Django datetime issues (default=datetime.now())
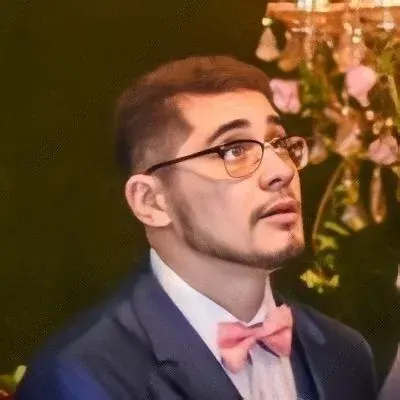
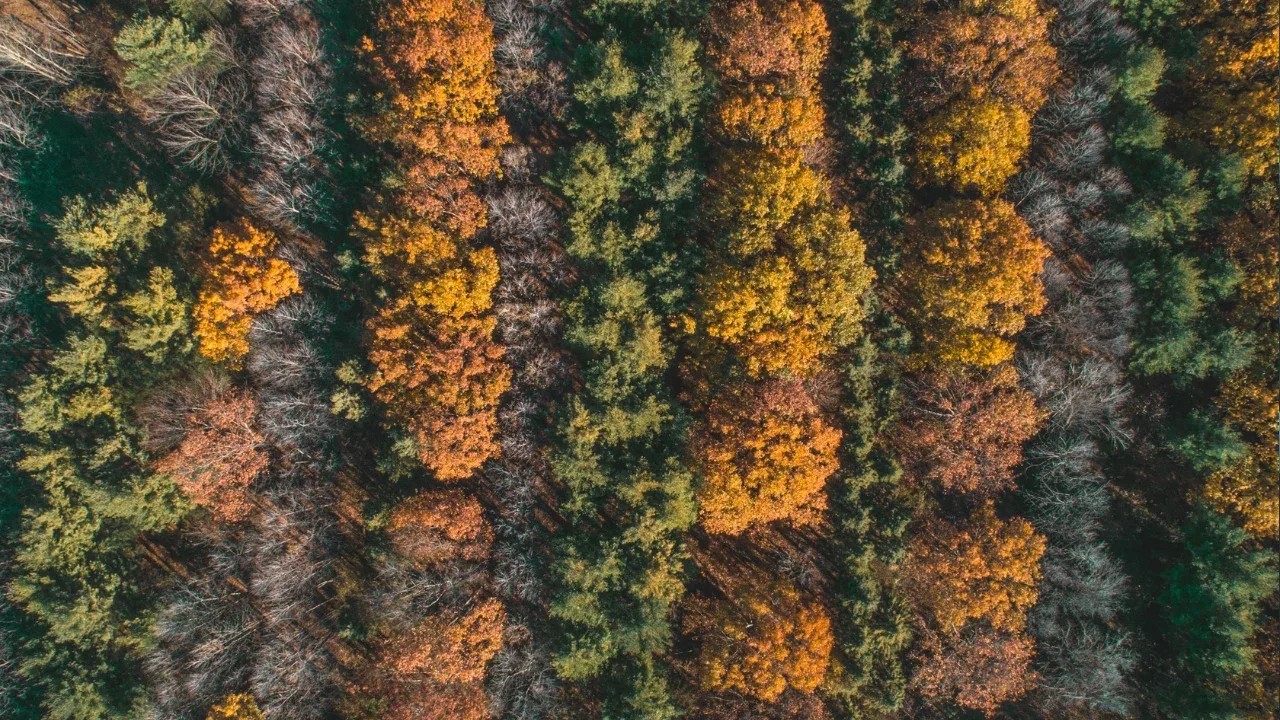
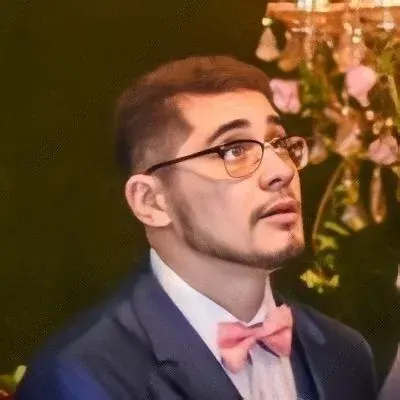
Django Datetime Issues: How to Solve the "Same Date for All Records" Problem
Are you experiencing the frustrating issue where all records in your Django application have the same date value, even though you set the default value to datetime.now()
? 📅😞 Don't worry! You're not alone. This is a common problem faced by many Django developers, and we have an easy solution for you. 🎉🔧
Understanding the Issue
Let's take a look at the code snippet that exhibits the issue:
from datetime import datetime
class TermPayment(models.Model):
date = models.DateTimeField(default=datetime.now(), blank=True)
You might be thinking, "This should work, right?" Unfortunately, it won't behave as expected. The default
value is evaluated when the model is defined, which means that it will use the same datetime value for all records. 😱
The Solution: Use a Callable as the Default Value
To solve this issue, we need to use a callable as the default value instead of a fixed value. ✨💡
from datetime import datetime
class TermPayment(models.Model):
date = models.DateTimeField(default=datetime.now, blank=True)
By removing the parentheses after datetime.now
, we pass the callable datetime.now
itself rather than its evaluated value. This ensures that each record gets its own current date and time when it is created. 🕒⚙️
Explanation
Now, you might be wondering why this change makes a difference. Let's break it down:
Previous Code:
The
datetime.now()
is evaluated only once when the model is defined.The evaluated value becomes the default value for all new records.
Any new record created will have the same date and time as the first evaluation.
Updated Code:
The
datetime.now
is a callable function that gets evaluated each time a new record is created.Every time a new record is created, it will use the current date and time.
Take It a Step Further
If you want to have even more control over the default value, you can use the timezone.now
method from Django's timezone module instead. This method takes into account the time zone settings specified in your Django project. 🌍🕒
from django.utils import timezone
class TermPayment(models.Model):
date = models.DateTimeField(default=timezone.now, blank=True)
Now, your default value will be aware of the correct time zone and adapt accordingly. 🌐
Have You Faced Other Django Issues?
If you've encountered other Django issues or have any questions regarding Django development, feel free to reach out to us in the comments section below. We're here to help you out! 💪❓
Happy coding! 💻🚀