Extracting extension from filename in Python
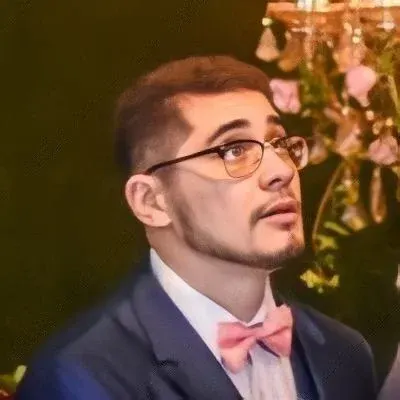
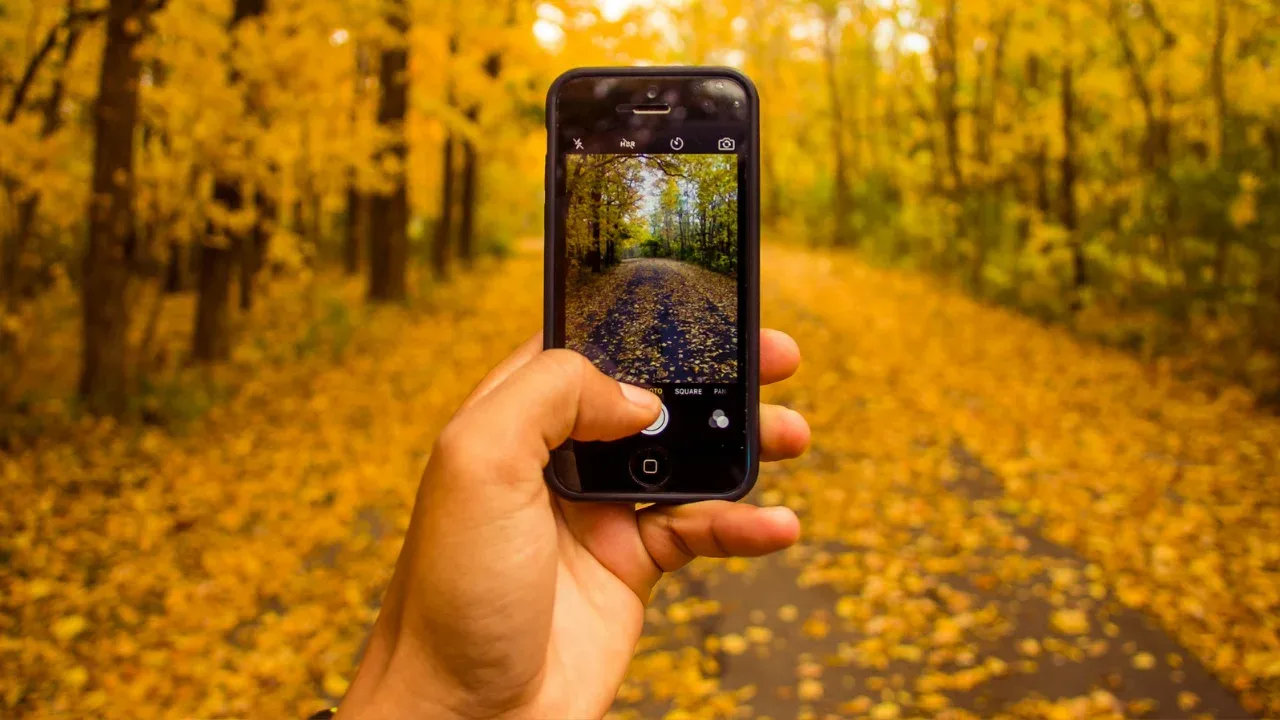
🐍 Extracting extension from filename in Python
So you have a filename and you need to extract the extension. But how do you do it in Python? 🤔 Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions to extract the extension from a filename.
The Problem: Extracting the Extension
Let's say you have a filename like "my_file.txt" and you want to extract the extension ".txt". How do you go about doing that? 🤷♂️
The Solution: Using Python's os.path
Module
Python provides a built-in module called os.path
that has several handy functions for working with file paths. One of these functions is os.path.splitext()
, which splits a file path into its root and extension parts.
