"dict" object has no attribute "has_key"
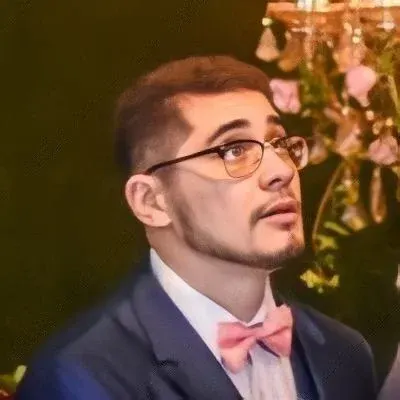
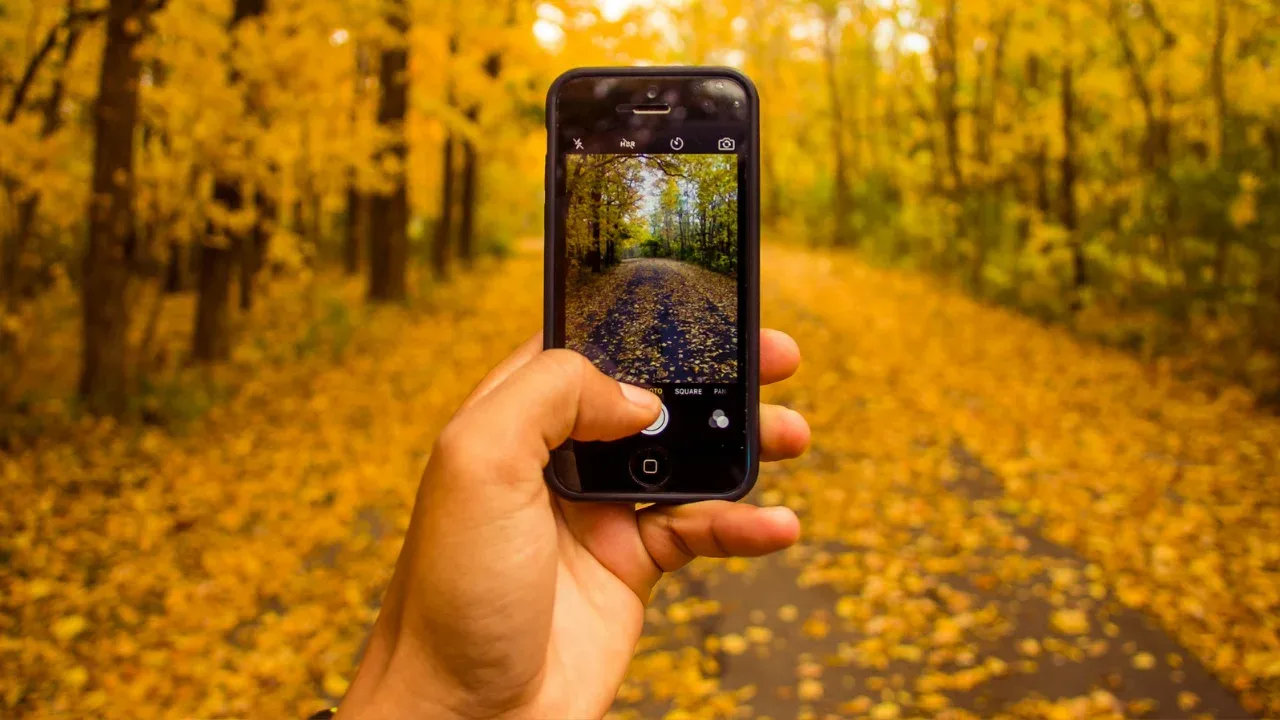
Traversing a Graph in Python: The 'dict' object has no attribute 'has_key'
š Hey there! If you're seeing the error message "'dict' object has no attribute 'has_key'", you've stumbled upon a common issue while traversing a graph in Python. Don't worry, I'll walk you through the problem and provide you with a quick solution. Let's dive in! šāāļø
Understanding the Problem
In Python, dictionaries (dict
objects) play a crucial role when representing graphs. The error message you encountered suggests that the method has_key()
is not available in Python 3.x, causing the code to fail. In earlier versions of Python (2.x), has_key()
was used to check if a key existed in a dictionary. However, in Python 3.x, this method has been removed.
