Download file from web in Python 3
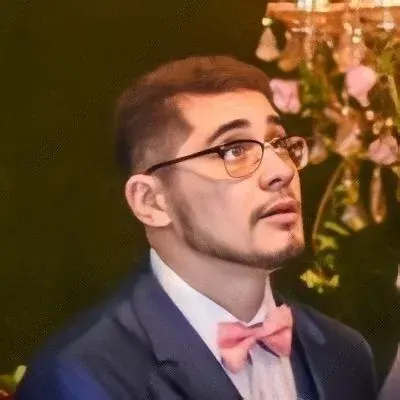
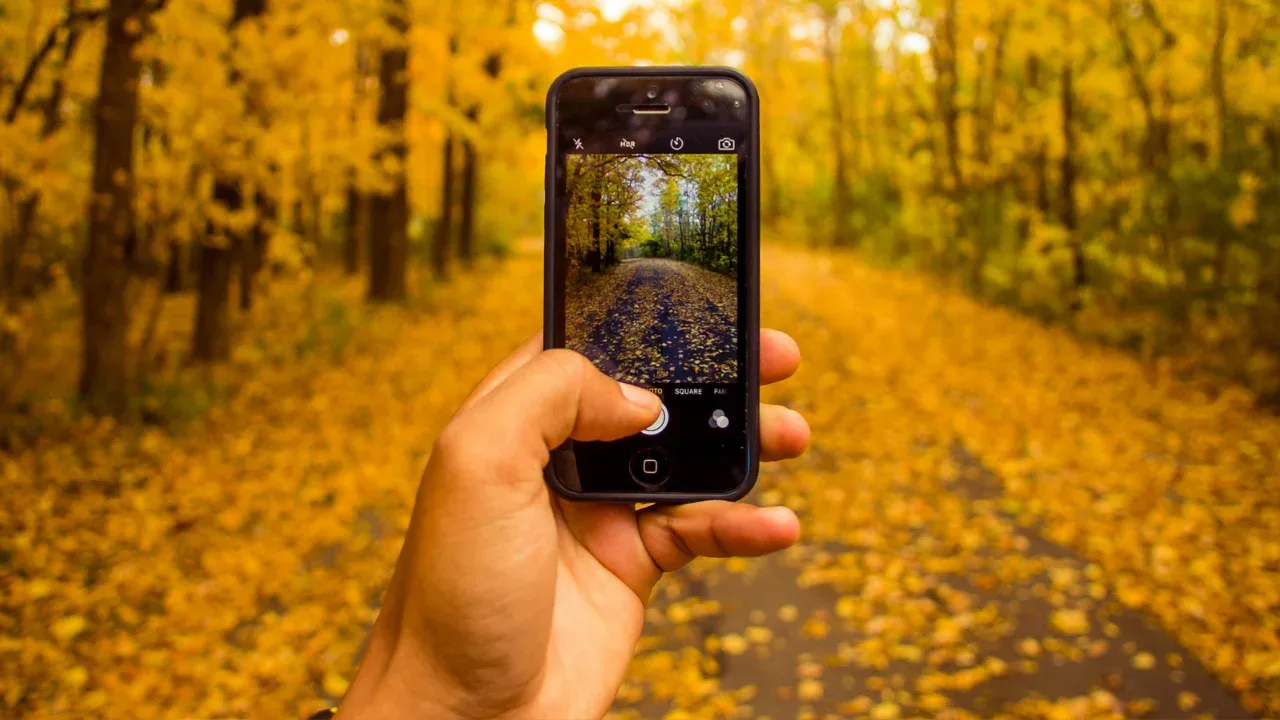
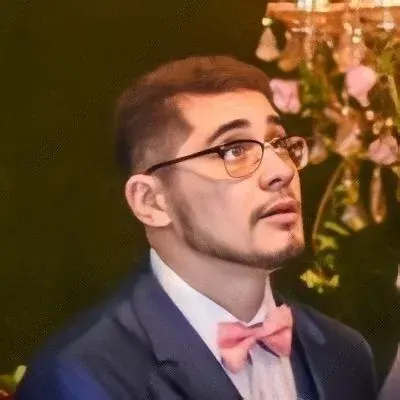
📥 How to Download a File from the Web in Python 3 🐍
Have you ever tried to write a program in Python that downloads a file from a web server? It can be super useful, but it can also be quite challenging, especially when you encounter common issues like dealing with different types of data or encoding problems.
In this guide, we'll explore a common problem where the URL to the file you want to download is stored as a string, rather than the expected bytes type. We'll walk you through the steps to resolve this issue and provide you with an easy solution. So let's dive in!
🚧 The String Type Error
The first thing you may encounter is an error stating that the type in your download file function should be bytes, not a string. This error occurs when you pass a string URL to the httplib2.Http.request()
method.
Luckily, there's a way to convert the string URL into the appropriate bytes type and solve this issue.
💡 Solution
To convert the string URL into bytes, you can use the encode()
method from the str
class. Replace the line resp, content = h.request(URL, "GET")
with the following code snippet:
<pre><code>resp, content = h.request(URL.encode('utf-8'), "GET") </code></pre>
By encoding the URL as UTF-8, you transform the string into bytes, ensuring it is compatible with the Http.request()
method.
Here's the updated downloadFile
function:
<pre><code>def downloadFile(URL=None): import httplib2 h = httplib2.Http(".cache") resp, content = h.request(URL.encode('utf-8'), "GET") return content downloadFile(URL_from_file)</code></pre>
With this solution, you should be able to download the file from the web server without encountering any type errors.
🌟 Call-to-Action: Share Your Experience
We hope this guide helped you understand how to download a file from the web in Python 3, even when dealing with string URLs. Now, it's your turn! Have you encountered any other challenges or errors while working with web downloads in Python? Share your experience in the comments below. Let's help each other learn and grow as developers!
Happy coding! 💻✨