Django set default form values
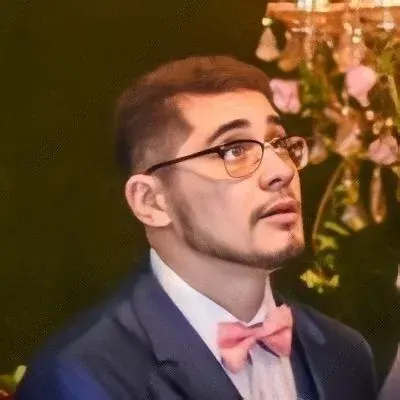
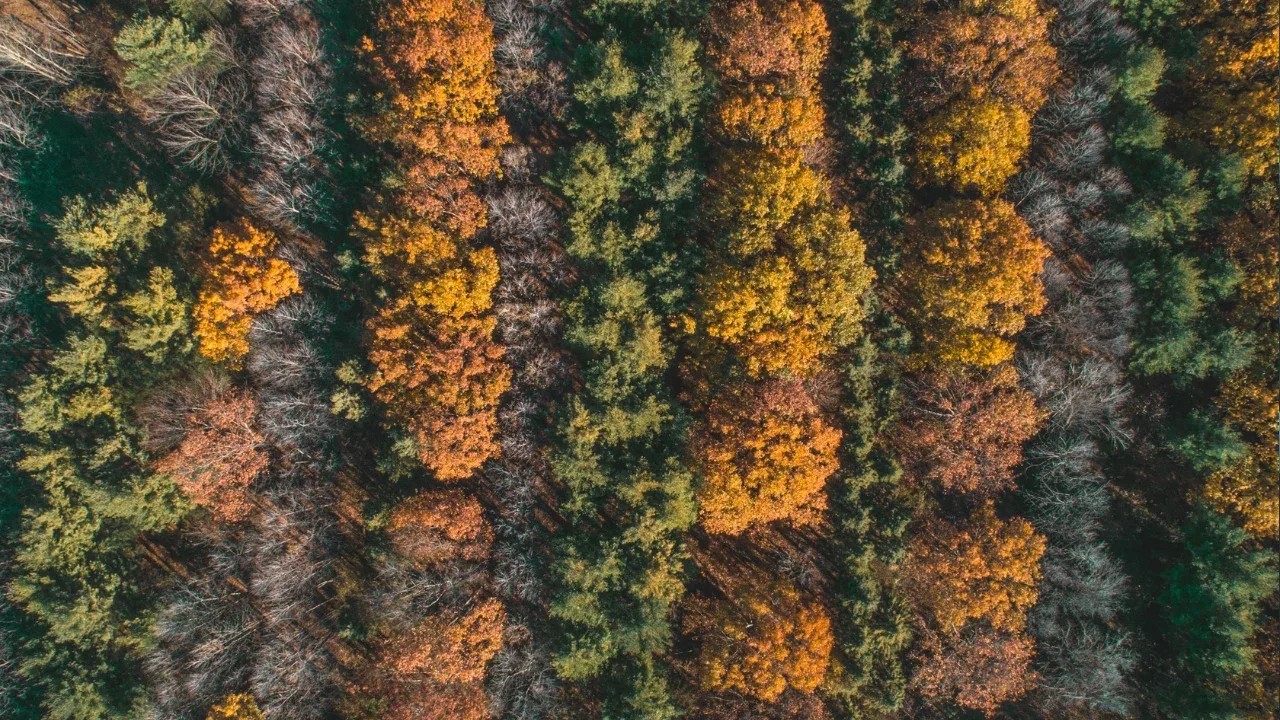
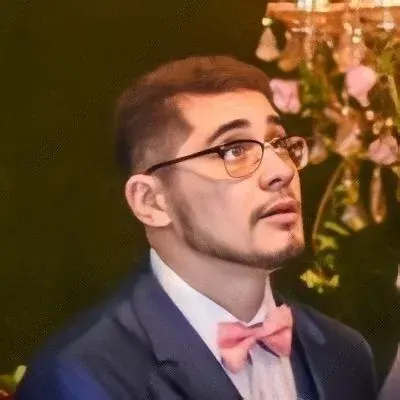
Django Set Default Form Values: A Complete Guide for Beginners
If you're working with Django and have encountered the need to set default form values, you're in the right place! In this guide, we'll address common issues and provide easy solutions to help you breeze through this process. 💪🚀
The Problem
Let's start by understanding the problem at hand. In the provided context, we have a TankJournal
model and a corresponding JournalForm
model form. The challenge is to set a default value for the tank
field, which happens to be a hidden field in this case.
The Solution
To tackle this problem, we'll walk through the code and explain the necessary steps.
Step 1: Add a Default Value to the Form Field
In our JournalForm
class, we need to specify a default value for the hidden tank
field. We can achieve this by modifying the form's __init__
method. Here's an updated version of the form:
class JournalForm(ModelForm):
tank = forms.IntegerField(widget=forms.HiddenInput(), initial=DEFAULT_TANK_ID)
class Meta:
model = TankJournal
exclude = ('user','ts')
In the above code snippet, DEFAULT_TANK_ID
represents the default value you want to set for the hidden tank
field. Replace DEFAULT_TANK_ID
with the desired default value.
Step 2: Update the View Function
In the provided addJournal
view function, we'll make some modifications to ensure that the default value is correctly passed to the form.
def addJournal(request, id=0):
if not request.user.is_authenticated():
return HttpResponseRedirect('/')
# Checking if they own the tank
from django.contrib.auth.models import User
user = User.objects.get(pk=request.session['id'])
if request.method == 'POST':
form = JournalForm(request.POST)
if form.is_valid():
obj = form.save(commit=False)
# Setting the user and ts
from time import time
obj.ts = int(time())
obj.user = user
obj.tank = TankProfile.objects.get(pk=form.cleaned_data['tank'])
# Saving the test
obj.save()
else:
form = JournalForm(initial={'tank': DEFAULT_TANK_ID})
try:
tank = TankProfile.objects.get(user=user, id=id)
except TankProfile.DoesNotExist:
return HttpResponseRedirect('/error/')
In the else
block of the view function, we set the initial value for the tank
field by passing it as a dictionary to the form's initial
parameter. Replace DEFAULT_TANK_ID
with the desired default value.
Call-to-Action
Congratulations! 🎉 You've successfully learned how to set default form values in Django. Make sure to apply these steps whenever you encounter a similar situation. If you have any questions or face any issues, feel free to reach out to the Django community for assistance.
If you found this guide helpful, share it with your fellow Django enthusiasts. Let's spread the knowledge and empower more developers! 💻🌟
#YourTurn
Have you faced any challenges while working with Django forms? How did you solve them? Share your experiences and let's learn from each other in the comments below!