Dropping infinite values from dataframes in pandas?
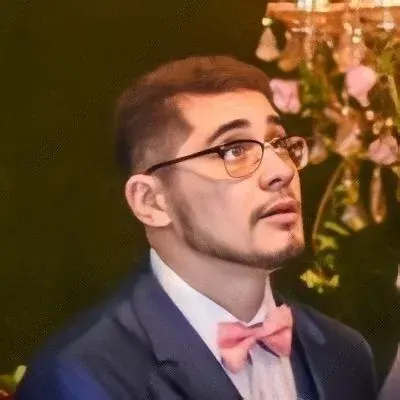
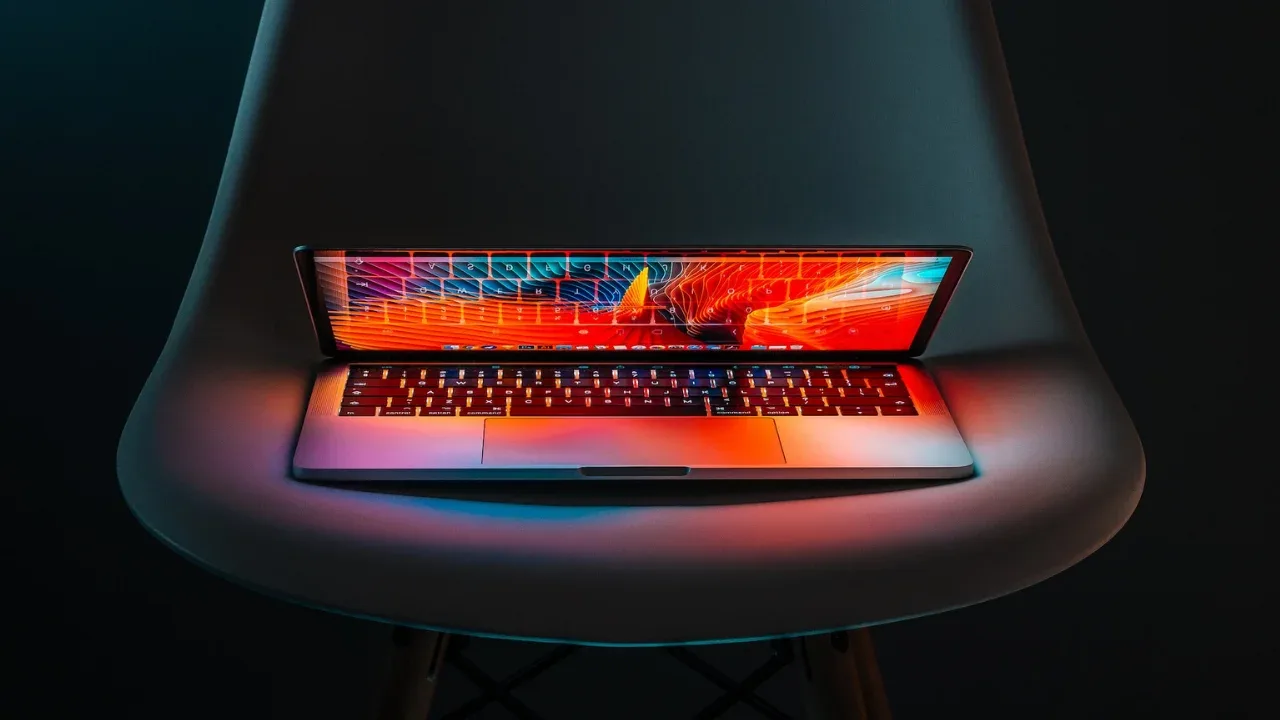
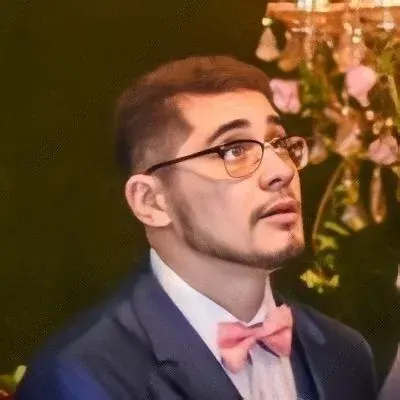
Dropping Infinite Values from Dataframes in Pandas: A Complete Guide 🐼
Are you struggling with how to drop those pesky infinite values from your pandas dataframe? It can be a frustrating challenge, but fear not! In this blog post, we will explore common issues and provide easy solutions to help you clean up your data like a pro.
The Problem 🤔
Let's start by understanding the problem. Your dataframe may contain various types of missing values, including nan
, inf
, and -inf
. These infinite values can be generated during mathematical operations, and they can wreak havoc on your data analysis if not handled properly.
The typical approach to dropping missing values is to use the dropna
method in pandas. However, by default, dropna
does not consider inf
and -inf
as missing values. This presents a challenge when you want to drop rows or columns containing these infinite values.
The Solution 💡
Fortunately, there are a couple of straightforward solutions that can help you drop infinite values from your dataframe.
Solution 1: Changing the mode.use_inf_as_null
Option
Pandas provides a configuration option called mode.use_inf_as_null
, which controls whether to treat inf
and -inf
as missing values. By default, this option is set to False
. To include infinite values in the definition of missing values and enable dropna
to work as expected, you can change this option to True
. Here's an example:
import pandas as pd
# Change the mode.use_inf_as_null option to True
pd.set_option('mode.use_inf_as_null', True)
# Now dropna will consider inf and -inf as missing values
df.dropna(subset=["col1", "col2"], how="all")
Solution 2: Customizing the dropna
Method
Alternatively, you can handle infinite values explicitly while using the dropna
method. The subset
parameter allows you to specify the columns in which you want to drop the missing values. By default, dropna
removes any rows containing nan
, but you can customize it to include inf
and -inf
. Here's an example:
# Drop rows with nan, inf, and -inf values from specific columns
df.dropna(subset=["col1", "col2"], how="all")
Conclusion and Call-to-Action 🏁
Dropping infinite values from your pandas dataframe is no longer a challenge. You've learned two effective solutions to handle inf
and -inf
as missing values using the dropna
method. Now, it's time to put this knowledge into practice!
Try applying these solutions to your own dataframe and see how it cleans up your data. Don't forget to share your success stories and any additional tips in the comments section below. Happy coding!