Django self-referential foreign key
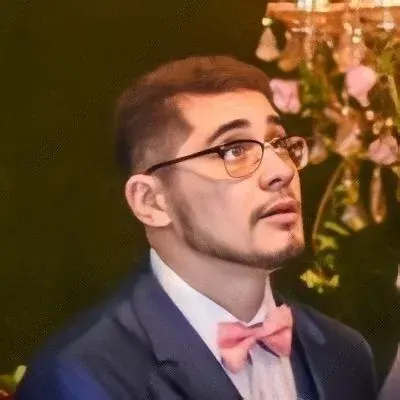
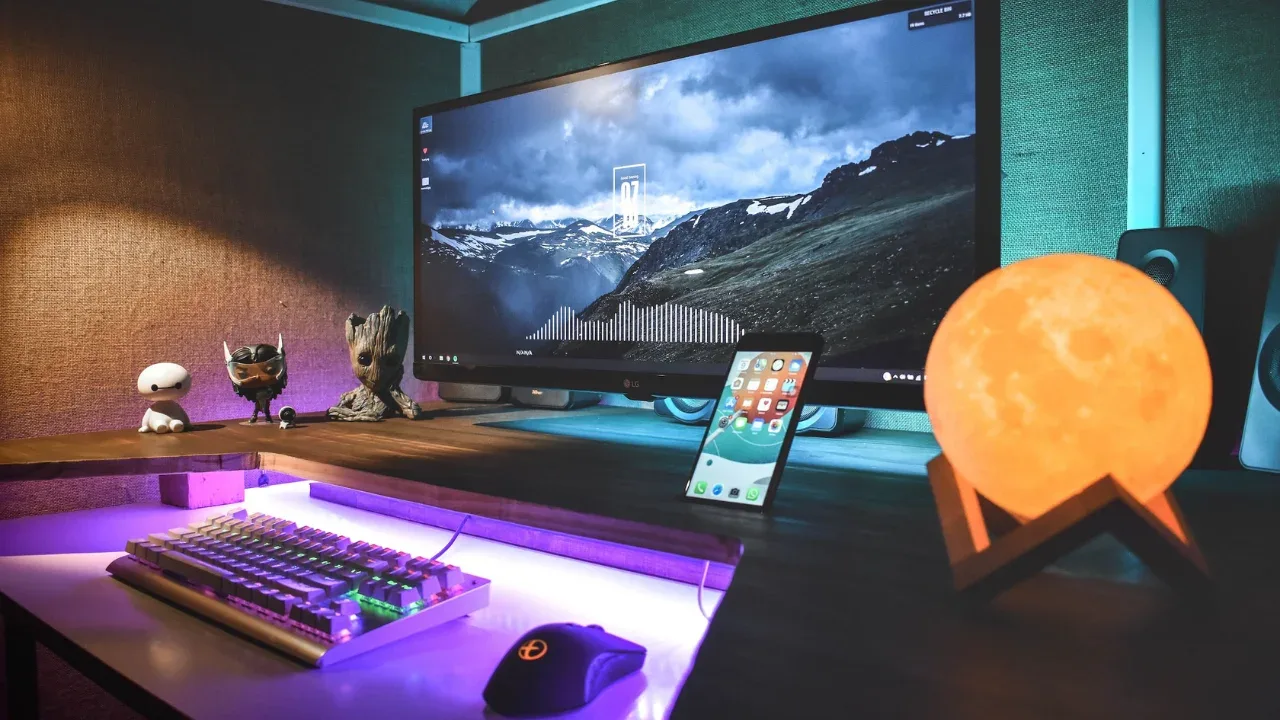
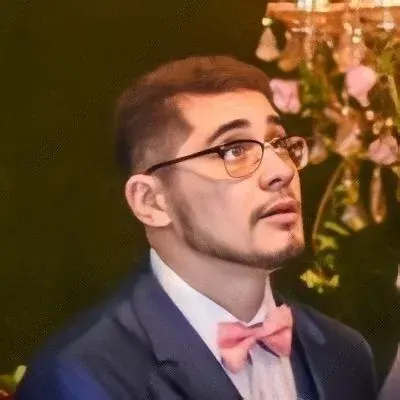
Django Self-Referential Foreign Key: A Guide for Newbies 👥
Are you new to web apps and the world of databases? Don't worry, we've got your back! In this blog post, we'll tackle a common question many beginners ask: How do you create a self-referential foreign key in Django? 🤔
Understanding the Problem 🤔
The question you posed is not dumb at all! In fact, it's quite common to have a model that needs to reference another instance of the same model. In your case, the CategoryModel
needs to have a field (parent
) that points to another CategoryModel
instance.
The Solution 💡
To create a self-referential foreign key in Django, you can follow these steps:
Import the necessary modules:
from django.db import models
Define your model, making use of the
models.ForeignKey
field:
class CategoryModel(models.Model):
parent = models.ForeignKey('self', null=True, blank=True, on_delete=models.CASCADE)
We set the
on_delete
parameter tomodels.CASCADE
to specify what happens when a referencedCategoryModel
is deleted. In this case, we delete the instance along with its children.We set
null=True
andblank=True
to allow theparent
field to be optional. This way, the root categories (those without a parent) can be defined without a foreign key reference.
Apply the changes to your database by running the migrations:
python manage.py makemigrations
python manage.py migrate
That's it! You've successfully created a self-referential foreign key in Django. 🎉
Example Usage 🌟
To better understand how this works, let's imagine a scenario where we have a CategoryModel
representing a tree-like structure of categories:
from django.db import models
class CategoryModel(models.Model):
name = models.CharField(max_length=100)
parent = models.ForeignKey('self', null=True, blank=True, on_delete=models.CASCADE)
def __str__(self):
return self.name
Now, we can create categories and set their parent-child relationships:
# Creating root categories
category1 = CategoryModel.objects.create(name='Technology')
category2 = CategoryModel.objects.create(name='Sports')
# Creating child categories
sub_category1 = CategoryModel.objects.create(name='Web Development', parent=category1)
sub_category2 = CategoryModel.objects.create(name='Software Engineering', parent=category1)
sub_category3 = CategoryModel.objects.create(name='Football', parent=category2)
In this example, category1
and category2
are root categories, while sub_category1
, sub_category2
, and sub_category3
are child categories.
Stay Curious! 🐱🏍
Congratulations on learning how to create a self-referential foreign key in Django! We hope this guide has helped you understand the concept better. Remember, the key to mastering new technologies is to stay curious and keep exploring.
If you have any more questions or need further clarification, feel free to leave a comment below. Happy coding! 💻