Does Python have a string "contains" substring method?
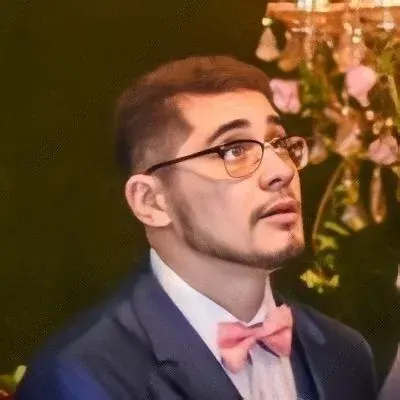
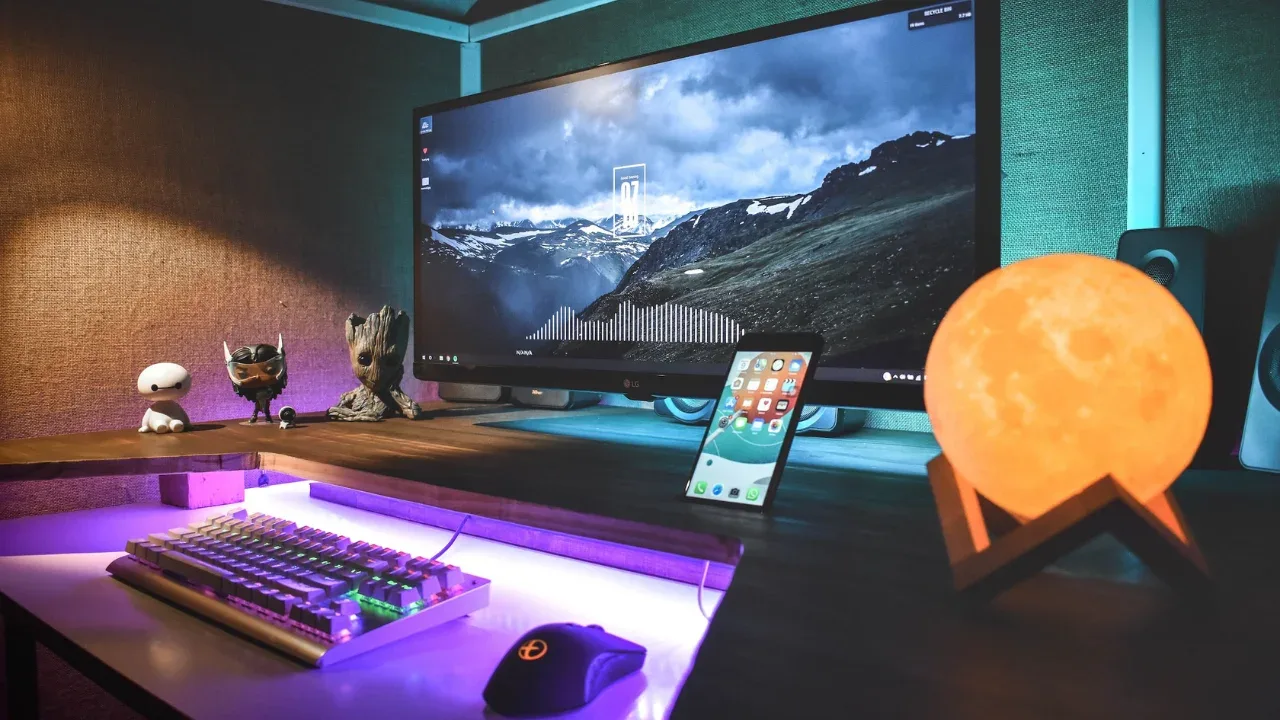
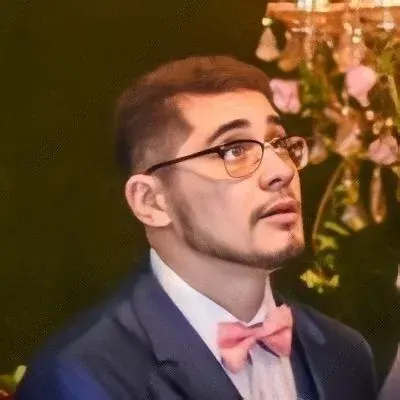
Does Python Have a String 'Contains' Substring Method? 🐍🔍
So you're looking for a way to check if a string contains a specific substring in Python, huh? Well, you've come to the right place! 🎉
The Common Confusion 😕
When transitioning from other programming languages to Python, it's natural to look for a method similar to string.contains
or string.indexof
that you might have used before. However, Python does not provide these exact methods. 😮
The Good News 🎉
Fear not, my friend! Python does offer an elegant solution to check if a string contains a substring. 🙌 The most common way to do this is by using the in
keyword. Let me explain how it works with a couple of examples:
if "blah" not in somestring:
# Do something if 'blah' is not present in somestring
continue
In this code snippet, the not in
keyword checks if the substring "blah" is not present in the somestring
. If it's true, the code inside the if
block is executed. Otherwise, it continues to the next line.
But what if you want to check if the substring is present instead? 🤔 Easy-peasy! Just remove the not
keyword:
if "blah" in somestring:
# Do something if 'blah' is present in somestring
continue
This time, the code block will be executed only if the substring "blah" is found within the somestring
.
The All-Inclusive Method 🔍
If you prefer a method-style approach, Python includes the str.find()
method that can be used to achieve the same result. Here's how you can use it:
if somestring.find("blah") == -1:
# Do something if 'blah' is not present in somestring
continue
In this code snippet, we check if find()
returns -1, which indicates that the substring "blah" is not found within somestring
. If that's the case, the code block inside the if
statement will be executed.
Similarly, if you want to check if the substring is present, you can modify the condition as follows:
if somestring.find("blah") != -1:
# Do something if 'blah' is present in somestring
continue
The Bottom Line ✅
Although Python lacks a string.contains
or string.indexof
method, it offers multiple ways to check if a string contains a substring. Whether you prefer using the in
keyword or the str.find()
method, you have options that can suit your coding style and preference. 😎
So, next time you're working with strings in Python, remember to use the in
keyword or the str.find()
method to check for substring presence or absence. And if you ever get confused, just refer back to this guide. 😉✨
Now, go forth and write code that checks for awesome substrings! And remember to share this post with your fellow Pythonistas! 🚀🔗
Did this guide help you understand how to check for substrings in Python? Share your thoughts and questions in the comments below! Let's learn together! 🤓💬