Extract a subset of key-value pairs from dictionary?
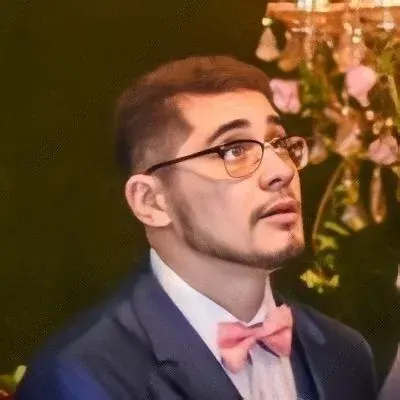
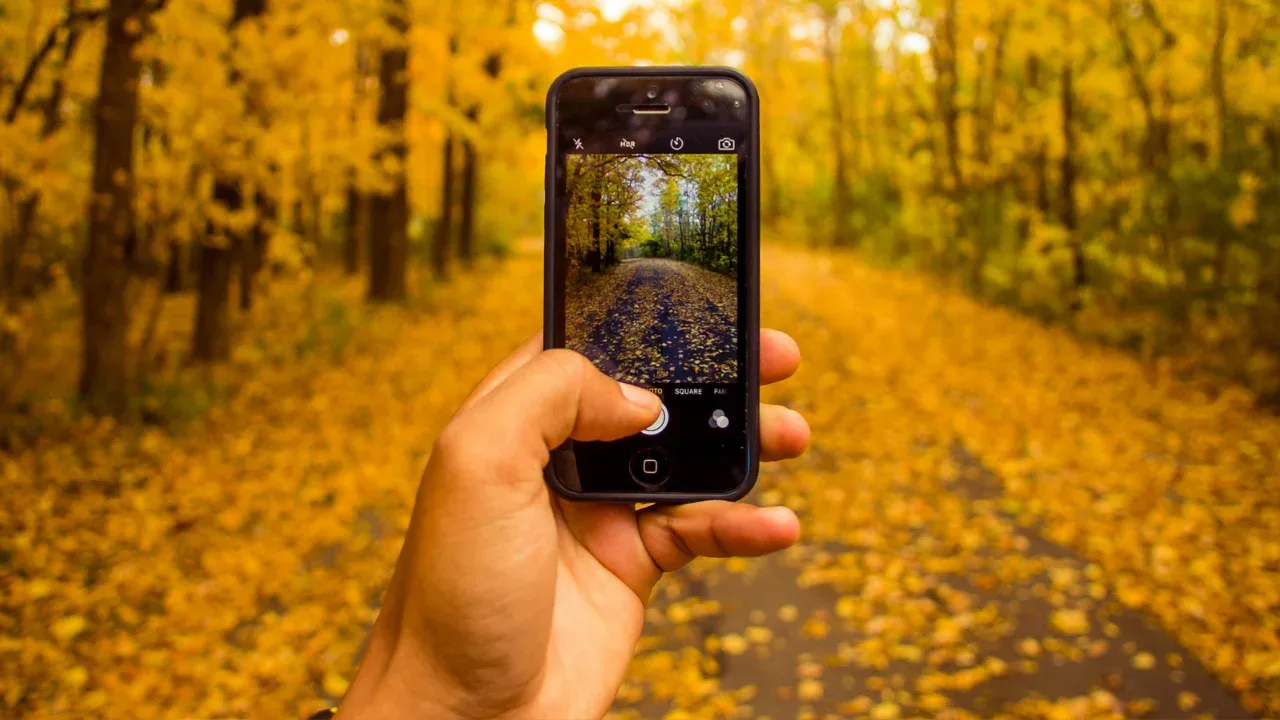
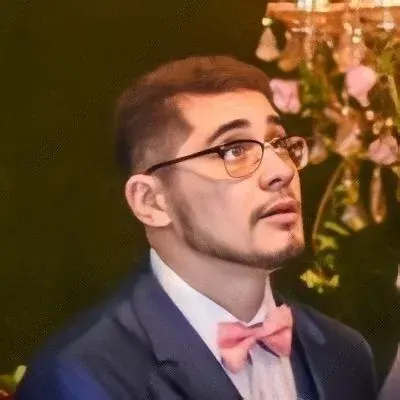
Extract a Subset of Key-Value Pairs from Dictionary: A Handy Guide for Python Developers!
š So you have this huge dictionary, probably with 16 key-value pairs, but you only need to extract a few specific ones? We get it! Sometimes, you just need a subset of the data without all the clutter. In this guide, we'll show you the easiest and most elegant ways to accomplish this task in Python. Let's dive right in! š»š
The Scenario: Big Dictionary, Few Interests
The scenario here is pretty straightforward: You have a dictionary object called bigdict
that contains a multitude of key-value pairs. However, you're only interested in a specific subset of three key-value pairs (let's call it subdict
).
In the example provided, the bigdict
looks something like this:
bigdict = {'a': 1, 'b': 2, ..., 'z': 26}
š¤ The challenge is to efficiently extract a subset of this bigdict
containing only the key-value pairs you're interested in.
The Initial Approach: Manually Creating a Subset
The initial approach suggested in the provided context is to manually create the subdict
by individually selecting the required key-value pairs from the bigdict
. Here's how it looks like in code:
subdict = {'l': bigdict['l'], 'm': bigdict['m'], 'n': bigdict['n']}
š While this solution works perfectly fine, the developer acknowledges that there might be a more elegant and efficient way to achieve the same result.
The Elegant Solution: Dictionary Comprehension
š” Good news! There indeed is a more elegant and concise way to extract a subset of key-value pairs from a dictionary, and it's called Dictionary Comprehension. This technique allows us to create a new dictionary based on the existing one, selecting only the desired key-value pairs using a simple one-liner.
Here's how you can use dictionary comprehension to extract the subdict
in a more concise way:
subdict = {key: bigdict[key] for key in ['l', 'm', 'n']}
š Ta-da! In just a single line of code, we have created a new dictionary subdict
containing only the key-value pairs with keys 'l'
, 'm'
, and 'n'
. No more manual hardcoding required! š
Additional Tips and Tricks
š¹ Flexibility: Dictionary comprehension allows you to dynamically select the keys you want to extract. You can easily replace ['l', 'm', 'n']
with any list or iterable containing the desired keys.
š¹ Avoiding Key Errors: If the keys you're interested in may not exist in the bigdict
, you can use the get()
method to provide a default value for missing keys. For example:
subdict = {key: bigdict.get(key, default_value) for key in ['l', 'm', 'n']}
š¹ Subset based on conditions: You can also use conditional statements within dictionary comprehension to filter key-value pairs based on certain conditions. This allows you to create a subset that meets specific criteria!
Time to Level Up Your Code!
š You've now learned a more elegant and efficient way to extract a subset of key-value pairs from a dictionary using dictionary comprehension. Say goodbye to manual extraction and welcome a sleeker coding approach.
š” So go ahead and try out this technique in your programs! If you haven't used dictionary comprehension before, it's a fantastic addition to your Python toolkit.
We hope you found this guide helpful! If you have any questions or would like to share your own tips, feel free to leave a comment below. Happy coding! š©āš»šØāš»š¬