Extract file name from path, no matter what the os/path format
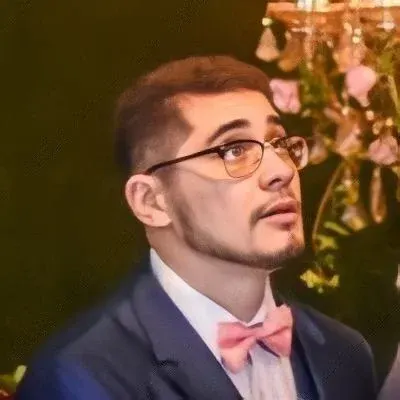
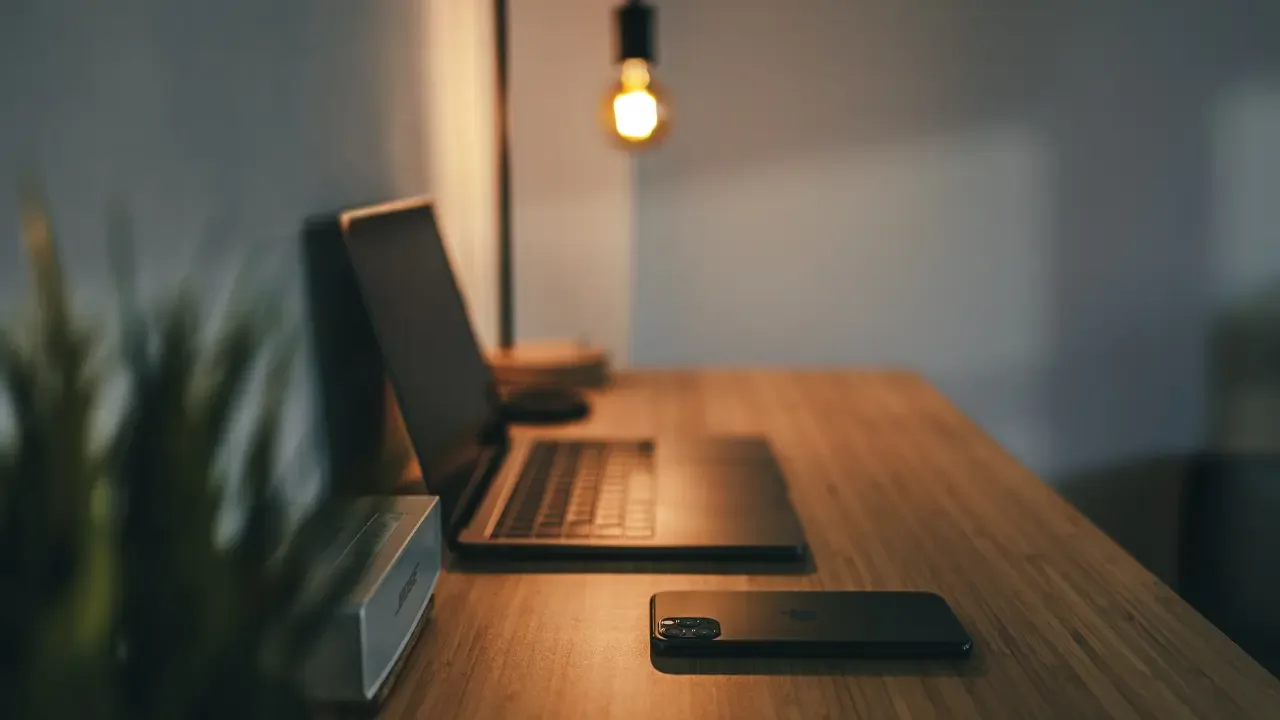
Extracting File Names from Paths: A Path of No Return ππ
Have you ever found yourself struggling to extract file names from paths, only to realize that the operating system or path format has thrown a wrench in your plans? Fear not! We're here to save the day with the perfect Python library that can handle this task, regardless of the OS or path format. ππ
The Quest for the Filename πΌπ
Imagine this scenario: you have a path, and all you really need is the file name. Seems simple enough, right? But when you encounter paths with mixed separators or unexpected characters, things can quickly become messy and frustrating.
To illustrate the challenge, let's take a look at some example paths:
a/b/c/
a/b/c
\a\b\c
\a\b\c\
a\b\c
a/b/../../a/b/c/
a/b/../../a/b/c
In each of these cases, our goal is to extract the file name "c"
from the given paths, regardless of the confusion caused by different operating systems or path formats.
