CSV file written with Python has blank lines between each row
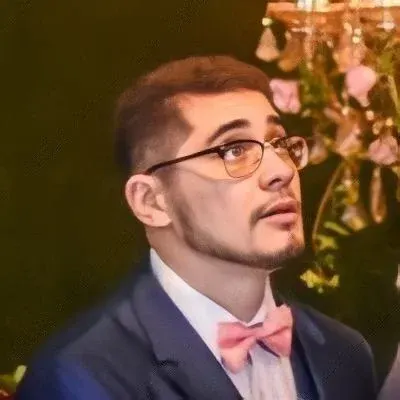
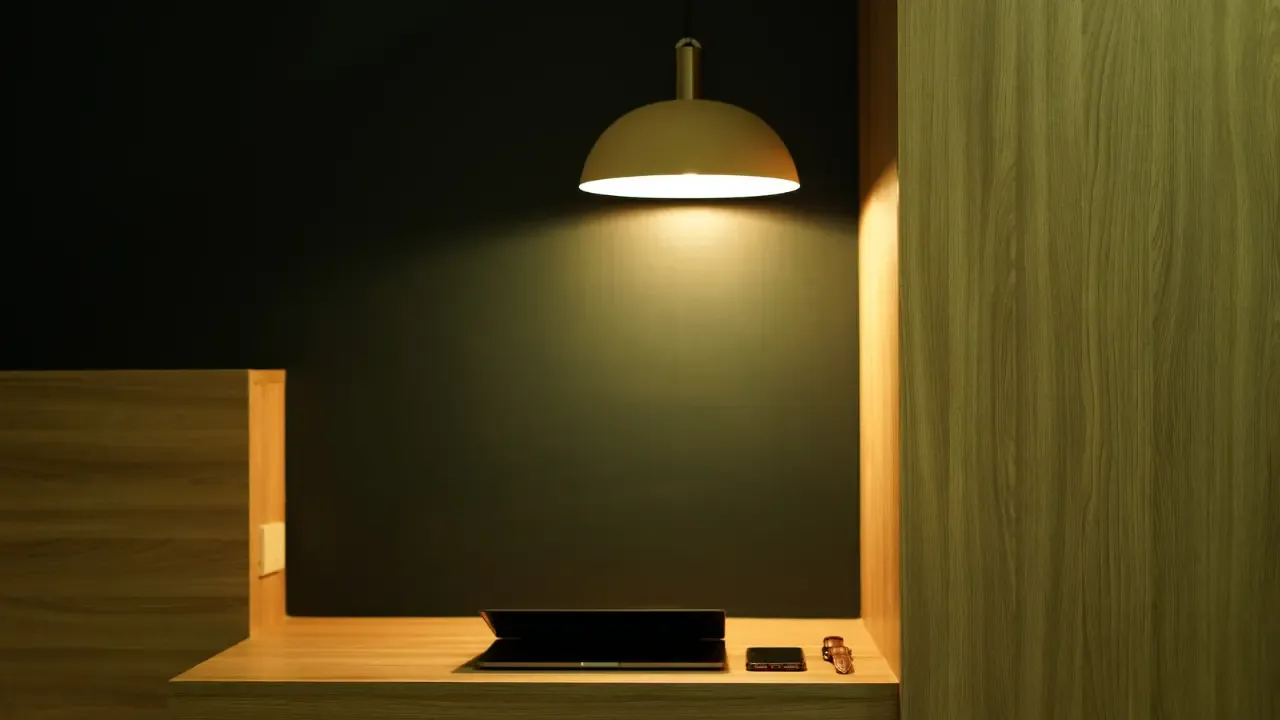
📃 Hey there tech enthusiasts! 👋 Are you faced with the frustrating issue of having blank lines between each row in a CSV file written with Python? 📁 Don't worry, you're not alone! This is a common problem that many developers encounter when working with CSV files.
Let's dive in and explore a simple solution to get rid of those pesky blank lines and ensure your CSV file is clean and tidy. 💪
The code snippet you provided seems to be a Python script that reads a CSV file, performs some operations on it, and writes the modified data to a new CSV file. However, when opening the resulting file in Microsoft Excel, you notice the presence of those annoying blank lines.