Solution
To fix this issue, you can use the in
keyword to check if a key exists in a dictionary. Here's the modified code with the fix applied:
def find_path(graph, start, end, path=[]):
path = path + [start]
if start == end:
return path
if start not in graph:
return None
for node in graph[start]:
if node not in path:
newpath = find_path(graph, node, end, path)
if newpath:
return newpath
return None
By replacing graph.has_key(start)
with start not in graph
, you ensure that the code functions correctly in Python 3.x without relying on the deprecated has_key()
method.
Explaining the Updated Solution
The code checks if the
start
key is in thegraph
dictionary by usingstart not in graph
. This is the updated way to check for key existence.If the
start
key is not present in thegraph
dictionary,None
is returned. This prevents the code from throwing an error when attempting to accessgraph[start]
.The remaining logic in the code remains the same, ensuring that the path is correctly traversed.
Conclusion
You've learned how to fix the "'dict' object has no attribute 'has_key'" error while traversing a graph in Python. Now you're equipped to handle this problem with ease. Remember, always adapt your code to the version of Python you're using to avoid any compatibility issues.
If you have any questions or suggestions, leave a comment below. Happy coding! šš
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
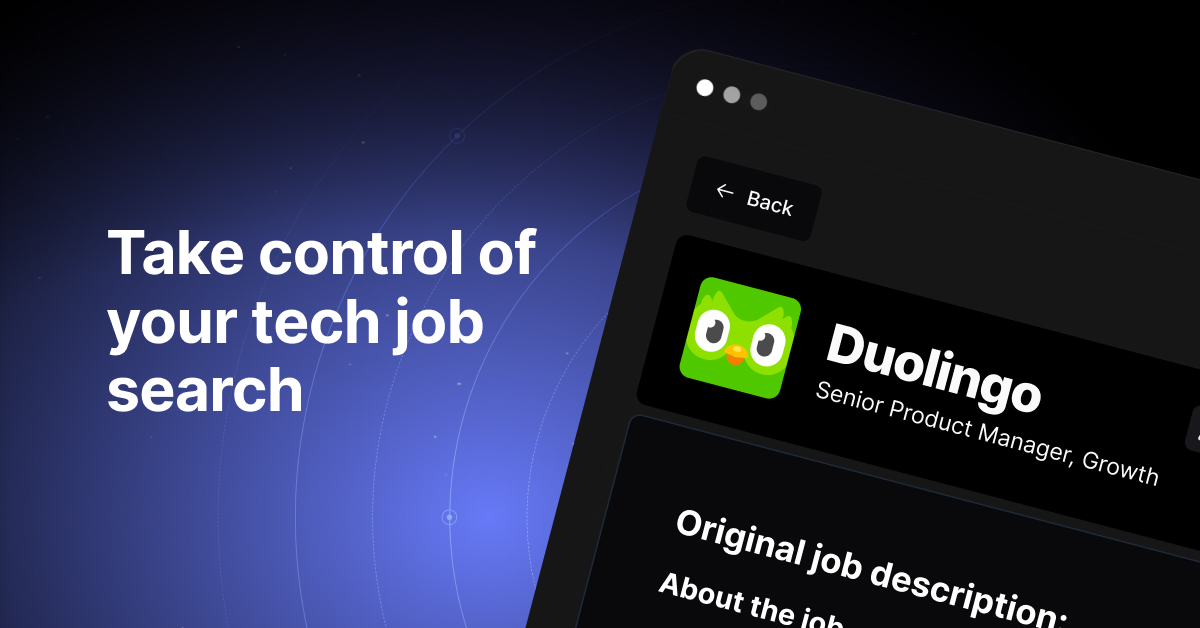