Delete a column from a Pandas DataFrame
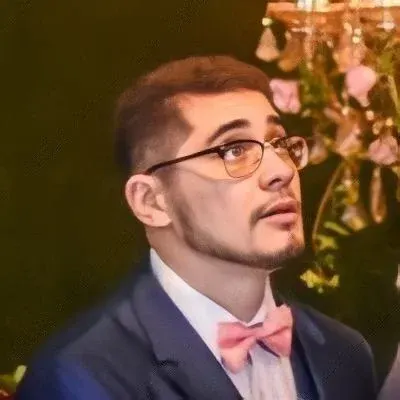
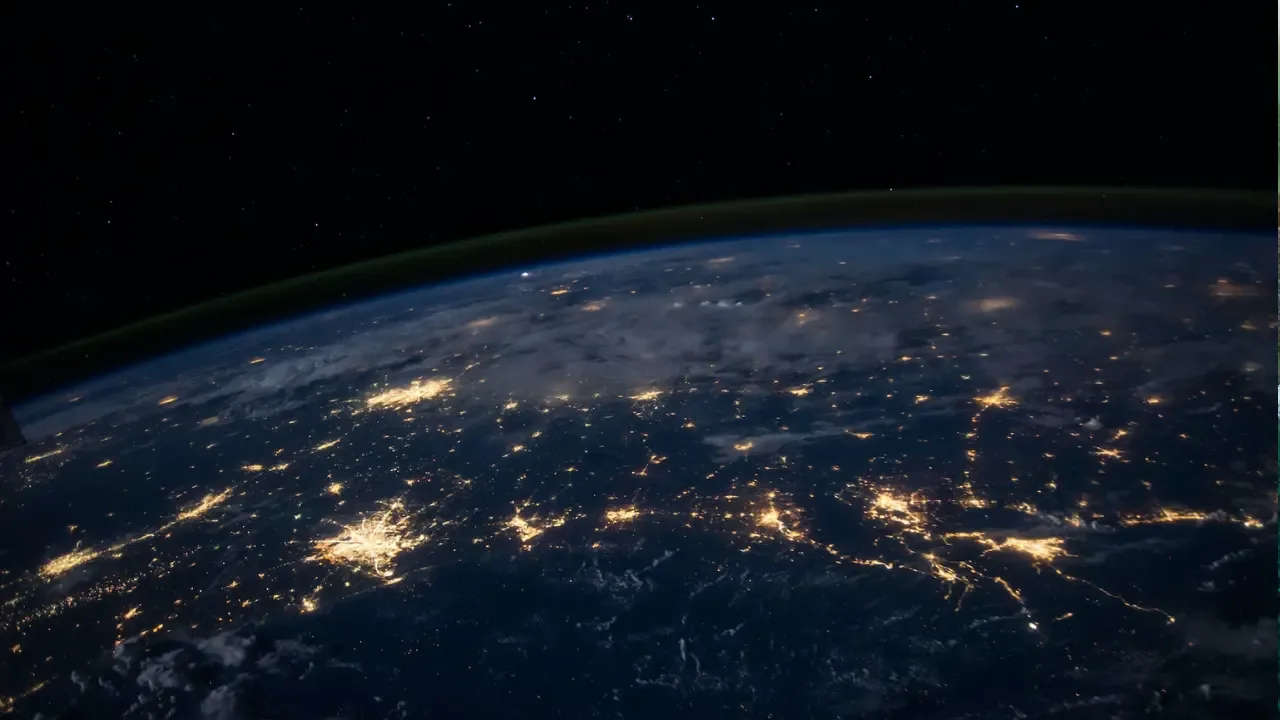
How to Delete a Column from a Pandas DataFrame 🗑️
Deleting a column from a Pandas DataFrame is a common operation when working with data analysis or preprocessing tasks. While the official Pandas documentation suggests using del df['column_name']
to delete a column, you might wonder why del df.column_name
doesn't work. In this article, we'll explore this issue and provide easy solutions to delete a column from a Pandas DataFrame.
The Problem 🤔
Let's consider the example mentioned earlier:
del df['column_name']
Using this syntax works perfectly fine, and it deletes the specified column from the DataFrame. However, when you try to delete a column using del df.column_name
, you might encounter an error. But why?
