Detect and exclude outliers in a pandas DataFrame
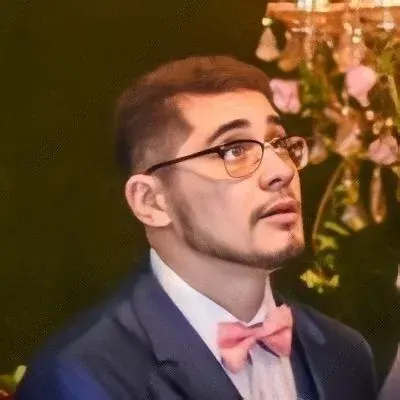
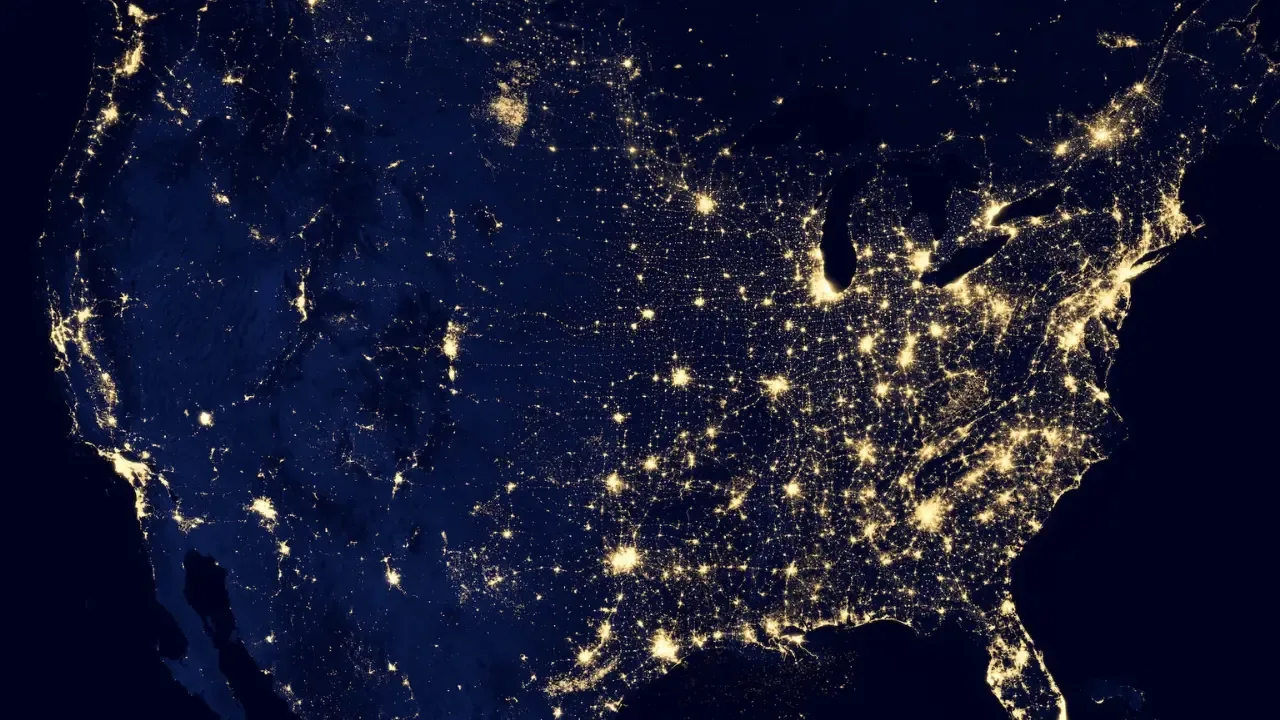
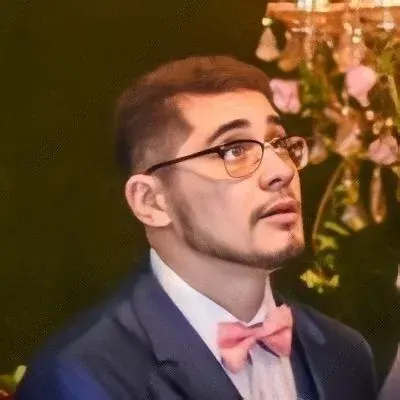
π How to Detect and Exclude Outliers in a pandas DataFrame
Outliers in a dataset can skew our analysis and lead to incorrect conclusions. So, it's crucial to detect and exclude outliers in a pandas DataFrame to ensure accurate insights. In this blog post, we'll address the common issue of identifying outliers based on column values and provide easy solutions to exclude them. Let's dive in!
The Problem: Outliers in a pandas DataFrame
Imagine you have a pandas DataFrame with several columns, but you know that certain rows are outliers based on a specific column value. For example, consider the following scenario:
import pandas as pd
# Create a sample DataFrame
data = {
'Vol': [1200, 1210, 1220, 4000],
'Price': [10, 12, 11, 9]
}
df = pd.DataFrame(data)
In this case, the 'Vol' column has values around 1200, but one value, 4000, stands out as an outlier.
The Solution: Excluding Outliers
To exclude the rows that have outliers in the 'Vol' column, we can apply a filter on the DataFrame. The goal is to select all rows where the values of the column are within a certain range from the mean.
Step 1: Calculate the Mean and Standard Deviation
We need to start by calculating the mean and standard deviation of the 'Vol' column. This information will help us determine the range within which the values are considered normal.
mean = df['Vol'].mean()
std = df['Vol'].std()
Step 2: Define the Outlier Threshold
Next, we can define a threshold to determine which values are outliers. One common approach is to consider values outside a certain number of standard deviations as outliers. Let's say we want to exclude rows where the 'Vol' values are more than 3 standard deviations away from the mean:
threshold = 3
Step 3: Apply the Filter
Now, we can create a filter to exclude the outliers based on our defined threshold. We'll store the filtered DataFrame in a new variable called filtered_df
.
filtered_df = df[abs(df['Vol'] - mean) <= threshold * std]
That's it! The filtered_df
DataFrame will exclude the outlier row, giving you a refined dataset.
Conclusion and Reader Engagement
By following these simple steps, you can easily detect and exclude outliers in a pandas DataFrame. Remember to calculate the mean and standard deviation of the column, define an outlier threshold, and apply the filter. Voila! You'll have a refined DataFrame ready for analysis.
Have you encountered outliers in your datasets? How did you handle them? Share your experiences and insights in the comments below! Let's continue the discussion and learn from each other. π€©
And if you found this blog post helpful, don't forget to share it with your tech-savvy friends or colleagues who might benefit from it. Happy data wrangling! ππΌπͺ