Django model "doesn"t declare an explicit app_label"
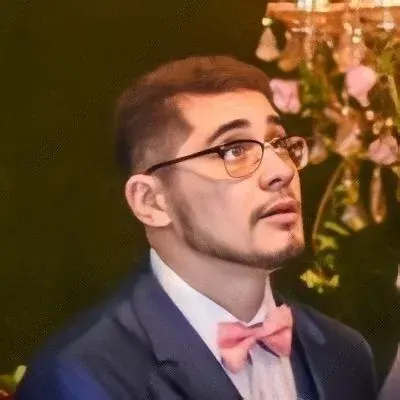
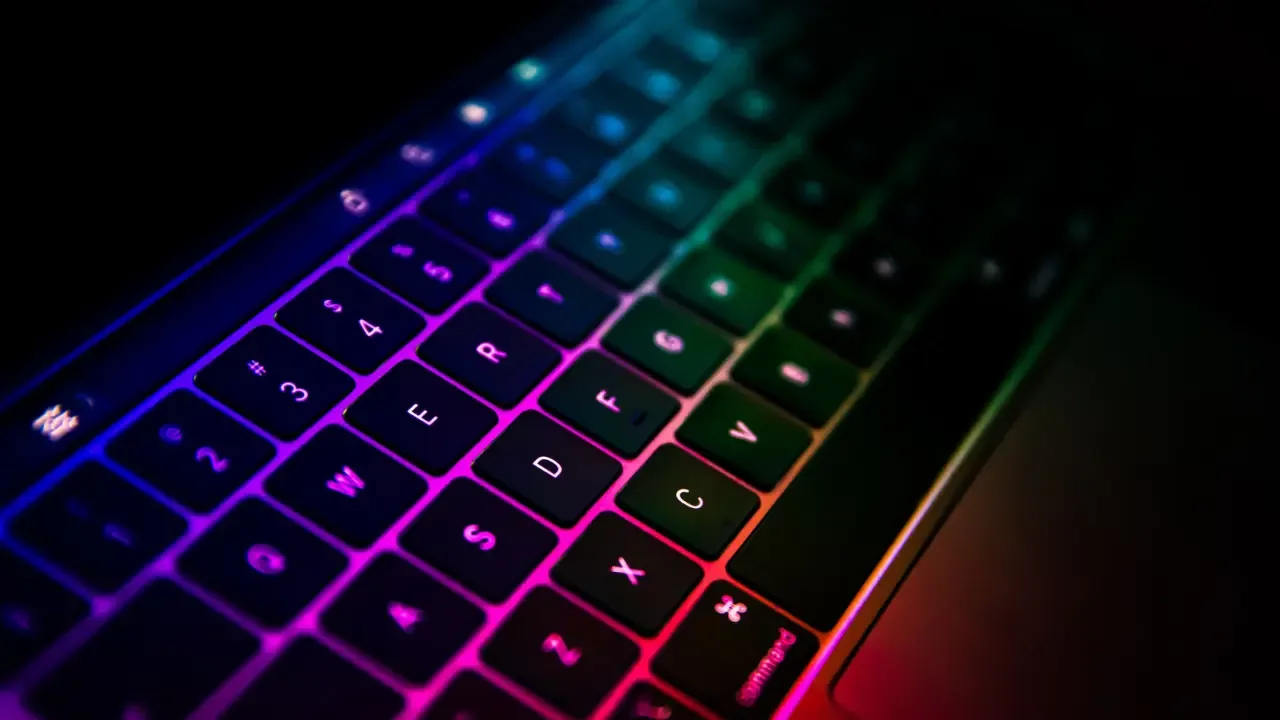
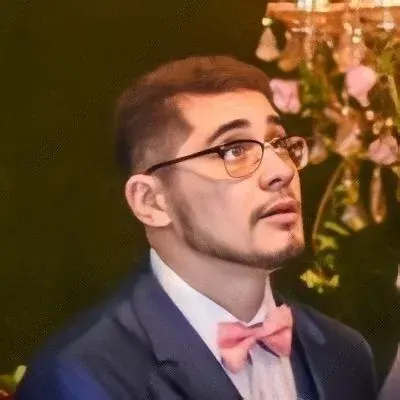
Django Model "doesn't declare an explicit app_label" Error
So, you've encountered the dreaded "Model class doesn't declare an explicit app_label" error in Django. It's frustrating, I know! But don't worry, I'm here to help you understand what's causing this issue and guide you through some easy solutions.
Understanding the Problem
This error message usually occurs when Django cannot determine the app label for a model. An app label is a unique identifier for your Django app, which helps Django distinguish between different apps in your project.
Possible Causes
There are a few common causes for this error:
Incorrect app name in
INSTALLED_APPS
: Double-check that you have correctly specified the app name in yourINSTALLED_APPS
setting in thesettings.py
file.Missing or incorrect
AppConfig
class in your app'sapps.py
file: Make sure you have defined theAppConfig
class and specified the correctname
attribute. This class is responsible for providing metadata about your app to Django.
Easy Solutions
Now that we understand the problem, let's explore some solutions:
Solution 1: Check INSTALLED_APPS
First, make sure that you have added your app to the INSTALLED_APPS
list in the settings.py
file. In your case, the relevant portions of INSTALLED_APPS
should look like this:
INSTALLED_APPS = [
'DeleteNote.apps.DeletenoteConfig',
'LibrarySync.apps.LibrarysyncConfig',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
Solution 2: Verify AppConfig
Class
Take a look at your apps.py
files for both the DeleteNote
and LibrarySync
apps. Ensure that the AppConfig
class is correctly defined with the name
attribute set to match your app's label.
For example, your apps.py
files should look like this:
from django.apps import AppConfig
class DeletenoteConfig(AppConfig):
name = 'DeleteNote'
And:
from django.apps import AppConfig
class LibrarysyncConfig(AppConfig):
name = 'LibrarySync'
Solution 3: Check for Typos
Sometimes, a simple typo can be the cause of this error. Double-check for any spelling mistakes or case-sensitive errors in your app names, whether in INSTALLED_APPS
or the AppConfig
classes.
Wrapping Up
Hopefully, one of these solutions helped you resolve the "Model class doesn't declare an explicit app_label" error in Django. Remember to save your changes and restart your server to apply any modifications.
If you're still facing issues or have any other questions, feel free to leave a comment below. I'll be more than happy to assist you!
Now, go ahead and dive back into your Django project with confidence! 💪🌟
Share your experience: Have you encountered this error before? How did you solve it? Let me know in the comments below. Let's learn from each other!