Django - what is the difference between render(), render_to_response() and direct_to_template()?
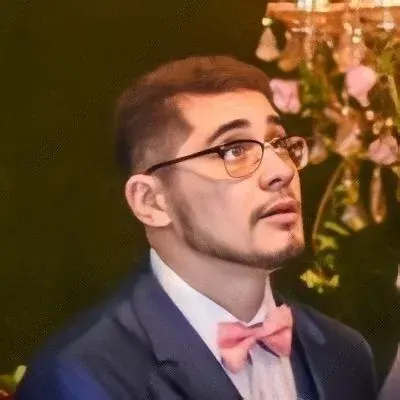
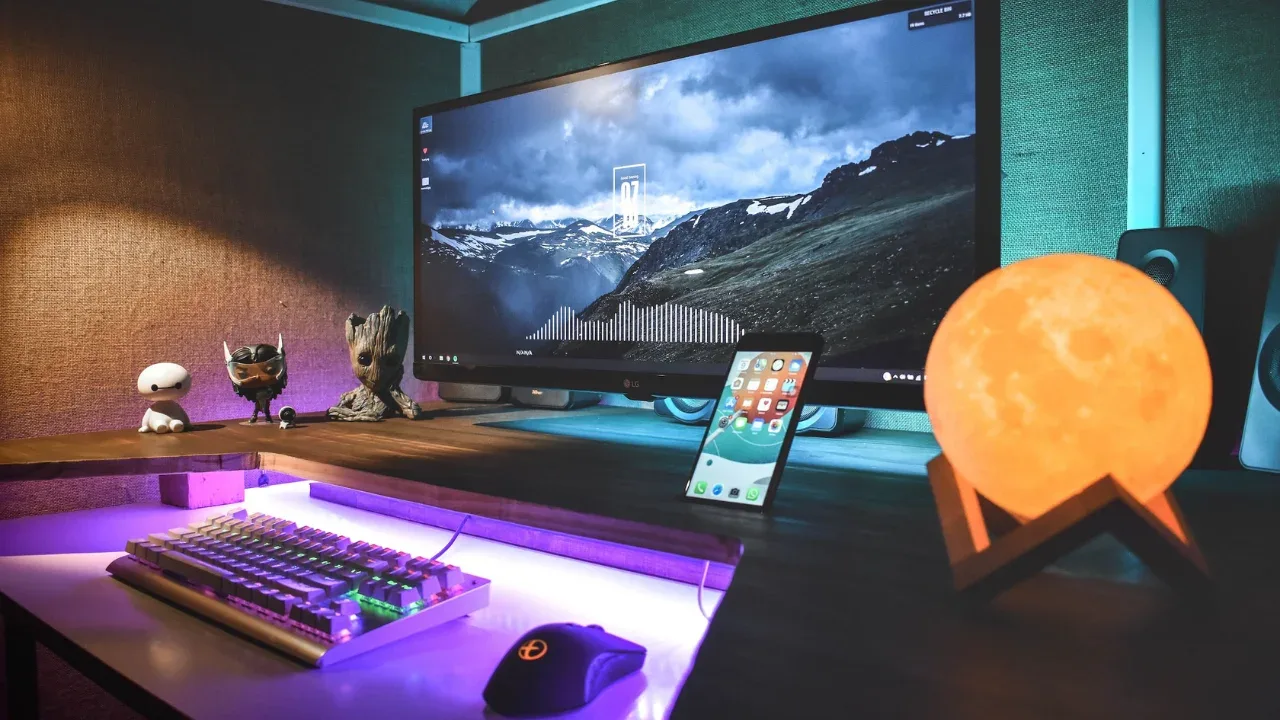
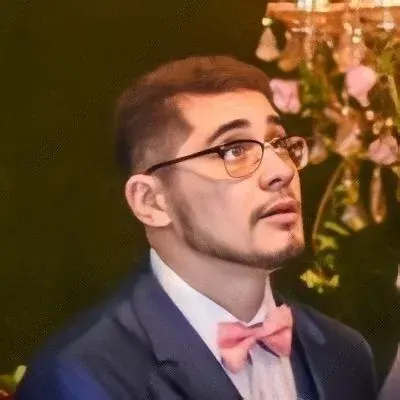
Django - What's the Difference Between render(), render_to_response(), and direct_to_template()?
So you're just starting out with Python and Django, and you come across these three methods: render()
, render_to_response()
, and direct_to_template()
. You're probably wondering, "What's the difference between them, and when should I use each one?" Don't worry, I've got you covered! In this blog post, I'll break it down for you in simple terms and provide easy-to-understand examples.
render()
Let's start with render()
. This method is commonly used in Django views to render a template with a given context. It takes the following parameters:
render(request, template_name, context=None, content_type=None, status=None, using=None)
request
is the HttpRequest object.template_name
is the name of the template to render.context
(optional) is a dictionary of values to populate the template with.content_type
(optional) is the content type of the response.status
(optional) is the HTTP status code.using
(optional) is the name of the template engine to use.
Here's an example of how you can use render()
in a view:
def comment_edit(request, object_id, template_name='comments/edit.html'):
comment = get_object_or_404(Comment, pk=object_id, user=request.user)
# ...
return render(request, template_name, {
'form': form,
'comment': comment,
})
In this example, render()
is used to render the comments/edit.html
template and pass the form
and comment
variables as context.
render_to_response()
Next up is render_to_response()
. This method is similar to render()
, but there is one main difference: it does not use the request object for context processing. The parameters for render_to_response()
are as follows:
render_to_response(template_name, context=None, content_type=None, status=None, using=None)
template_name
is the name of the template to render.context
(optional) is a dictionary of values to populate the template with.content_type
(optional) is the content type of the response.status
(optional) is the HTTP status code.using
(optional) is the name of the template engine to use.
Here's an example to demonstrate the usage of render_to_response()
:
return render_to_response(template_name, my_data_dictionary, context_instance=RequestContext(request))
In this example, render_to_response()
is used to render a template and pass a my_data_dictionary
as context. Notice that we also use context_instance=RequestContext(request)
to ensure that context processors are applied.
direct_to_template()
Last but not least, we have direct_to_template()
. This method is a shortcut for rendering a template with a given context. It is a simpler alternative to render()
and render_to_response()
. The parameters for direct_to_template()
are:
direct_to_template(request, template=template_name, extra_context=None, mimetype=None, **kwargs)
request
is the HttpRequest object.template
is the name of the template to render.extra_context
(optional) is a dictionary of extra values to populate the template with.mimetype
(optional) is the content type of the response.**kwargs
can be used to pass additional context variables.
Here's an example of using direct_to_template()
:
return direct_to_template(request, template_name, my_data_dictionary)
In this example, direct_to_template()
is used to render a template and pass my_data_dictionary
as context.
Which one should I use?
Now that we've covered the differences and examples of each method, you might be wondering when to use them. Here's a simple guide:
Use
render()
when you want to render a template with the request object and pass a context.Use
render_to_response()
when you want to render a template without the request object (e.g., in a script or a custom method).Use
direct_to_template()
when you want a shortcut to render a template with a given context.
Remember, these are just guidelines, and you should choose the method that best suits your needs and the structure of your project.
Conclusion
Congratulations! You've made it to the end of this guide, and now you have a clear understanding of the differences between render()
, render_to_response()
, and direct_to_template()
in Django. You've also learned how to use them with easy-to-understand examples.
If you still have any questions or need further clarification, feel free to leave a comment below. Happy coding! 😊🚀
Did this guide help you understand the differences between render()
, render_to_response()
, and direct_to_template()
in Django? Let us know in the comments and share your insights!