Django migration strategy for renaming a model and relationship fields
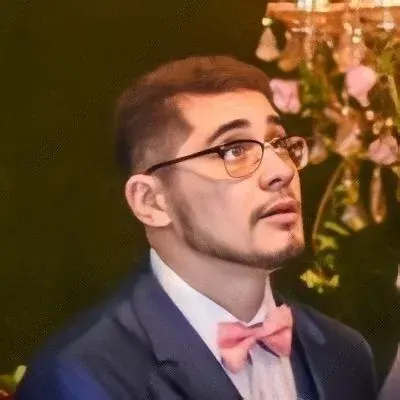
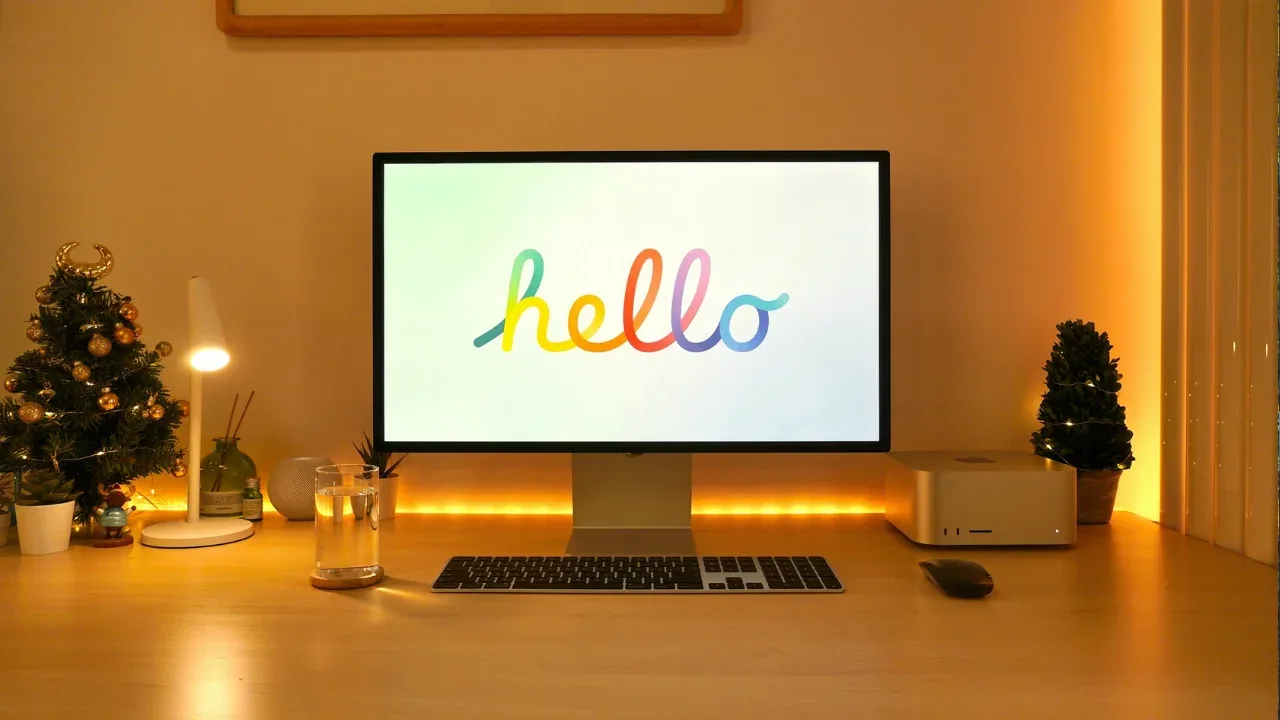
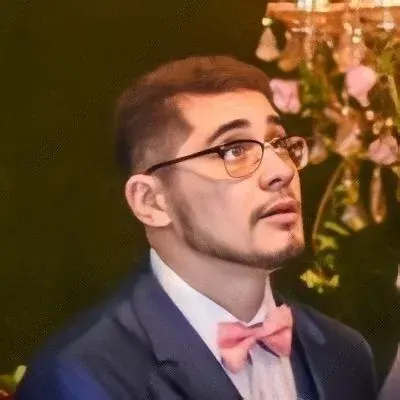
A Comprehensive Guide to Renaming Models and Relationship Fields in Django Migrations
So you want to rename a model and its related fields in your Django project? No problem! In this guide, we'll walk through the step-by-step process to help you achieve that. Let's dive in!
