Deep copy of a dict in python
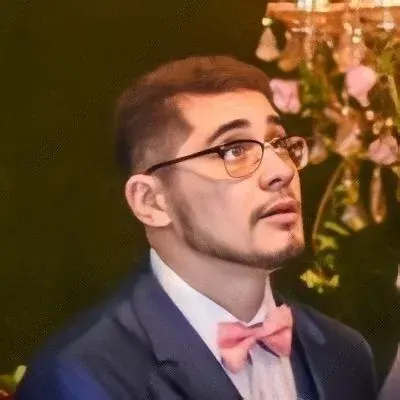
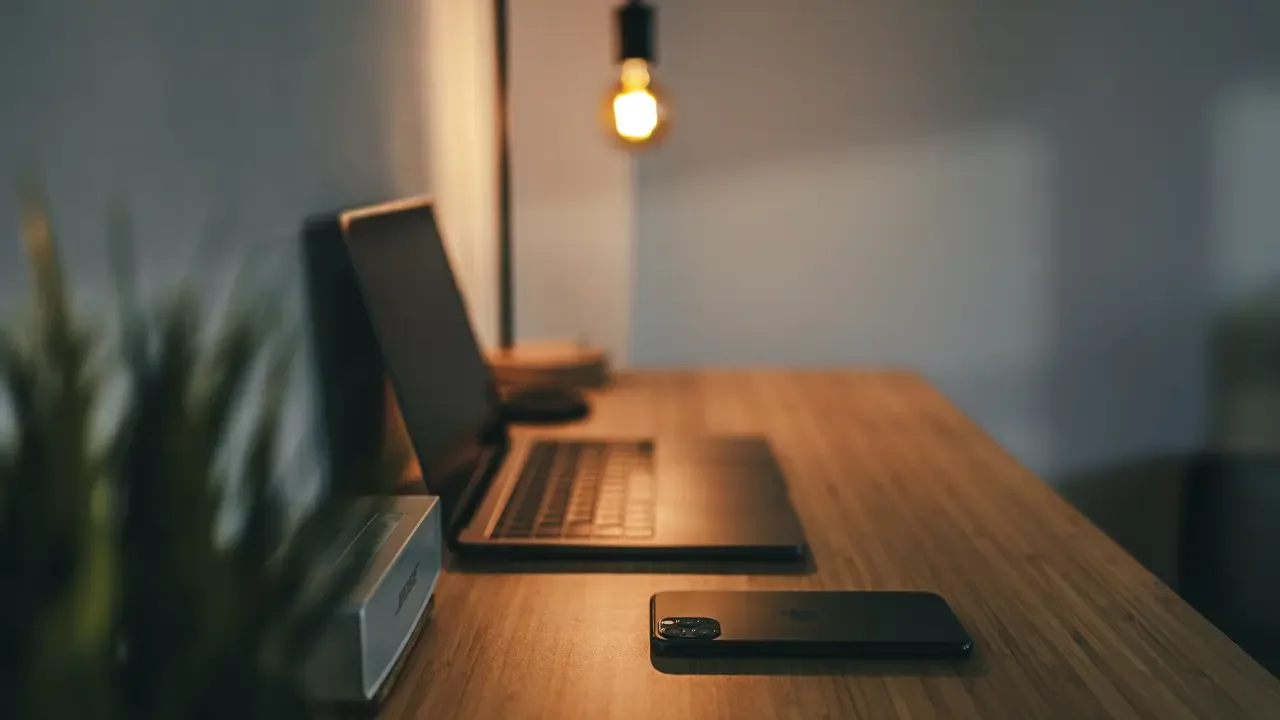
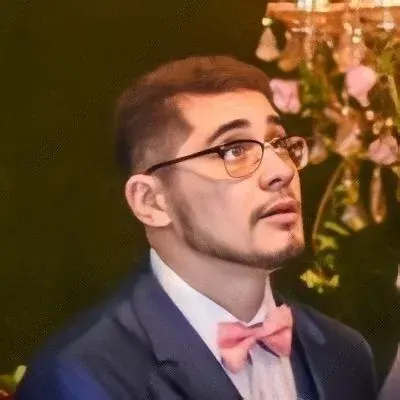
The Deep Copy Dilemma: Cloning a Dictionary in Python
š Have you ever found yourself in a situation where you needed to create a deep copy of a dictionary in Python? š¤ You might be scratching your head, wondering why the built-in .deepcopy()
method doesn't work for dictionaries. Well, fear not! In this blog post, we will explore the common issues surrounding deep copying dictionaries, provide you with easy solutions, and leave you with a compelling call-to-action. Let's dive in! š”
The Problem š®
So, you have a dictionary called my_dict
and you want to create a completely independent copy of it. You've read about the handy .deepcopy()
method, but it seems that dictionaries in Python don't have this magical ability. š
>>> my_dict = {'a': [1, 2, 3], 'b': [4, 5, 6]}
>>> my_copy = my_dict.deepcopy()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'dict' object has no attribute 'deepcopy'
The above code throws an AttributeError
because dictionaries in Python do not have a built-in .deepcopy()
method like other objects. This means that a simple .copy()
won't give you the desired deep copy behavior.
The Solution š”
To resolve this issue, we can take advantage of the copy
module provided by Python's standard library. This module contains a function called deepcopy()
that can be used to perform deep copies of objects, including dictionaries.
>>> import copy
>>> my_dict = {'a': [1, 2, 3], 'b': [4, 5, 6]}
>>> my_copy = copy.deepcopy(my_dict)
By utilizing copy.deepcopy()
, we can create an independent copy of my_dict
that won't be affected by subsequent modifications to the original dictionary.
>>> my_dict['a'][2] = 7
>>> my_copy['a'][2]
3
With the deep copy, the last line correctly returns 3
, as the modification to my_dict
did not impact the snapshot held by my_copy
. š
Compatibility with Python 3.x āļø
The solution provided using the copy
module is fully compatible with Python 3.x versions. You can safely use it regardless of the specific version you are working with.
Wrap-Up and Call-to-Action āØ
Creating a deep copy of a dictionary in Python is made easy with the copy.deepcopy()
function from the copy
module. By using this solution, you can ensure that changes made to the original dictionary do not affect its cloned counterpart. š
Next time you find yourself needing to clone a dictionary, remember to reach out for the copy.deepcopy()
function and enjoy your independent copy! š
If you found this blog post helpful, don't hesitate to share it with your fellow Pythonistas! And if you have any further questions or insights, we would love to hear from you in the comments section below. Let's keep the Python community thriving! šš