Drop all duplicate rows across multiple columns in Python Pandas
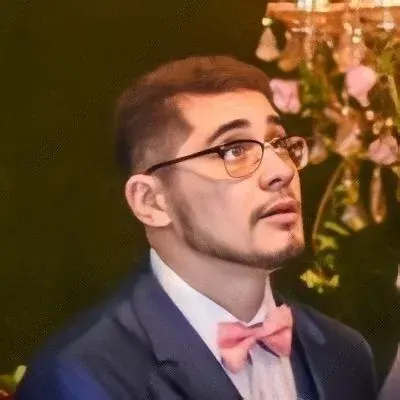
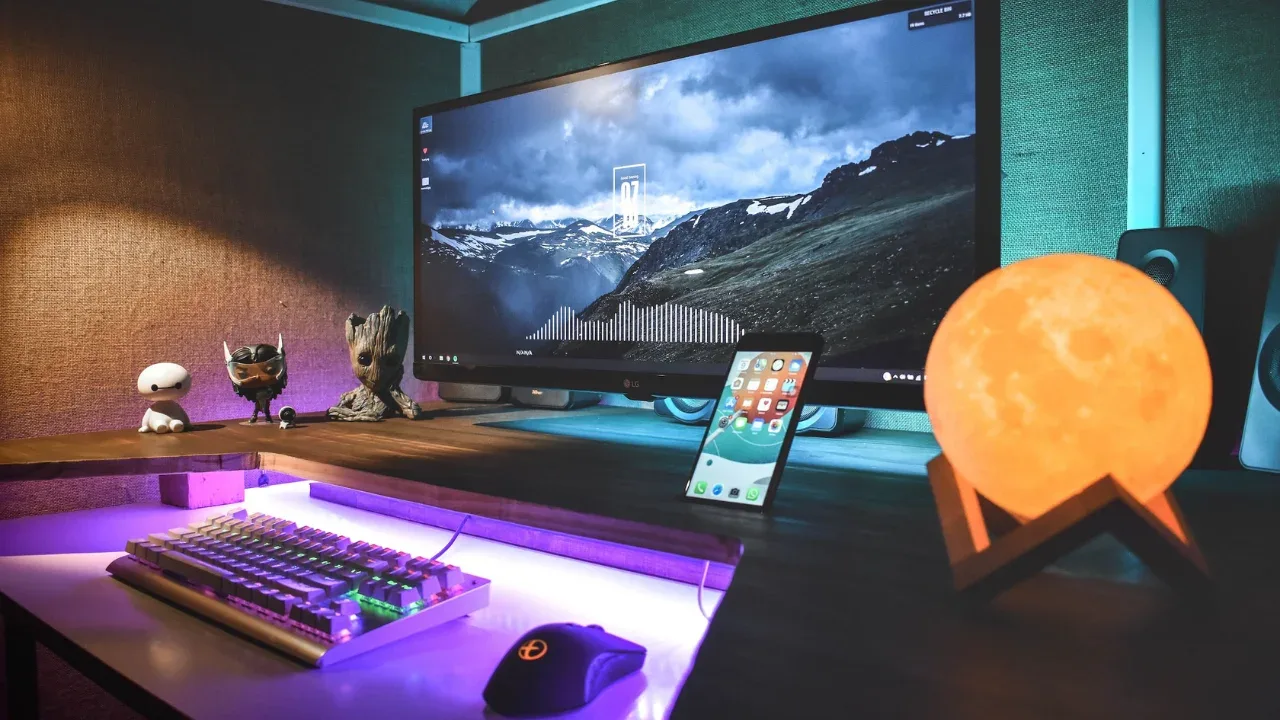
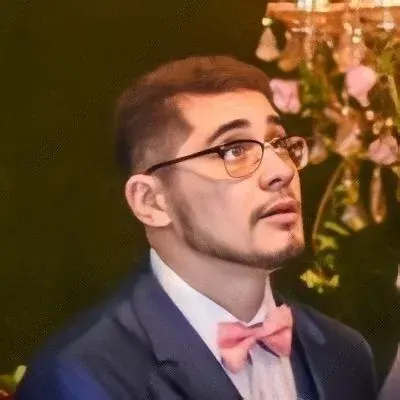
How to Drop All Duplicate Rows Across Multiple Columns in Python Pandas 😎
Have you ever encountered a situation where you needed to remove duplicate rows that occur across multiple columns in a Python Pandas DataFrame? 🧐 Don't worry, you're not alone! In this post, I will show you how to address this common data manipulation problem with easy solutions.
Let's dive right in! 💪
The Problem 🤔
Suppose you have a DataFrame with multiple columns and you want to drop all the rows that contain duplicate values across a subset of those columns. 📊 For example, consider the following DataFrame:
A B C
0 foo 0 A
1 foo 1 A
2 foo 1 B
3 bar 1 A
In this case, you want to drop rows 0 and 1 because they have duplicates in columns A and C. How can you achieve this in Python Pandas? Let's find out! 💡
The Solution 🔧
Python Pandas provides a convenient function called drop_duplicates()
that allows us to remove duplicate rows from a DataFrame. However, by default, it considers all columns when checking for duplicates. In order to drop rows with duplicates only across specific columns, we can pass a subset of columns to the subset
parameter of the drop_duplicates()
function. 🙌
Here's how you can use drop_duplicates()
to drop all duplicate rows across multiple columns:
df.drop_duplicates(subset=['A', 'C'], inplace=True)
In the above code snippet, we specify the columns 'A' and 'C' as the subset for checking duplicates. By setting the inplace
parameter to True
, we modify the original DataFrame in place. If you want to create a new DataFrame without the duplicate rows, you can omit the inplace
parameter or set it to False
.
And just like that, the duplicate rows across the specified columns are dropped, and you're left with a clean DataFrame. 🎉
The Call-to-Action 📢
Now that you know how to drop all duplicate rows across multiple columns in Python Pandas, go ahead and try it out on your own datasets. It's a great way to ensure data integrity and streamline your data analysis workflows! 💯
If you found this guide helpful, don't forget to give it a thumbs-up 👍 and share it with your fellow Pythonistas! If you have any questions or need further assistance, feel free to leave a comment below. I'd be more than happy to help you out. 😊
Happy coding! 🚀