Escaping regex string
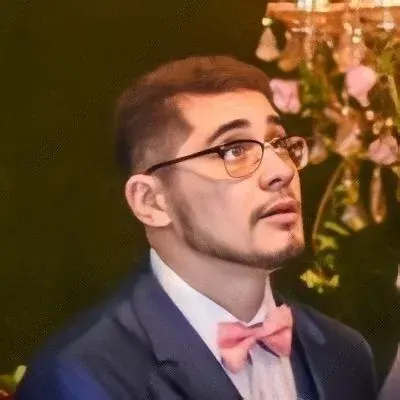
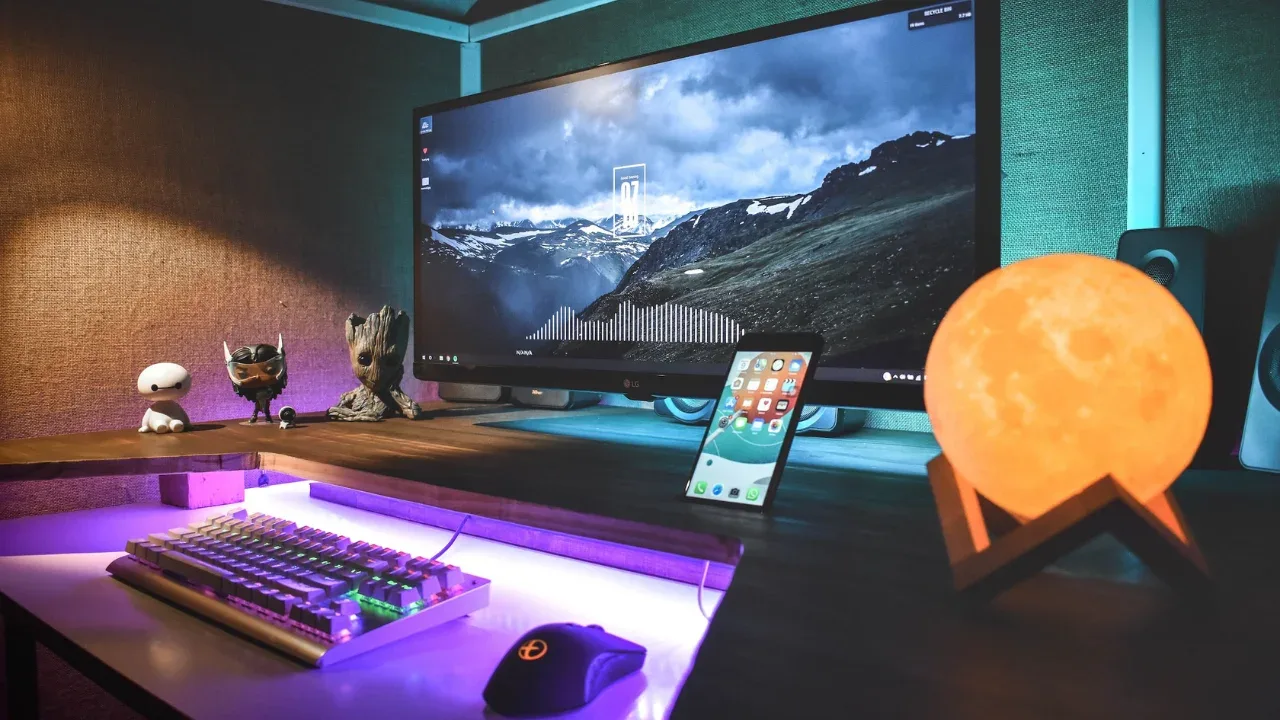
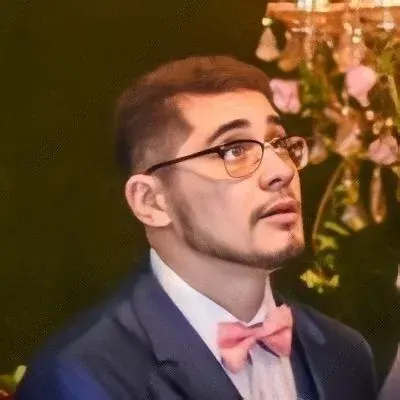
Escaping Regex String: A Handy Guide to Tame the Wild Symbols 🦁
Are you tired of tussling with regex patterns that seem to have a mind of their own? Do you find it challenging to handle user input when it contains characters that have special meaning in regex? Fear not, for we have some tricks up our sleeves to help you escape those wild symbols and regain control over your regex searches! 🎩✨
Understanding the Challenge 🕵️♀️
So, you want to allow users to input a regex pattern for searching through text. However, when users include characters like parentheses, brackets, or other regex metacharacters, the regex engine interprets them as special symbols. This can lead to unexpected behavior and incorrect search results. 😱
For instance, let's say a user wants to search for the word "Word (s)". The regex engine will treat the "(s)" as a capture group instead of a literal string. This can mess up your search logic and cause frustration for your users. 😫
The Replace Solution 💡
One way to tackle this problem is by using the replace
function to escape the regex symbols in the user's input. This involves replacing each regex symbol with its escaped counterpart. However, this approach can be time-consuming and error-prone, especially if you need to handle all possible regex metacharacters. 😓
To escape certain characters, you need to prepend a backslash () before them. For example, ( and ) represent the literal parentheses you want to match. Similarly, to escape characters like *, +, ?, and ., you prepend them with a backslash as well.
Here's an example in JavaScript:
function escapeRegex(pattern) {
const specialChars = ['\\', '^', '$', '.', '|', '?', '*', '+', '(', ')', '[', ']', '{', '}'];
return pattern.replace(new RegExp(`[${specialChars.join('')}]`, 'g'), '\\$&');
}
const userInput = '(s)';
const escapedPattern = escapeRegex(userInput);
console.log(escapedPattern); // Prints '\(s\)'
With this function, you can quickly escape common regex symbols in the user's input. However, it's important to note that this is a basic solution and might not handle all edge cases. 🧐
The Pre-Built Function Solution 🚀
Luckily, many programming languages and libraries offer built-in functions to escape regex strings automatically. These functions take care of escaping all the necessary symbols, so you don't have to worry about missing any.
Let's look at a couple of examples:
In JavaScript, you can use the
RegExp.escape()
function (added in ECMAScript 2019) to automatically escape regex metacharacters:const userInput = '(s)'; const escapedPattern = RegExp.escape(userInput); console.log(escapedPattern); // Prints '\(s\)'
In Python, the
re.escape()
function allows you to escape all the special characters in a regex string:import re userInput = '(s)' escapedPattern = re.escape(userInput) print(escapedPattern) # Prints '\\(s\\)'
These pre-built functions provide a more robust and reliable solution, saving you time and effort. 😎
Your Turn to Escape the Wild Symbols 🦁
Now that you know how to escape regex symbols like a pro, go ahead and apply this knowledge to your own projects. Experiment with different programming languages and libraries to find the most convenient solution for your specific needs. Remember, escaping regex symbols is not a problem – it's an opportunity for growth! 💪🌟
If you have any questions or want to share your own tips and tricks, leave a comment below. Let's escape the regex jungle together! 🌴🐒
Keep learning, keep coding! 💻❤️⌨️