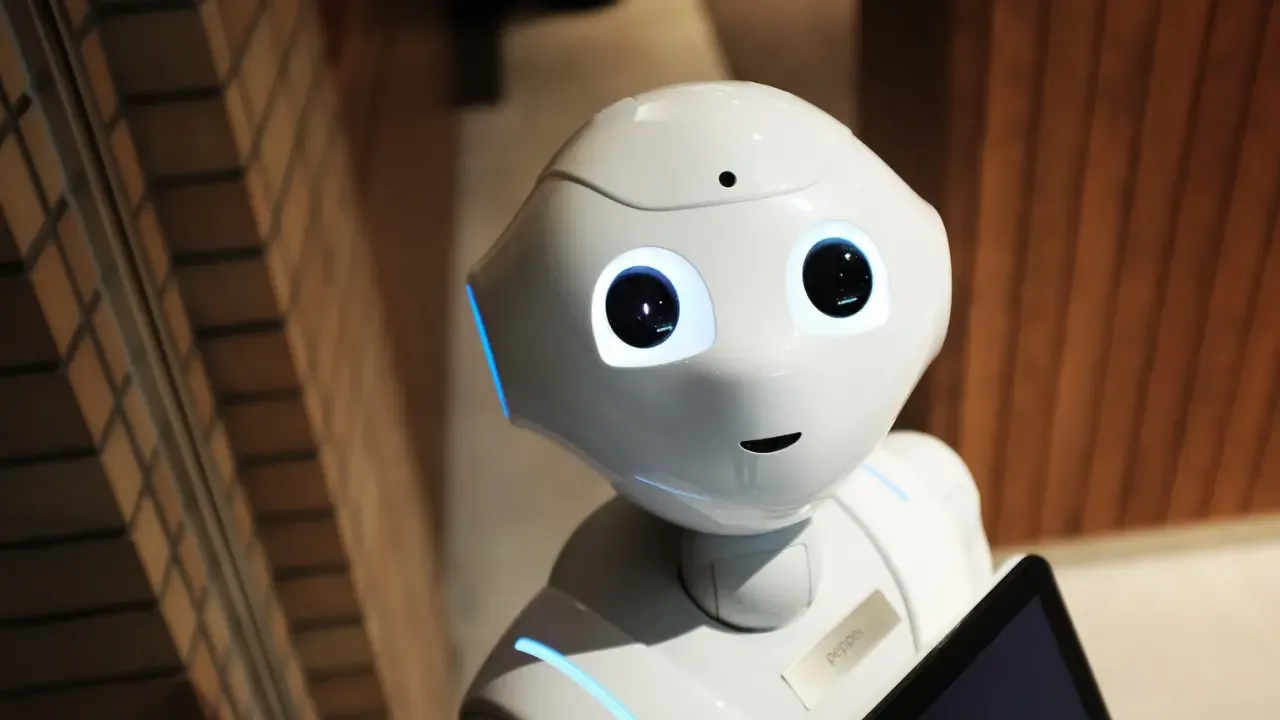
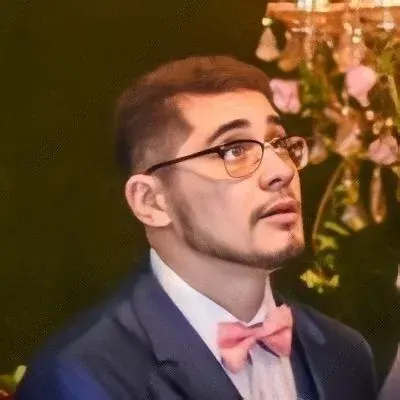
🚀 The Fastest Way to Get the First Object from a Django Queryset 🐍
Are you tired of wasting precious time and resources when trying to retrieve the first object from a queryset in Django? 🤔 Don't worry, we've got you covered! In this guide, we'll explore the different methods, discuss their performance, and provide you with the fastest solution. Let's dive in! 💪
1️⃣ Method 1: Using the count() method 📊
qs = MyModel.objects.filter(blah=blah)
if qs.count() > 0:
return qs[0]
else:
return None
This method checks the count of the queryset, but it results in TWO database calls! 😱 The first call is to retrieve the count while the second call retrieves the first object. It works, but it's not the most efficient solution.
2️⃣ Method 2: Using the len() function 📏
qs = MyModel.objects.filter(blah=blah)
if len(qs) > 0:
return qs[0]
else:
return None
This method also works, but it suffers from the same problem as the previous one - TWO database calls. While it might seem like a minor inconvenience, unnecessary database calls can add up and impact performance in larger projects. 💥
3️⃣ Method 3: Using exception handling ✨
qs = MyModel.objects.filter(blah=blah)
try:
return qs[0]
except IndexError:
return None
This method tackles the issue of multiple database calls by generating a single database call. In case the queryset is empty, it catches the IndexError
exception and returns None
. This approach works, but creating exception objects can be memory-intensive. It might not be the most efficient solution in terms of memory usage.
4️⃣ The Fastest Method: Using the first() method 🔥
Now, for the moment you've been waiting for, the fastest method to get the first object from a Django queryset in just ONE database call! 🙌
qs = MyModel.objects.filter(blah=blah)
first_obj = qs.first()
return first_obj
By using the first()
method, Django retrieves the first object from the queryset directly from the database and returns it. If the queryset is empty, it returns None
. This method is the most performant as it only requires a single database call and avoids unnecessary memory usage. 🏎️
🔔 Call-to-Action: Have you tried any of these methods? Let us know in the comments which one you found most efficient! We love hearing from you and learning about your experiences. 🗣️💬
That's all for now, folks! We hope this guide helped you find the fastest way to fetch the first object from a Django queryset. Remember, optimizing your code can lead to significant improvements in performance. Happy coding! 😄👩💻👨💻