Extracting specific selected columns to new DataFrame as a copy
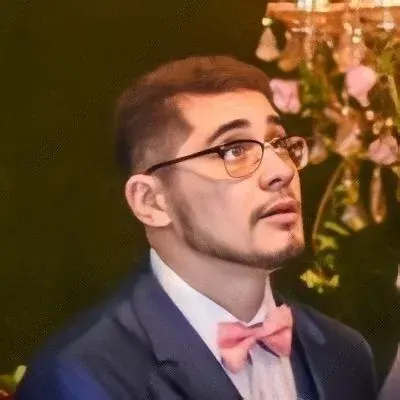
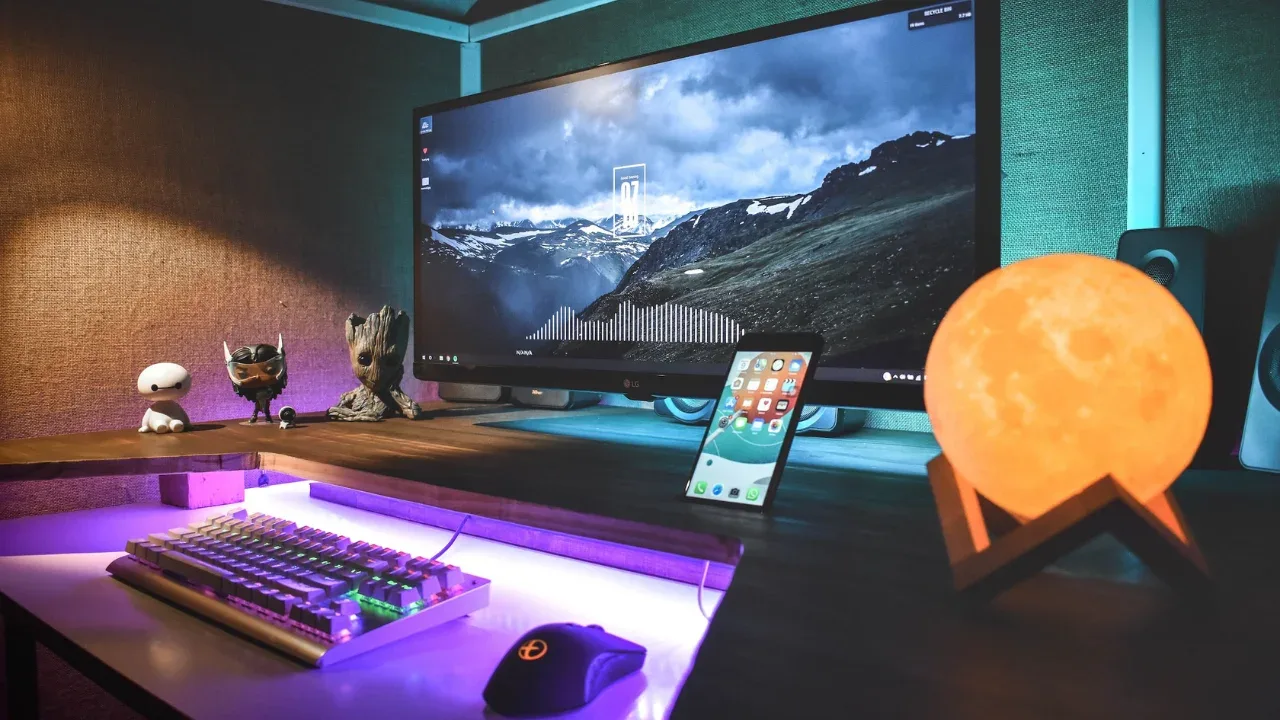
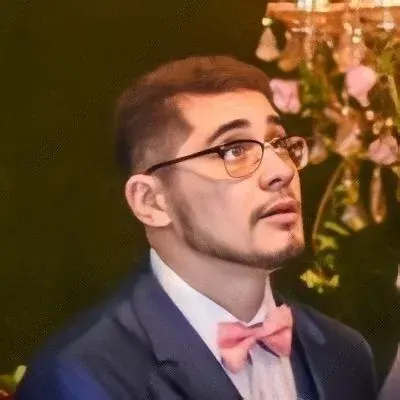
Extracting specific selected columns to new DataFrame as a copy ππ»π‘
So you have a pandas DataFrame with multiple columns, but you only need a few of those columns for further analysis or processing. You want to create a new DataFrame that contains only those selected columns. But how do you accomplish this task efficiently and in the pandas way? Let's find out!
The Initial Approach β
Before diving into the pandas way, let's take a look at the initial code provided, which raises an error:
import pandas as pd
old = pd.DataFrame({'A' : [4,5], 'B' : [10,20], 'C' : [100,50], 'D' : [-30,-50]})
new = pd.DataFrame(zip(old.A, old.C, old.D))
# raises TypeError: data argument can't be an iterator
The above code attempts to create a new DataFrame, new
, by zipping together the selected columns (A
, C
, D
) from the original DataFrame, old
. However, it raises a TypeError
stating that the data argument cannot be an iterator. Clearly, this approach is not the pandas way to achieve our goal.
The Pandas Way β
To extract specific selected columns from a pandas DataFrame and create a new DataFrame, we can use the loc
method along with slicing notation.
import pandas as pd
old = pd.DataFrame({'A' : [4,5], 'B' : [10,20], 'C' : [100,50], 'D' : [-30,-50]})
selected_columns = ['A', 'C', 'D']
new = old.loc[:, selected_columns].copy()
In the above code, we define the selected_columns
list, which contains the names of the columns we want to extract (A
, C
, D
). Then, using the loc
method, we pass :
to select all rows and selected_columns
to select the desired columns. Finally, we call the copy
method to create a new DataFrame that is independent of the original DataFrame old
.
Now, you have successfully extracted the specific selected columns (A
, C
, D
) into the new DataFrame new
π
Bonus Tip: Avoiding Copy-on-Write Pitfalls β οΈ
When dealing with large datasets, it's important to be mindful of memory usage. By default, pandas performs what is known as "copy-on-write" behavior, meaning that modifications made to a subset of a DataFrame create a copy of the subset in memory. This behavior ensures data integrity but can lead to memory inefficiencies.
To mitigate this, we explicitly use the copy
method after selecting the desired columns. This creates a true copy of the selected columns in memory, separate from the original DataFrame.
π‘ Pro Tip: If you're working with a large DataFrame and only require read-only access to the selected columns, you can use copy=False
as an optimization. However, ensure you do not modify the selected columns in such scenarios.
Share Your Thoughts π€π¬β¨
Have you ever faced difficulties extracting specific columns from a pandas DataFrame? What other pandas-related topics would you like to explore? Let's discuss and learn from each other in the comments section below!
Remember, extracting specific selected columns to a new DataFrame as a copy is a common requirement in data analysis, and now you know the pandas way to achieve it effortlessly. Start utilizing this technique today and optimize your data workflows!
π Happy coding and pandas-ing! πΌπ»
References
Stack Overflow: Extracting specific columns from a data frame
pandas documentation:
DataFrame.loc
β¨ Stay tuned for more exciting pandas tutorials on our blog! β¨