Difference between except: and except Exception as e:
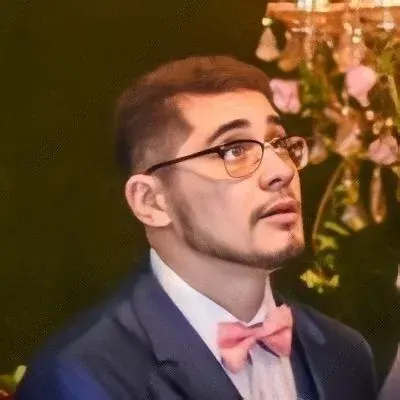
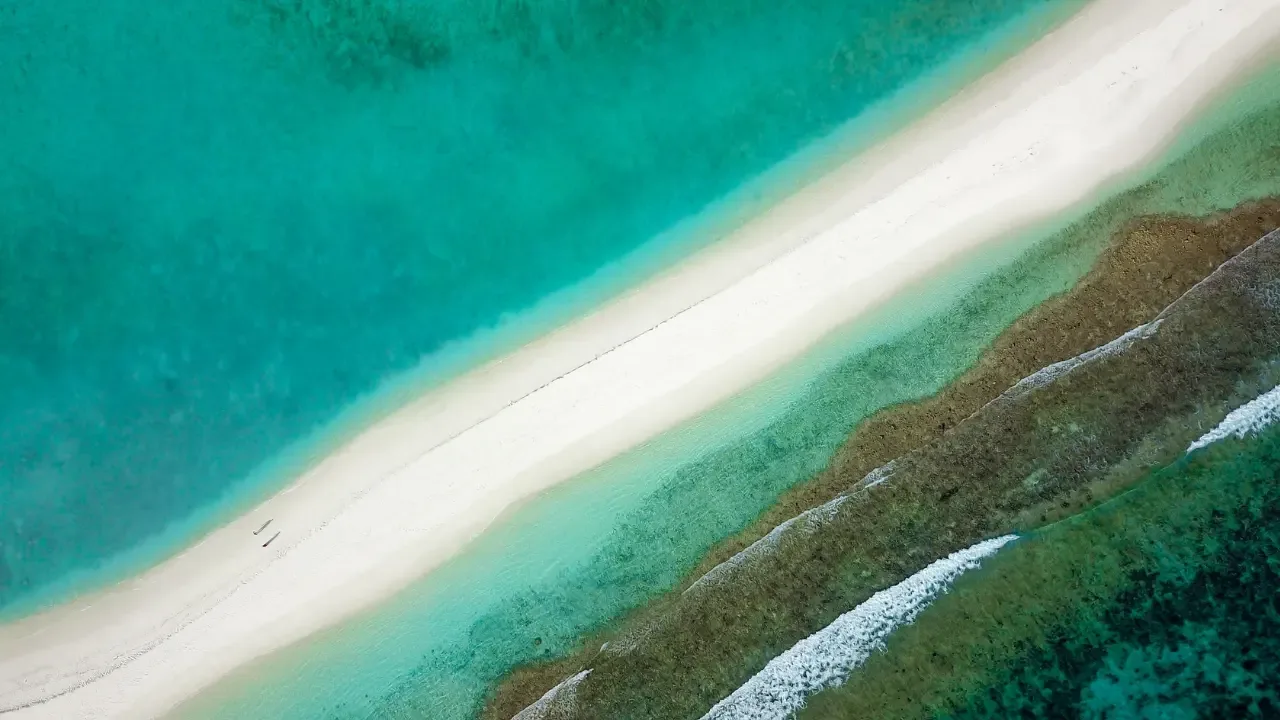
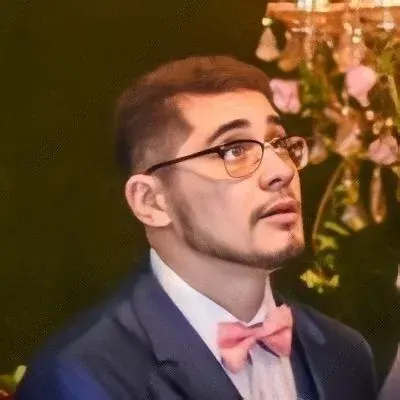
Understanding the Difference: except:
vs except Exception as e:
So you've stumbled upon some code snippets that look almost identical, but have a slight difference. The question on your mind is, what exactly is the difference between except:
and except Exception as e:
?
🔍 The Context
Let's start with some background. Both of these code snippets serve the purpose of catching exceptions and executing specific code in the except:
block. This is crucial for handling errors and preventing our programs from crashing.
📝 Snippet 1 - The Classic Approach
The first snippet of code uses a simple except:
statement without any specific error type. It looks like this:
try:
# some code that may throw an exception
except:
# exception handling code
This construct catches all types of exceptions. It doesn't discriminate between different errors. Whether it's a ValueError
, TypeError
, or any other exception, it will be captured by this generic except:
block.
📝 Snippet 2 - A More Specific Approach
In contrast, the second code snippet introduces a more granular way to catch exceptions. It uses except Exception as e:
:
try:
# some code that may throw an exception
except Exception as e:
# exception handling code
This construct is a bit more powerful as it allows us to handle different types of exceptions separately. By using Exception
as the base class for catching exceptions, we gain access to the specific error type that occurred through the variable e
. This means you can analyze the error, log it, or even display a customized error message based on the specific exception raised.
For example, consider the following snippet:
try:
# some code that may throw an exception
except ValueError as ve:
# handling code for ValueError
except TypeError as te:
# handling code for TypeError
except Exception as e:
# generic handling code for all other exceptions
In this case, we handle ValueError
and TypeError
separately, while still having a fallback except Exception
block to handle any other type of exception not mentioned explicitly.
💡 So, which one should you use?
Both constructs have their pros and cons. Here's a breakdown to help you decide:
except:
✔️ Simplicity: It catches all exceptions without any added complexity.
❌ Lack of granularity: You won't have access to the specific exception type, making it harder to handle different errors with targeted code.
except Exception as e:
✔️ Granularity: It allows you to handle specific exceptions separately, enhancing the clarity and precision of your error handling.
❌ Increased complexity: It requires additional
except
blocks for each type of exception you want to handle.
The decision ultimately depends on the requirements and complexity of your codebase. If you have a simple codebase without many different exception types, the generic except:
approach may suffice. On the other hand, if you need a more fine-grained handling mechanism, except Exception as e:
can be a valuable tool in your error-handling arsenal.
💪 Your Turn to Act!
Now that you understand the difference between except:
and except Exception as e:
, it's time to apply your knowledge. Analyze your existing codebase or start writing new error-handling code using the approach that best suits your needs.
Share your thoughts and experiences with us in the comments section below! Which approach do you prefer, and why? Let's ignite a discussion and learn from each other's insights.
🔗 Looking for More?
If you're hungry for more Python knowledge or want to explore other programming topics, check out our blog for additional guides, tutorials, and tips. Feel free to share this post with your fellow developers who might find it helpful!
Keep coding and may your exceptions always be handled gracefully! 🚀