Introducing the Pathlib Library π
To rescue us from this conundrum, we will enlist the help of the incredible pathlib
library. This powerful module was introduced in Python 3.4 as a way to work with file paths in a platform-independent manner.
The Almighty Solution: Python + Pathlib ππ‘
Let's dive right into the code and unlock the magic of pathlib
. Here's how you can extract the file name from a path using the library:
from pathlib import Path
def extract_file_name(path):
return Path(path).name
Yes, you heard that right! With just a few lines of code, we can extract the file name using the name
property of a Path
object. π©β¨
Testing Our Superpower β π§ͺ
To demonstrate the full capabilities of our solution, let's run through the example paths mentioned earlier and see if we can successfully extract the file name "c"
from each one:
paths = [
"a/b/c/",
"a/b/c",
"\\a\\b\\c",
"\\a\\b\\c\\",
"a\\b\\c",
"a/b/../../a/b/c/",
"a/b/../../a/b/c"
]
for path in paths:
print(extract_file_name(path))
When we execute this code, we should see the following output:
c
c
c
c
c
c
c
VoilΓ ! Our extract_file_name
function beautifully handles the paths with different separators, extra slashes, or even navigating directories with ".."
. The result is always the file name we desire. π
Conclusion: Embrace the Power of pathlib
! πͺπ
No longer will you struggle to extract file names from paths like a lost adventurer in the jungle of mixed separators and unexpected characters. By harnessing the power of the pathlib
library and our nifty extract_file_name
function, you can confidently handle this task in a platform-independent manner.
So, what are you waiting for? Unleash the code, extract those file names, and conquer your path-related challenges! Share your success stories, questions, or any other cool tips in the comments below. Let's navigate these paths together and embrace the beauty of clean, platform-independent code! ππ¨βπ»π¬
Remember, the path to success is just one function away!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
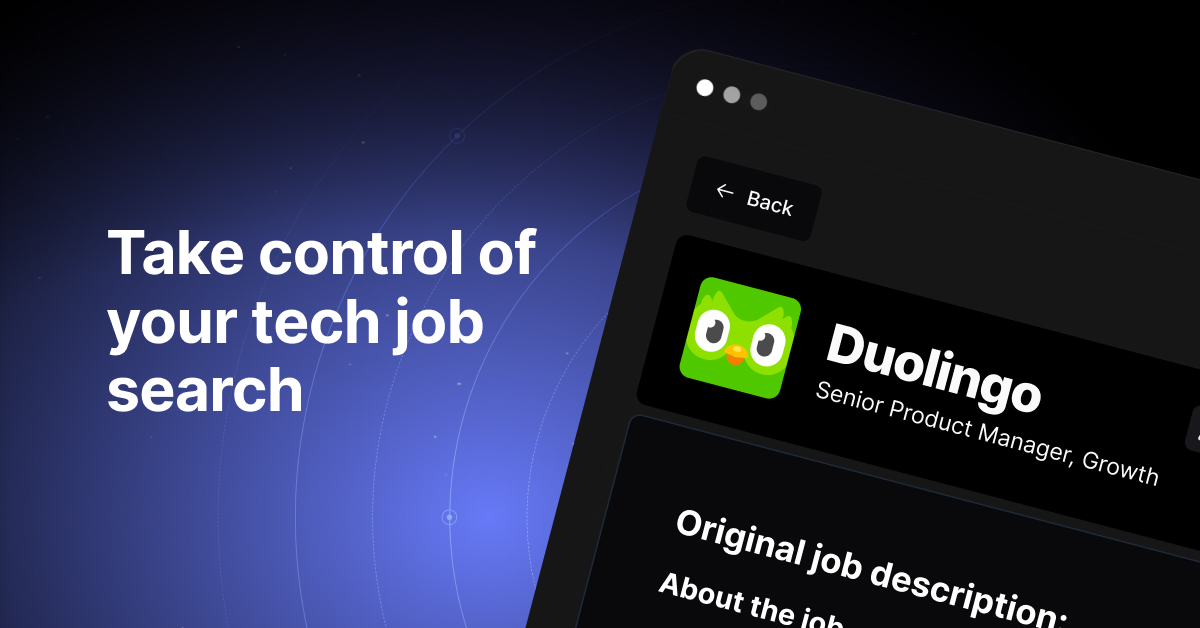