Difference between map, applymap and apply methods in Pandas
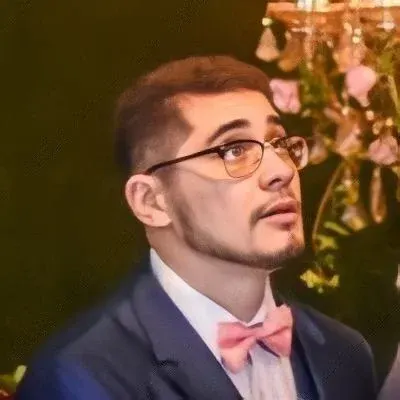
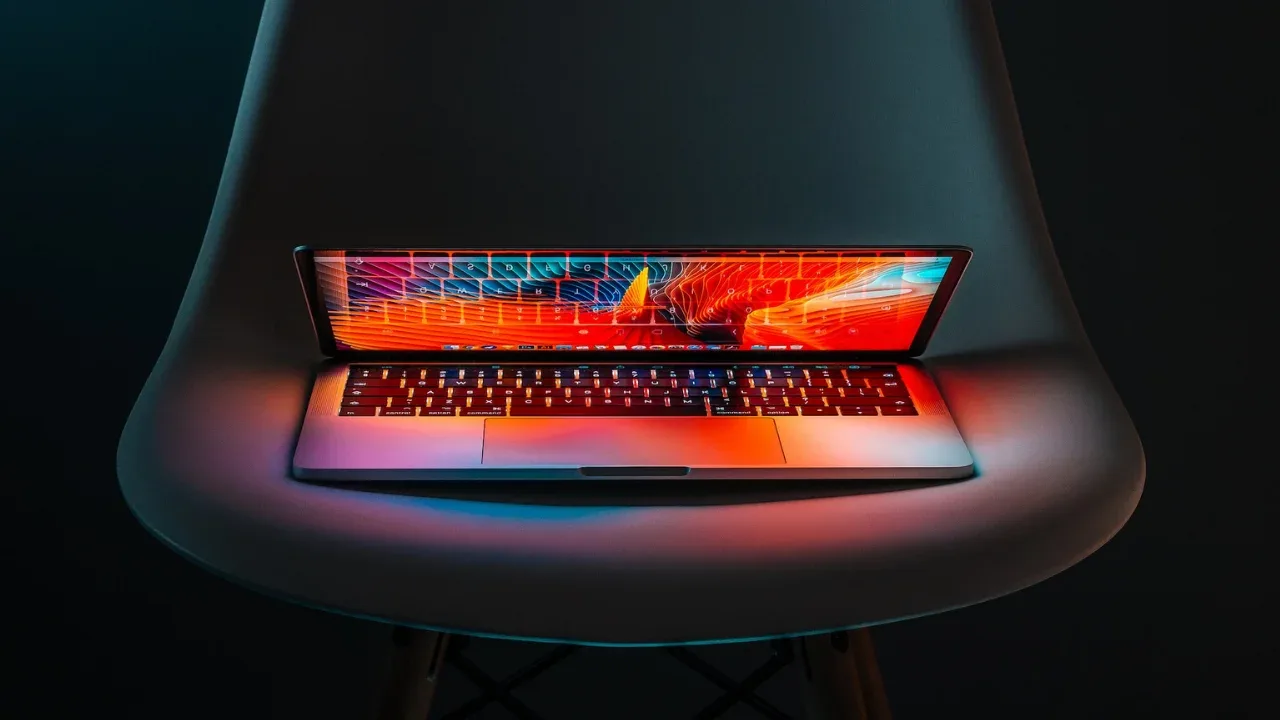
π Understanding map, applymap and apply methods in Pandas
Pandas is a powerful library widely used for data manipulation and analysis in Python. It provides various methods to efficiently work with Series and DataFrames. In this blog post, we will demystify the differences between three important methods: map
, applymap
, and apply
.
πΊοΈ Mapping your way with map
The map
method in Pandas is used to substitute each value in a Series with another value. It takes a dictionary or a function as an argument and applies it element-wise to the Series. This method is only available for Series objects.
π‘ Pro Tip: map
is a great choice when you want to change values based on a specific condition or apply simple transformations to a single column in a DataFrame.
Let's say we have a Series of student grades:
grades = pd.Series(['A', 'B', 'C', 'D', 'A'])
And we want to convert these grades into their corresponding numeric values:
grade_map = {'A': 5, 'B': 4, 'C': 3, 'D': 2, 'F': 1}
numeric_grades = grades.map(grade_map)
The resulting numeric_grades
Series would be:
0 5
1 4
2 3
3 2
4 5
dtype: int64
ποΈ Applying function element-wise with applymap
applymap
is a DataFrame method that applies a function to every element in the DataFrame. The function can be either a NumPy ufunc or a Python function. Unlike map
, applymap
allows us to modify every single value in the DataFrame.
