Display number with leading zeros
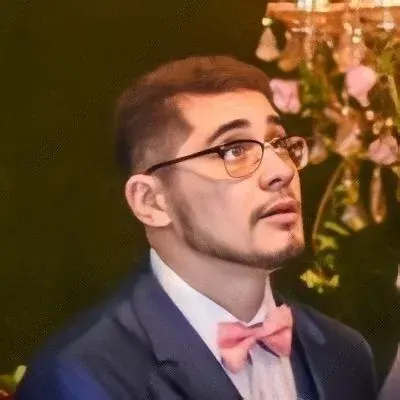
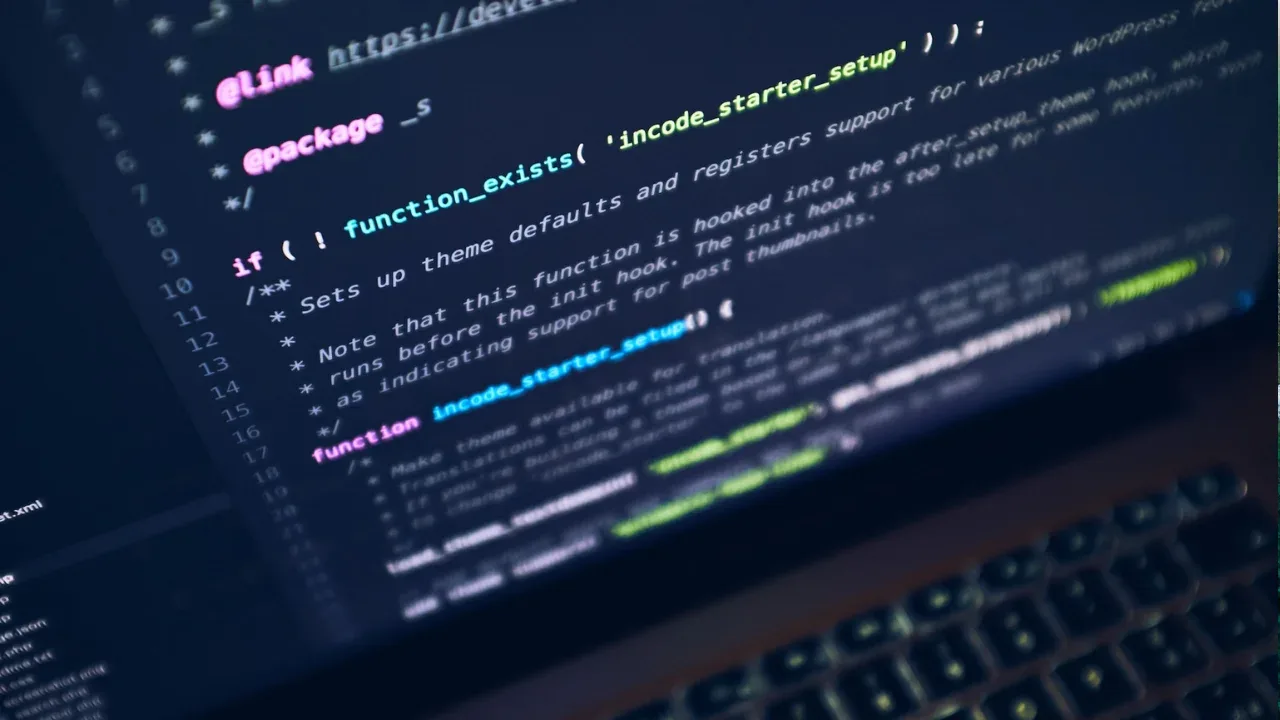
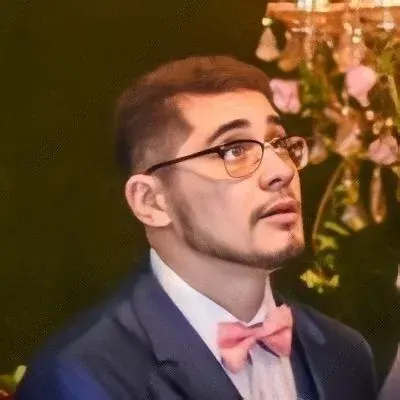
Display Number with Leading Zeros: Get it Right Every Time! 😎
So, you want to display numbers with leading zeros, huh? 🤔
Whether you're dealing with dates, phone numbers, or any other scenario where maintaining a consistent format is crucial, adding leading zeros can make all the difference. But fear not, my tech-savvy friend! 😄 In this guide, I'll walk you through some common issues, provide you with easy-to-implement solutions, and give you a compelling call-to-action that'll leave you feeling like a coding rockstar! 🚀
The Problem: How do I display a leading zero for all numbers with less than two digits?
Let's take a closer look at the problem at hand. You want to display numbers with at least two digits, and if the numbers have only one digit, you want to add a leading zero.
Here are some examples to illustrate what we're dealing with:
<pre class="lang-none prettyprint-override"><code>1 → 01 10 → 10 100 → 100 </code></pre>
Solution 1: The Almighty String Method 💪
One simple and elegant solution to this conundrum is to utilize the power of string manipulation. Most programming languages have some sort of method or function that allows you to convert numbers to strings and easily manipulate them.
Let's look at an example using JavaScript:
const num = 1; // Our original number
const formattedNum = num.toString().padStart(2, '0');
console.log(formattedNum); // Output: '01'
By using the toString()
method, we convert our number into a string. Then, we call the padStart()
method on our string and provide two arguments: the desired length (2) and the character ('0') we want to use for padding. Easy peasy, right? 😄
Solution 2: Formatting Magic with printf() (C/C++ and Other Languages) ✨
If you're working with languages like C or C++ (or with other languages that support the printf()
function), you're in for a treat!
// C program example
#include <stdio.h>
int main() {
int num = 1; // Our original number
printf("%02d", num); // Output: '01'
return 0;
}
By using the %02d
format specifier, we tell printf()
to display our number with a minimum width of 2 characters, padded with zeros if necessary. It's like magic!
Call-to-Action: Level up your formatting game! 📈
Now that you know how to display numbers with leading zeros, it's time to put your knowledge into action! Think about the projects you're working on and how this technique could improve the user experience or make your code more professional.
Share your thoughts, ideas, or even your own coding challenges in the comments below! Let's keep the conversation going and help each other become better coders. Happy coding! 😊💻✨
Did you find this blog post helpful? Be sure to share it with your fellow developers and spread the knowledge!