Django: Redirect to previous page after login
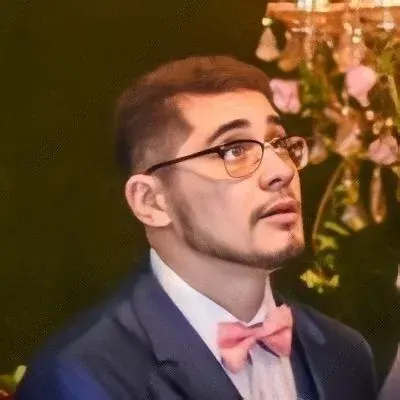
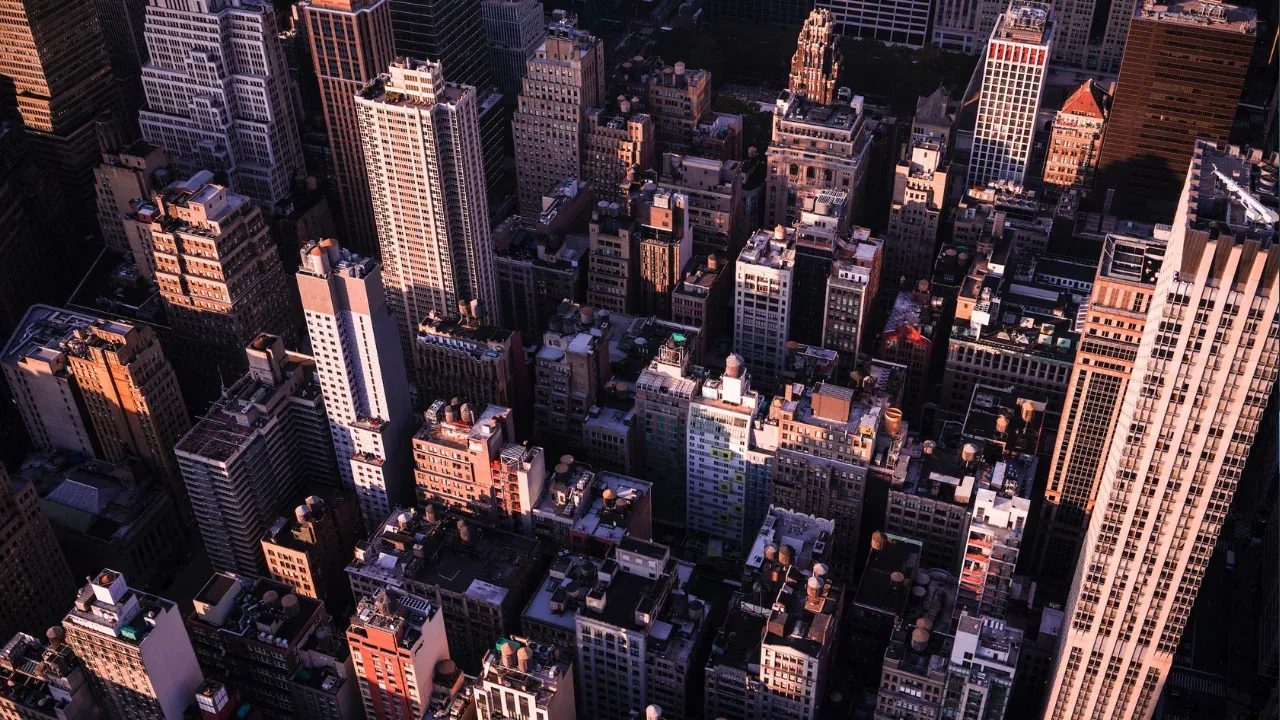
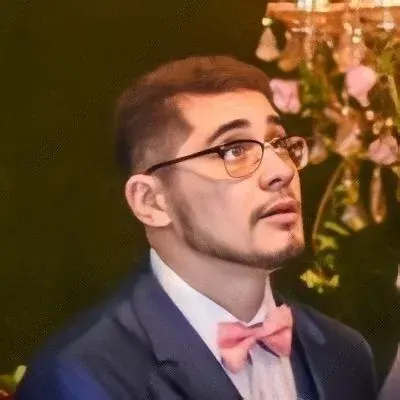
Django: Redirect to previous page after login
Are you building a website with login functionality, and want to redirect users back to the page they were on after they successfully login? Look no further! In this blog post, I will walk you through the steps to achieve this in Django.
The Problem
\🤔 Let's say you have a website where users can browse anonymously, and there is a login link on every page. When a user clicks on the login link, they should be taken to the login form. After a successful login, the user should be redirected back to the page they were on when they clicked the login link.
The Solution
\💡 Thankfully, Django makes it quite easy to handle this scenario. Here's how you can do it:
First, you need to pass the URL of the current page to the login view. You can do this by appending the
next
parameter to the login URL. For example:
<a href="/login/?next={{ request.path }}">Login</a>
In the above code snippet, {{ request.path }}
represents the current page's URL. When the user clicks on the login link, they will be redirected to the login page with the next
parameter containing the URL of the current page.
Next, you need to update the login view to handle the redirect. Here's an example implementation:
def login_view(request):
redirect_to = request.GET.get('next', '') # retrieves the 'next' parameter from the URL
if request.method == 'POST':
# create login form...
if valid_login_credentials_have_been_entered:
return HttpResponseRedirect(redirect_to)
# ...
return render(request, 'login.html')
In the code snippet above, we retrieve the next
parameter from the URL using request.GET.get('next', '')
. If the form is submitted and the login credentials are valid, we redirect the user to the URL specified by the next
parameter.
Lastly, you need to update the login form in the
login.html
template to include thenext
parameter in the form's action attribute. Here's an example:
<form method="post" action="./?next={{ redirect_to }}">
<!-- login form fields... -->
<input type="submit" value="Login">
</form>
In the code snippet above, we include the next
parameter in the form's action attribute. This ensures that when the form is submitted, the user will be redirected back to the page specified by the next
parameter.
And voila! With these changes, users will be redirected back to the page they were on after a successful login.
Conclusion
\🎉 You've now learned how to redirect users to the previous page after they login in Django. It's a simple but powerful feature that enhances the user experience on your website. Give it a try and see how it improves your login flow!
If you have any questions or face any challenges along the way, feel free to leave a comment below. Happy coding!