Here's an example of how you can use os.path.splitext()
to extract the extension from a filename:
import os
filename = "my_file.txt"
extension = os.path.splitext(filename)[1]
print(extension) # Output: .txt
In the example above, we pass the filename
to os.path.splitext()
, which returns a tuple containing the root and extension parts. Since we only need the extension part, we access it using [1]
.
Handling Different File Path Formats
Sometimes, filenames can have multiple dots, like "my.file.name.txt". In such cases, os.path.splitext()
would consider the last dot as the start of the extension. This might not be what you want.
To handle this, you can first extract the filename from the full path using os.path.basename()
and then apply os.path.splitext()
to it. Here's an example:
import os
filepath = "/path/to/my.file.name.txt"
filename = os.path.basename(filepath)
extension = os.path.splitext(filename)[1]
print(extension) # Output: .txt
By using os.path.basename()
, we ensure that os.path.splitext()
operates on the actual filename, rather than the full path.
Going Beyond: Handling Edge Cases
While using os.path.splitext()
is generally sufficient for most cases, there may be some uncommon situations that require additional handling. For example, consider a filename like "my_file." or "my_file". In these cases, os.path.splitext()
would return an empty string as the extension. You might want to handle such cases differently based on your specific needs.
Wrapping Up
Extracting the extension from a filename in Python is a common task, and with the help of the os.path
module, it becomes a breeze. Remember to consider different file path formats and handle any edge cases that may arise.
Now that you know how to extract the extension, go ahead and solve your file-related problems with ease! 💪
If you found this blog post helpful, don't forget to share it with your friends and colleagues. And if you have any questions or additional tips, feel free to leave a comment below. Happy coding! 😊🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
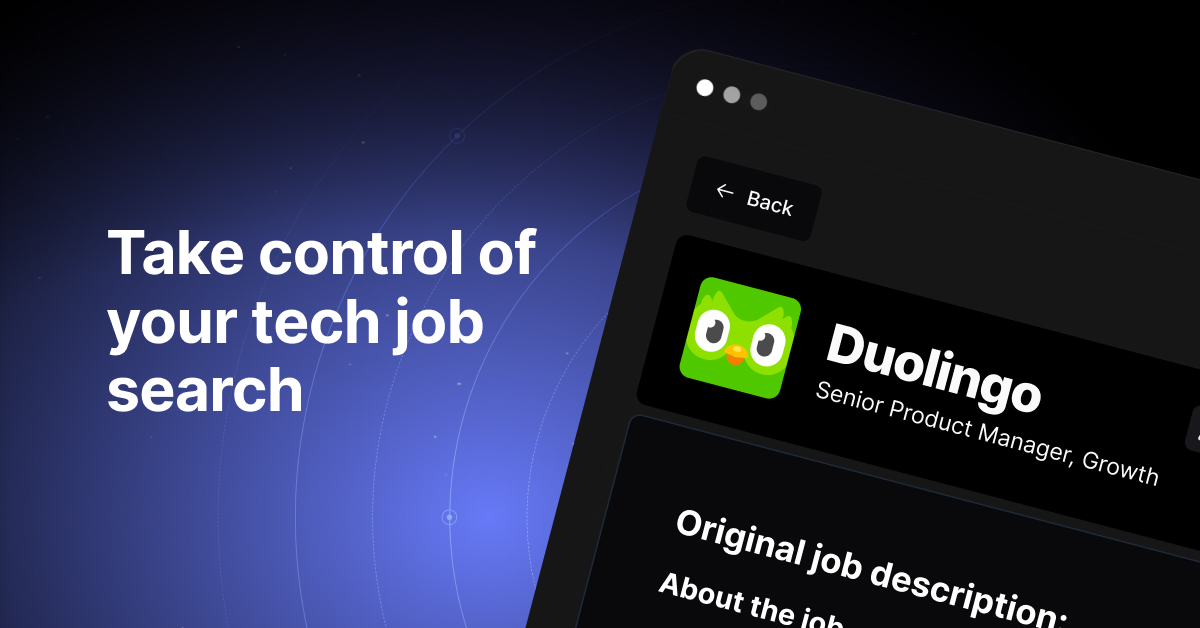