Delete the first three rows of a dataframe in pandas
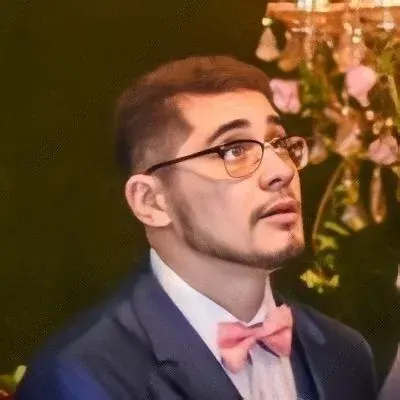
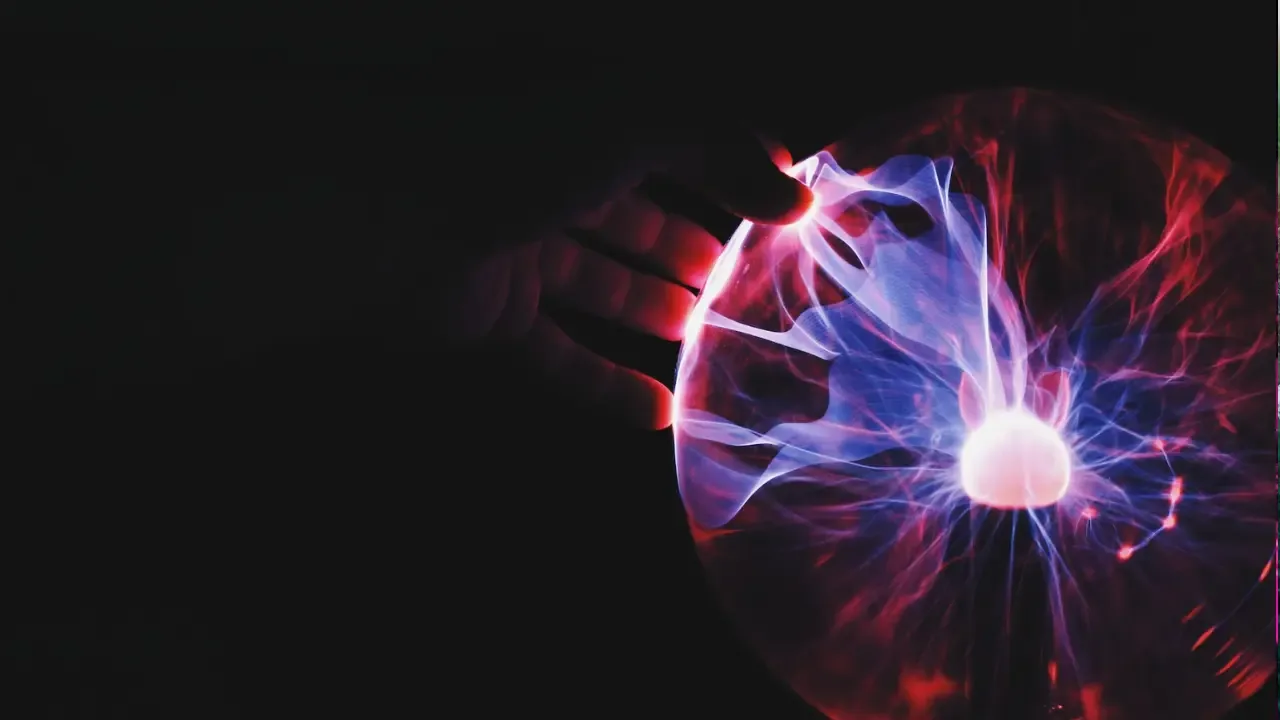
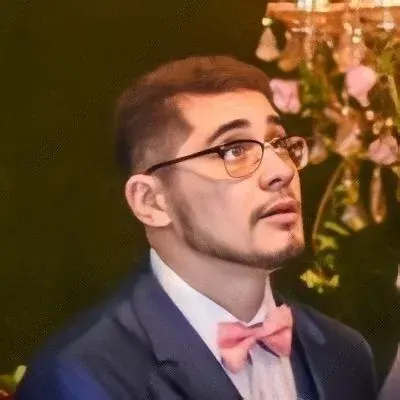
How to Delete the First Three Rows of a Pandas Dataframe 🐼
Deleting rows from a Pandas dataframe is a common task, but deleting the first three rows can be a bit tricky. In this guide, we'll walk you through different ways to delete those pesky first three rows and get your dataframe neat and clean. Let's dive in!
The Problem 🤔
You have a dataframe in Pandas, and you want to remove the first three rows. You might have already tried using df.ix[:-1]
to remove the last row, but it doesn't work for removing the first three rows. So how can we achieve this?
Solution 1: Using DataFrame Slicing 🗡️
The simplest way to delete the first three rows is by using dataframe slicing. With Pandas, slicing allows us to select a specific portion of a dataframe. In this case, we want to exclude the first three rows.
Here's the code:
df = df.iloc[3:]
This code uses the iloc
function to access rows by their integer positions. By specifying [3:]
, we are selecting all rows starting from the fourth row onwards. This effectively removes the first three rows from the dataframe.
Solution 2: Resetting the Index 🔢
Another way to remove the first three rows is by resetting the index of the dataframe. This can be helpful if you want to keep the original order of the rows intact.
Here's the code:
df = df.iloc[3:].reset_index(drop=True)
In this code, we're using the same slicing technique as before to exclude the first three rows. However, we also include reset_index(drop=True)
to reset the index of the dataframe. The drop=True
parameter ensures that the original index values are not included in the new index.
Solution 3: Using a Boolean Mask 😷
If you have a specific condition to identify the rows you want to remove, you can use a boolean mask. This allows you to delete rows based on a certain criterion.
Here's an example:
df = df[~(df.index < 3)]
In this code, we're using the ~
operator to negate the condition (df.index < 3)
. This means we're selecting all rows where the index is not less than 3. This effectively removes the first three rows.
Conclusion and Next Steps 🏁
Congratulations! You now know three different ways to delete the first three rows of a Pandas dataframe. 💪
To recap:
Use dataframe slicing with
df.iloc[3:]
to exclude the first three rows.Reset the index using
df.iloc[3:].reset_index(drop=True)
to keep the original order of the rows.Use a boolean mask like
df[~(df.index < 3)]
to delete rows based on a specific condition.
Experiment with these methods and choose the one that best fits your needs. If you have any other questions or need further assistance, don't hesitate to reach out! 💬
Now it's your turn! Have you ever encountered any challenges when manipulating dataframes in Pandas? Share your experience and any additional tips you might have in the comments section below. Let's discuss and learn from each other! 🗣️❤️
Stay tuned for more exciting tutorials and tips on our blog. Don't forget to hit the "Subscribe" button to receive updates straight to your inbox. Happy coding! 😊👩💻👨💻