π Cool Feature Alert: applymap
is particularly useful when you need to perform element-wise calculations or transformations on the entire DataFrame.
Let's say we have a DataFrame representing the temperatures in Celsius:
temps = pd.DataFrame({'City': ['New York', 'Paris', 'Tokyo'],
'Temperature': [18, 25, 30]})
And we want to convert the temperatures to Fahrenheit:
def celsius_to_fahrenheit(celsius):
return celsius * 9/5 + 32
temps['Temperature'] = temps['Temperature'].applymap(celsius_to_fahrenheit)
The updated DataFrame temps
will be:
City Temperature
0 New York 64.4
1 Paris 77.0
2 Tokyo 86.0
π€ Applying function row-wise with apply
The apply
method is a DataFrame method that applies a function along either axis of the DataFrame. By default, it applies the function column-wise, resulting in a Series. However, you can specify axis=1
to apply the function row-wise, which returns a DataFrame.
π Great to Know: apply
is handy when you need to perform custom operations involving multiple columns or complex calculations that can't be done element-wise.
Let's consider a DataFrame representing sales data:
sales = pd.DataFrame({'Product': ['Apple', 'Orange'],
'Price': [1.99, 2.49],
'Quantity': [10, 15]})
Suppose we want to calculate the total revenue for each product by multiplying the price and quantity:
def calculate_revenue(row):
return row['Price'] * row['Quantity']
sales['Revenue'] = sales.apply(calculate_revenue, axis=1)
The updated DataFrame sales
will be:
Product Price Quantity Revenue
0 Apple 1.99 10 19.90
1 Orange 2.49 15 37.35
π Engage with us!
Understanding the differences between map
, applymap
, and apply
methods in Pandas will help you unleash the power of data manipulation in Python. Start experimenting and incorporating these methods into your data analysis workflows!
If you have any questions or would like to share your own examples of how you use these methods, we'd love to hear from you in the comments below. Happy coding! ππΌβ¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
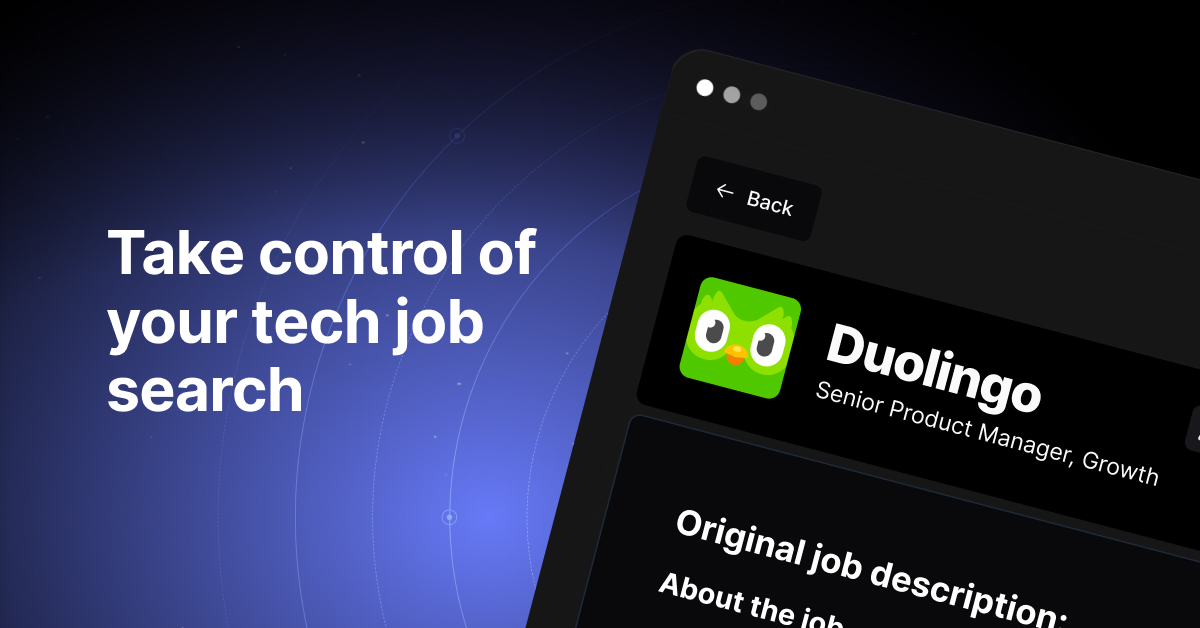