The Explanation 📚
The reason you cannot delete a column using del df.column_name
is that this syntax is primarily used for attribute access. In Pandas, when you load a DataFrame, the column names become attributes of the DataFrame itself. Hence, you can access a column using both dictionary-like notation (df['column_name']
) and attribute notation (df.column_name
).
However, when it comes to deleting a column, the attribute notation is not supported. Instead, you need to use the dictionary-like notation with the del
keyword to remove a column from the DataFrame.
Easy Solutions 💡
Now that we understand why del df.column_name
doesn't work, let's explore some easy ways to delete a column from a Pandas DataFrame.
1. Using del
Keyword
To delete a column, you can simply use the del
keyword followed by the column name enclosed in square brackets, like this:
del df['column_name']
This approach is simple, intuitive, and recommended by the Pandas documentation.
2. Using .pop()
Method
Another way to delete a column is by using the .pop()
method. This method removes a column from the DataFrame and returns it. Here's an example:
column = df.pop('column_name')
By assigning the popped column to a variable, you can perform any necessary operations on it before discarding it.
3. Using .drop()
Method
The .drop()
method is useful when you want to remove multiple columns at once. You can pass a list of column names to the columns
parameter to delete them from the DataFrame. Here's how:
df.drop(columns=['column_name_1', 'column_name_2'], inplace=True)
The inplace=True
argument ensures that the changes are made directly to the DataFrame.
Conclusion and Call-to-Action 🏁
In conclusion, to delete a column from a Pandas DataFrame, you should use del df['column_name']
instead of del df.column_name
. This ensures that you're using the correct syntax and following the recommended approach.
We hope this guide has helped you understand the issue and provided easy solutions to delete a column from a Pandas DataFrame. If you found this article helpful, feel free to share it with your friends or colleagues who might also benefit from it.
Now it's your turn to practice! Try deleting a column from a Pandas DataFrame using one of the methods discussed here. If you face any challenges or have any additional questions, please let us know in the comments section below. Happy coding! 😄🐼
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
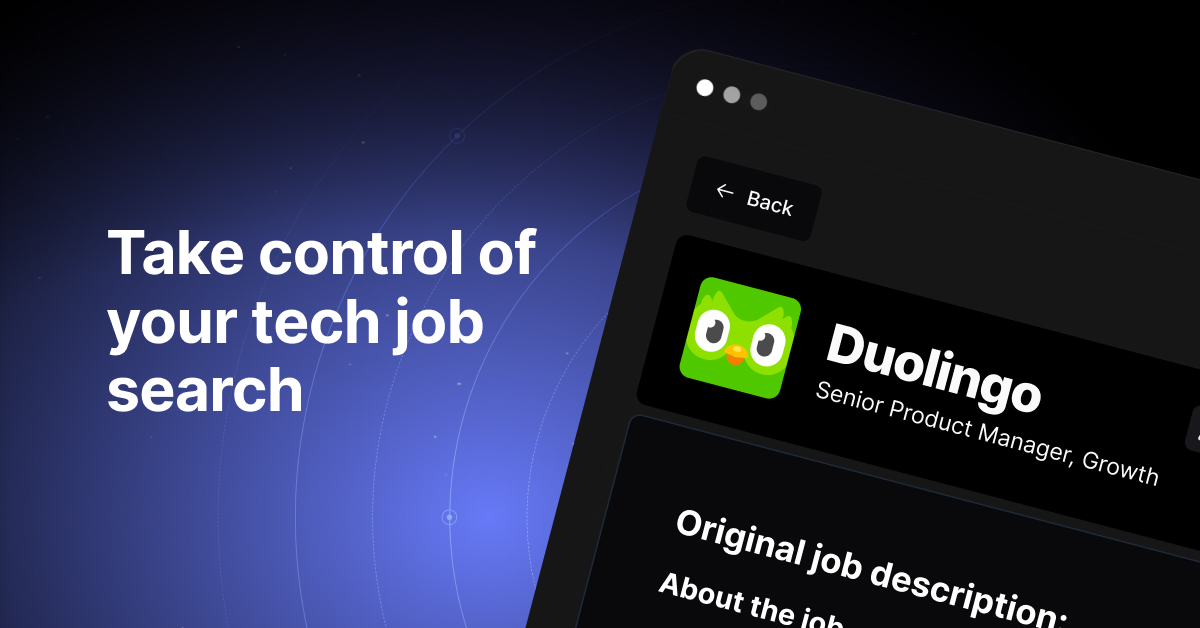