CSV in Python adding an extra carriage return, on Windows
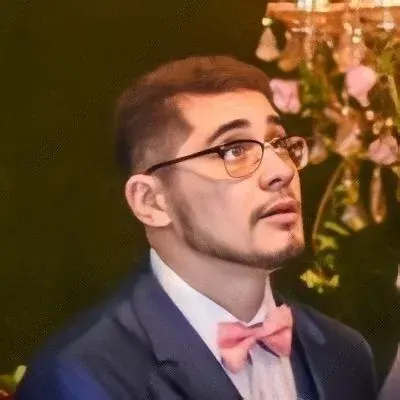
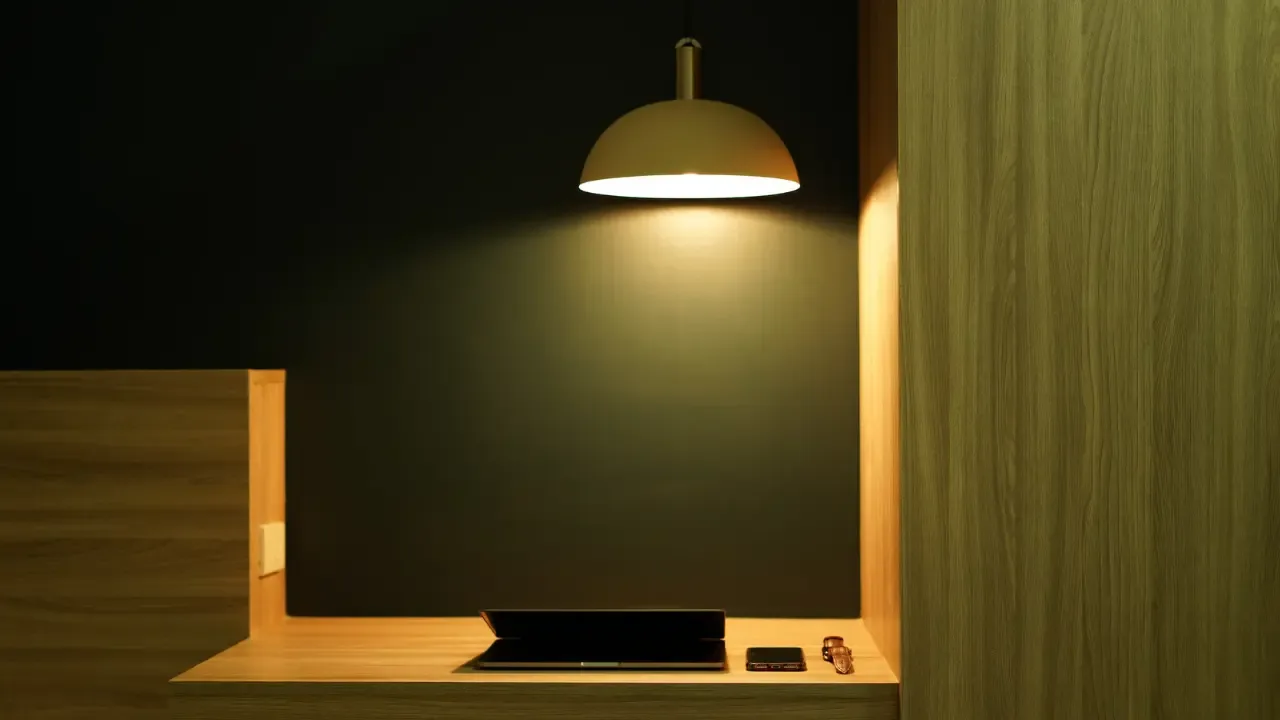
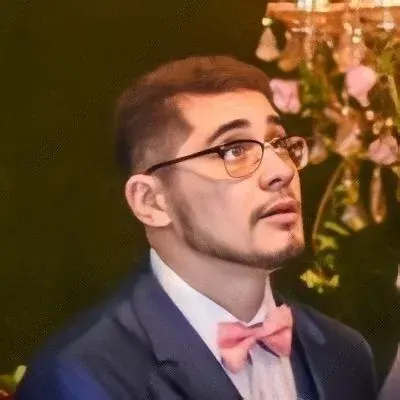
š Title: CSV in Python Adding an Extra Carriage Return on Windows: A Simple Solution!
š Hey tech enthusiasts! šØāš» Welcome back to another exciting blog post! Today, we are diving into the puzzling world of CSV handling in Python on Windows. šš»
The Problem:
So, there you are, using Python's fantastic csv
module to create a CSV file. You write down your rows, save the file, and when you open it, you notice something peculiar - an extra \r
at the end of each row! š±
import csv
with open('test.csv', 'w') as outfile:
writer = csv.writer(outfile, delimiter=',', quoting=csv.QUOTE_MINIMAL)
writer.writerow(['hi', 'dude'])
writer.writerow(['hi2', 'dude2'])
The generated file, test.csv
, looks like this:
hi,dude\r\r\nhi2,dude2\r\r\n
But, you expected it to look like this:
hi,dude\r\nhi2,dude2\r\n
What's causing this confusion? Is it a bug? Stick around as we unravel this mystery! š
Understanding the issue:
To comprehend what's happening, we need to delve into the depths of Windows and Python. On Windows, the end-of-line character consists of two characters: \r\n
(carriage return + line feed). However, Python's csv
module is designed to work with the Unix-style newline character, \n
, commonly used on non-Windows platforms.
So, the extra \r
in each row is essentially Windows saying "Hey, I'm adding a carriage return before the newline character because that's how we roll here!" š
The Solution:
Fear not, fellow developers! There are a couple of simple ways we can resolve this issue and obtain the desired output.
Solution 1: Using newline=''
By modifying the open
function's newline
parameter to an empty string ''
, we let Python handle line endings transparently. This way, Python handles the correct line endings for the underlying OS, eliminating the extra \r
.
š” Here's an updated snippet:
import csv
with open('test.csv', 'w', newline='') as outfile:
writer = csv.writer(outfile, delimiter=',', quoting=csv.QUOTE_MINIMAL)
writer.writerow(['hi', 'dude'])
writer.writerow(['hi2', 'dude2'])
Running this code will now generate the expected output:
hi,dude\r\nhi2,dude\r\n
Boom! Problem solved! š
Solution 2: Using io
module
Another approach involves using the io
module to create a universal newline writer. By wrapping the outfile
using io.TextIOWrapper
, we gain the advantage of transparently handling line endings according to the platform.
š Let's take a look at the updated code:
import csv
import io
with open('test.csv', 'w') as outfile:
writer = csv.writer(io.TextIOWrapper(outfile, newline=''), delimiter=',', quoting=csv.QUOTE_MINIMAL)
writer.writerow(['hi', 'dude'])
writer.writerow(['hi2', 'dude2'])
Executing this code results in the expected output:
hi,dude\r\nhi2,dude\r\n
Yippee! Another problem conquered! š
A Call to Action:
Hooray! You made it to the end! š We hope this guide helped you understand and resolve the mysterious extra carriage return issue when working with CSV files in Python on Windows.
Do you have any other Python-related conundrums you'd like us to untangle? Let us know in the comments below! ššÆļø
Happy Python coding! š»āØ