Django template how to look up a dictionary value with a variable
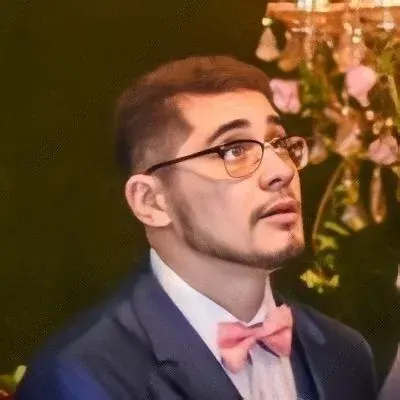
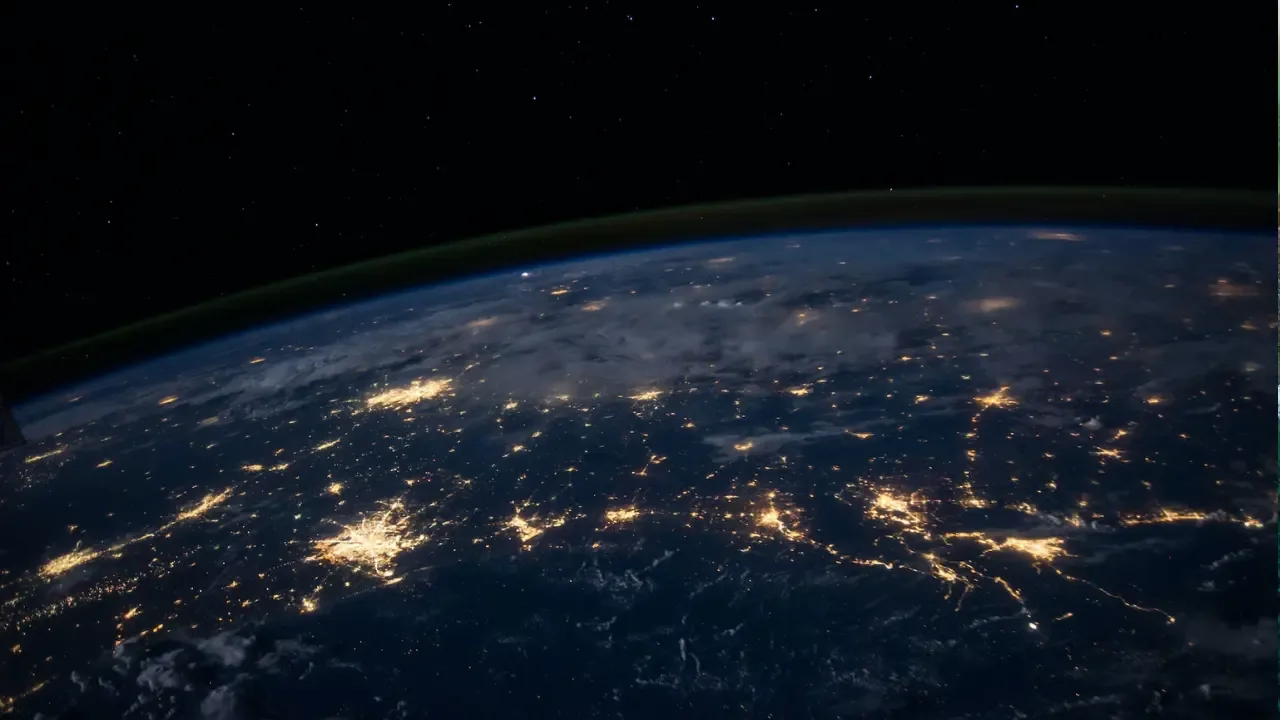
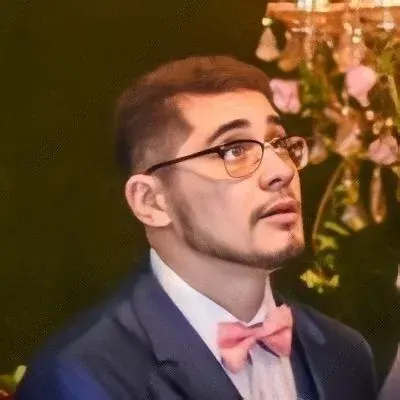
Django Template: How to Look Up a Dictionary Value with a Variable
š Do you find yourself constantly struggling with looking up dictionary values using variables in Django templates? Don't worry, you're not alone! In this blog post, we'll tackle this common issue head-on and provide you with easy and practical solutions. So, let's dive in! šŖš¼
The Regular Way
When you have a static key, looking up dictionary values in a Django template is a piece of š°. For example, if you have a dictionary called mydict
with keys "key1" and "key2" and their respective values "value1" and "value2", you can simply use {{ mydict.key1 }}
and {{ mydict.key2 }}
to access them.
The Problem
But what if you want to use a variable as the key? Uh oh! That's where the trouble starts! š Let's say you have a loop in your template, where each iteration has an item
with an attribute called "NAME". You want to use the value of item.NAME
as the key to look up a value in mydict
. Your code might look like this:
{% for item in list %}
{{ mydict.item.NAME }} # I want to look up mydict[item.NAME]
{% endfor %}
The Failed Attempt
Unfortunately, simply using mydict.item.NAME
won't work. Django doesn't allow this direct approach as it looks for "item" as a key in the dictionary, rather than the value of item.NAME
. As a result, you'll end up with a frustrating KeyError
. š¢
The Solution
Thankfully, there's a clever and easy way to overcome this hurdle! The trick is to use the built-in templatefilter
called dictlookup
, which allows us to perform dynamic dictionary lookups using variables.
Let's modify our previous code to make it work:
{% for item in list %}
{{ mydict|dictlookup:item.NAME }} # Yay! It works!
{% endfor %}
By appending |dictlookup:item.NAME
to mydict
, we're now telling Django to treat item.NAME
as the desired key and perform the lookup accordingly. Problem solved! š
The Bottom Line
Now that you have this handy solution in your toolkit, you don't need to fret when it comes to looking up dictionary values using variables in Django templates. Remember to utilize the dictlookup
template filter and pass in the variable as the key. With this knowledge, you're all set to conquer any Django template challenge that comes your way! š„
So, what are you waiting for? Go ahead and try implementing this solution in your code. Don't forget to share your experiences or ask any questions in the comments section below. Happy coding! āØ