To address this issue, we need to modify the code slightly. Let's take a closer look at the solution:
import csv
with open('thefile.csv', 'r', newline='') as infile, open('thefile_subset1.csv', 'w', newline='') as outfile:
reader = csv.reader(infile)
writer = csv.writer(outfile)
for row in reader:
writer.writerow(row)
Instead of using the list(csv.reader(f))
approach, we utilize the csv.reader
iterator directly. Here are the changes we made:
The file mode for opening the input file is modified to
'r'
instead of'rb'
. This ensures that the file is opened in text mode, which is more compatible with CSV files.We pass
newline=''
as an argument to bothopen()
functions. This is essential as it ensures that no extra blank lines are added when writing the data to the output file.We use
csv.reader(infile)
to read the input file directly within a loop, row by row.Finally, we write each row to the output file using
writer.writerow(row)
.
By making these changes, the resulting CSV file should no longer have those annoying blank lines. 🙌
To give you a better understanding, here's an example to illustrate the solution. Suppose we have the following input CSV file:
Name, Age, Email
John Doe, 25, john@example.com
Jane Smith, 30, jane@example.com
After running the modified code, the output CSV file will look like this:
Name, Age, Email
John Doe, 25, john@example.com
Jane Smith, 30, jane@example.com
Great! Now your CSV file should be clean and ready to use without any unwanted blank lines.
Remember, it's essential to stay up-to-date with best practices when working with CSV files to ensure smooth data processing.
If you found this solution helpful, don't forget to share it with your fellow developers! Sharing is caring, after all. 😉 And if you have any other tech-related questions or issues, feel free to reach out and let us know. We're here to help!
Keep coding and happy tech-ing! ✨🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
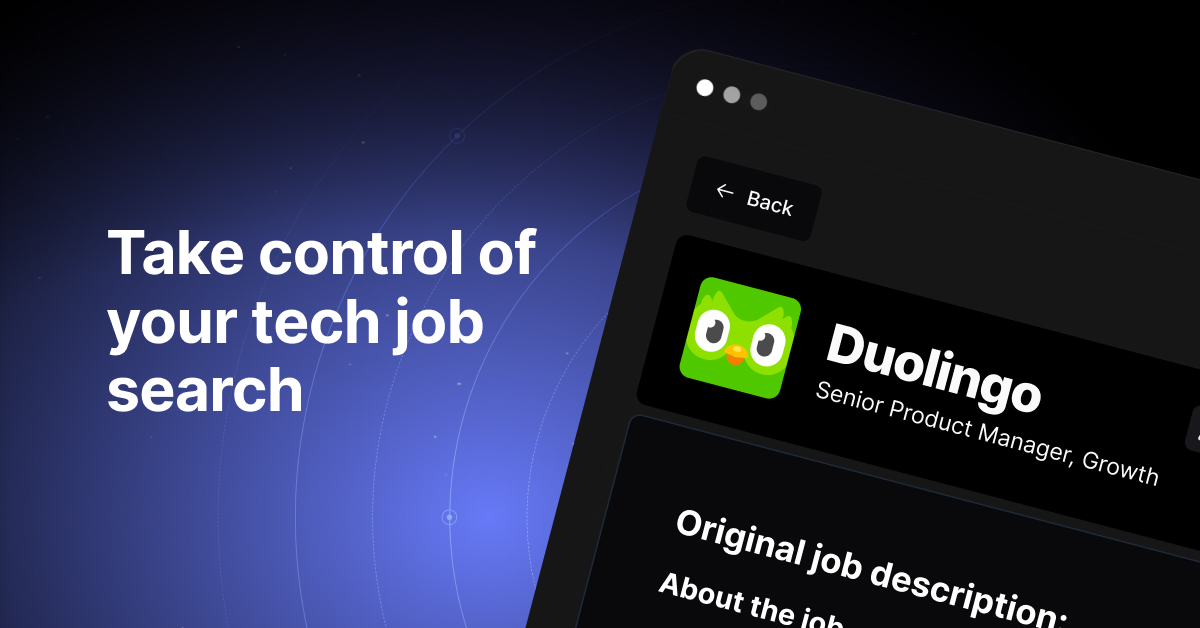