Execute code when Django starts ONCE only?
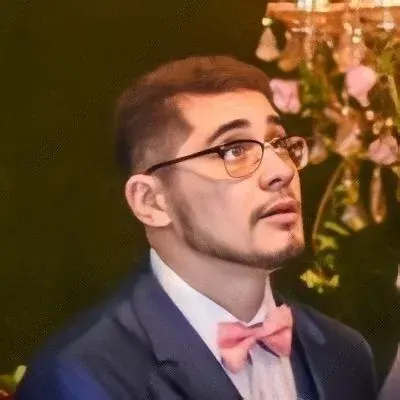
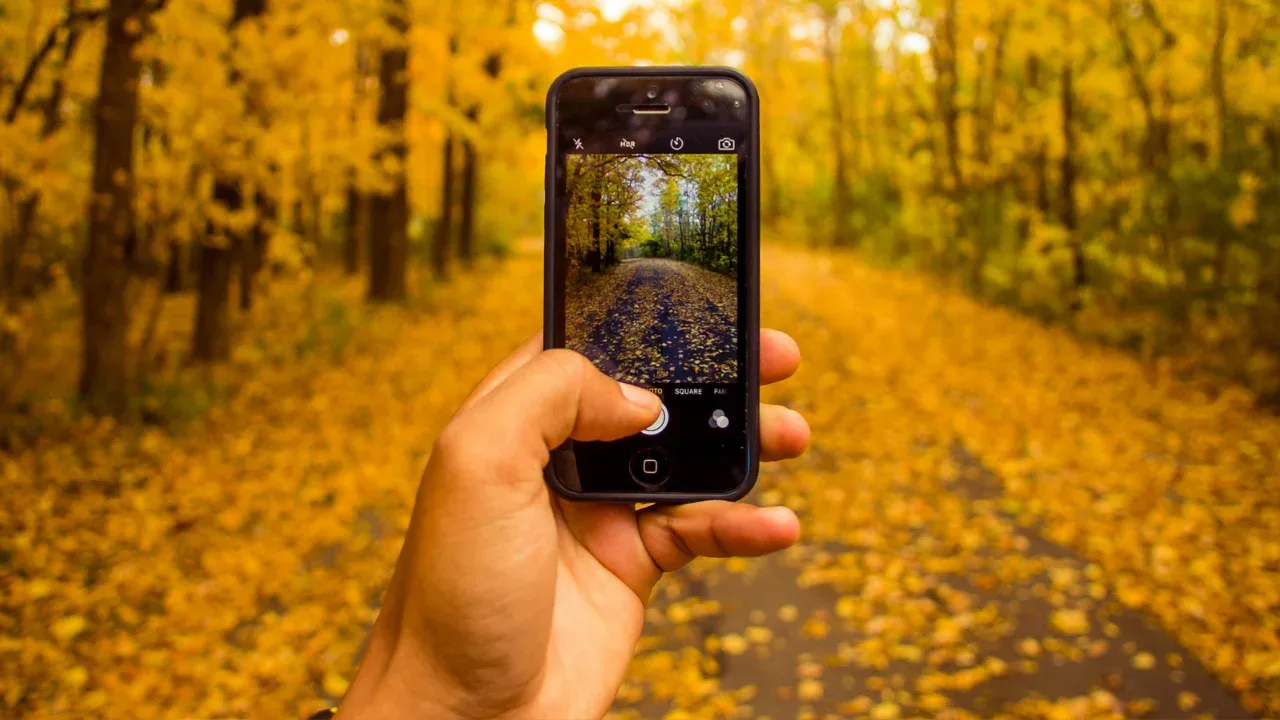
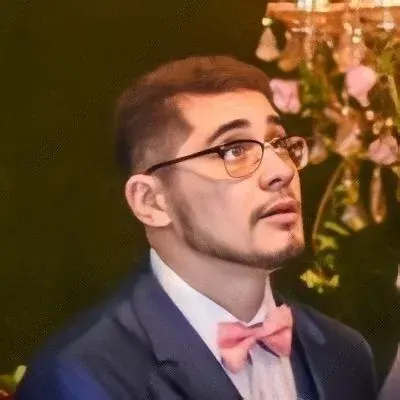
Executing Code When Django Starts Once Only 🚀
Have you ever found yourself in a situation where you need to execute code once when Django starts up? Maybe you want to initialize some arbitrary code or perform some setup tasks. If you have, then you've come to the right place!
In this blog post, we'll address the common issue of code being executed multiple times on Django startup and provide you with easy solutions to ensure your code runs only once. Let's dive in! 💪
Understanding the Problem 🕵️♀️
The issue you're facing is related to the Django Middleware class, which allows you to modify the request and response objects. You've followed a solution posted by sdolan, but unfortunately, the "Hello" message is output to the terminal twice when you run Django using runserver
. Let's figure out why this happens.
In the code snippet you shared, the StartupMiddleware
class has an __init__
method, which gets called every time a new instance of the middleware is created. The "Hello world" message is printed in the __init__
method. Additionally, you're raising a MiddlewareNotUsed
exception to indicate that the middleware should not be used.
However, Django treats this exception differently when running in development mode with runserver
. Instead of excluding the middleware entirely, it catches the exception and continues with the request. This is why you see the "Hello world" message printed twice in the terminal.
Easy Solutions to Run Code Once ✅
Now that we understand the problem, let's explore a couple of easy solutions to make sure your code runs only once when Django starts.
Solution 1: Use a flag 🚩
One simple approach is to use a flag variable to track whether the code has already been executed. Here's an updated version of the StartupMiddleware
class that incorporates this solution:
from django.core.exceptions import MiddlewareNotUsed
from django.conf import settings
class StartupMiddleware(object):
def __init__(self):
if hasattr(settings, 'startup_complete'):
return
settings.startup_complete = True
print("Hello world")
raise MiddlewareNotUsed('Startup complete')
In this solution, we're checking if the startup_complete
attribute exists in the settings module. If it does, we return early without executing the code. Otherwise, we set the startup_complete
attribute to True
, execute the code, and raise the MiddlewareNotUsed
exception as before.
Solution 2: Use a decorator 🎉
Another elegant way to achieve the desired behavior is by using a decorator. Decorators allow you to modify the behavior of a function or method, making them perfect for our use case. Here's how you can modify the StartupMiddleware
class using a decorator:
from django.core.exceptions import MiddlewareNotUsed
from django.conf import settings
def run_once(func):
def wrapper(*args, **kwargs):
if not wrapper.has_run:
wrapper.has_run = True
return func(*args, **kwargs)
wrapper.has_run = False
return wrapper
class StartupMiddleware(object):
@run_once
def __init__(self):
print("Hello world")
raise MiddlewareNotUsed('Startup complete')
In this solution, we define a run_once
decorator that keeps track of whether the decorated method has already run. If it hasn't, the method is executed, and the flag is set to indicate that it has run. If it has already run, the method is simply skipped. This decorator can be easily reused in other parts of your codebase as well.
Your Turn to Shine! 💡
Congratulations! You've learned how to execute code once when Django starts up without it being executed multiple times. Now it's time to implement these solutions and see them in action in your own Django projects. Don't forget to share your experiences and any additional solutions you come up with in the comments below! 👇
Let's make the Django startup experience smoother and more efficient together! Happy coding! 🎉✨