The Scenario
Let's start with a scenario to better understand what we aim to accomplish. Suppose we have the following models in a Django app called myapp
:
class Foo(models.Model):
name = models.CharField(unique=True, max_length=32)
description = models.TextField(null=True, blank=True)
class AnotherModel(models.Model):
foo = models.ForeignKey(Foo)
is_awesome = models.BooleanField()
class YetAnotherModel(models.Model):
foo = models.ForeignKey(Foo)
is_ridonkulous = models.BooleanField()
In this case, we want to rename the Foo
model to Bar
because the name isn't clear and causes confusion in the code. We also need to update the related fields throughout the project to maintain proper relationships.
The Migration Strategy
To achieve the desired renaming, we'll follow these steps:
Step 1: Modify models.py
First, we need to modify models.py
to reflect the changes. Update the code as follows:
class Bar(models.Model): # <-- changed model name
name = models.CharField(unique=True, max_length=32)
description = models.TextField(null=True, blank=True)
class AnotherModel(models.Model):
foo = models.ForeignKey(Bar) # <-- changed relation, but not field name
is_awesome = models.BooleanField()
class YetAnotherModel(models.Model):
foo = models.ForeignKey(Bar) # <-- changed relation, but not field name
is_ridonkulous = models.BooleanField()
Notice that we changed the model name from Foo
to Bar
. However, we didn't change the field name foo
in the AnotherModel
and YetAnotherModel
models to bar
to avoid losing the existing data in those fields.
Step 2: Create an Empty Migration
Next, we'll create an empty migration to handle the model renaming. Run the following command in your terminal:
python manage.py makemigrations --empty myapp
Step 3: Edit the Migration File
Open the migration file created in the previous step (000X_initial.py
), and locate the Migration
class. Add the RenameModel
operation to the operations
list:
class Migration(migrations.Migration):
dependencies = [
('myapp', '0001_initial'),
]
operations = [
migrations.RenameModel('Foo', 'Bar')
]
By adding the RenameModel
operation, Django knows that we want to rename the model from Foo
to Bar
.
Step 4: Apply the Migration
It's time to apply the migration by running the following command:
python manage.py migrate
This will execute the migration and rename the model in the database.
Step 5: Edit the Related Field Names
Now, let's go back to models.py
and update the field names to match the renamed model:
class Bar(models.Model):
name = models.CharField(unique=True, max_length=32)
description = models.TextField(null=True, blank=True)
class AnotherModel(models.Model):
bar = models.ForeignKey(Bar) # <-- changed field name
is_awesome = models.BooleanField()
class YetAnotherModel(models.Model):
bar = models.ForeignKey(Bar) # <-- changed field name
is_ridonkulous = models.BooleanField()
We changed the field names foo
to bar
in both the AnotherModel
and YetAnotherModel
models to ensure consistency with the renamed model.
Step 6: Create Another Empty Migration
To handle the field renaming, let's create another empty migration. Run the following command:
python manage.py makemigrations --empty myapp
Step 7: Edit the Migration File (Again)
Open the new migration file (000Y_rename_fields.py
) and locate the Migration
class. Add the RenameField
operation(s) for any related field names to the operations
list:
class Migration(migrations.Migration):
dependencies = [
('myapp', '0002_rename_fields'), # <-- the previous migration file
]
operations = [
migrations.RenameField('AnotherModel', 'foo', 'bar'),
migrations.RenameField('YetAnotherModel', 'foo', 'bar')
]
By adding the RenameField
operation(s), we inform Django to rename the field(s) from foo
to bar
in the respective models.
Step 8: Apply the Second Migration
Finally, run the migration command to apply the second migration:
python manage.py migrate
The second migration will update the field names in the database to match the changes made in models.py
.
Conclusion
Congratulations! You've successfully renamed a model and its related fields in your Django project using migrations. 🎉
Remember to update the rest of your code, such as views, forms, and templates, to reflect the new variable names. And if you encounter any issues along the way, don't hesitate to refer to the Django documentation or seek help from the Django community.
So go ahead, give it a try! Share your experience and let us know if this guide helped you in the comments below. Happy coding! 💻💪
Looking for more informative Django guides? Check out our blog for a plethora of articles to level up your Django skills!