csv.Error: iterator should return strings, not bytes
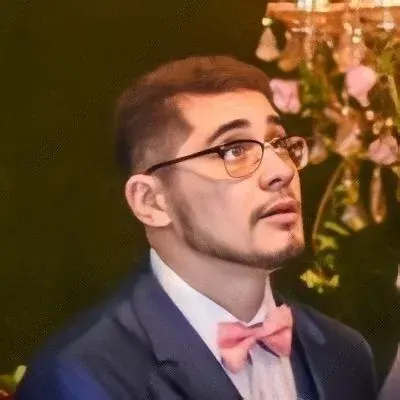
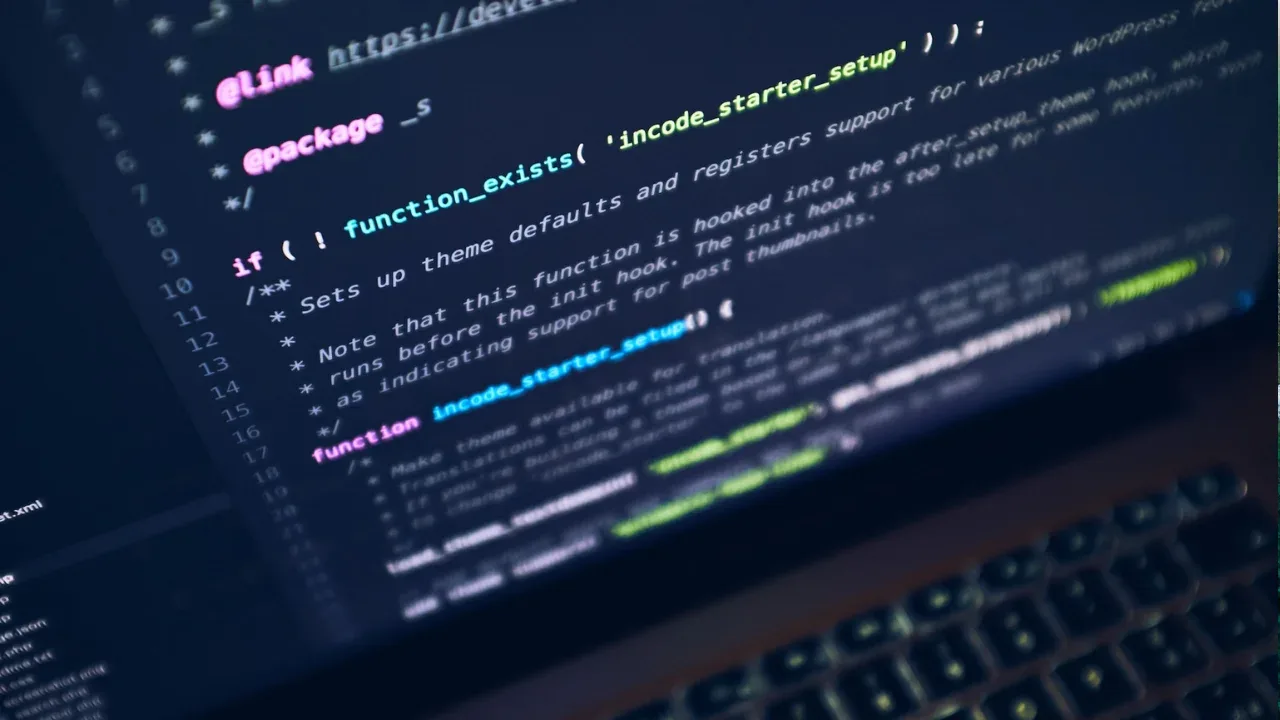
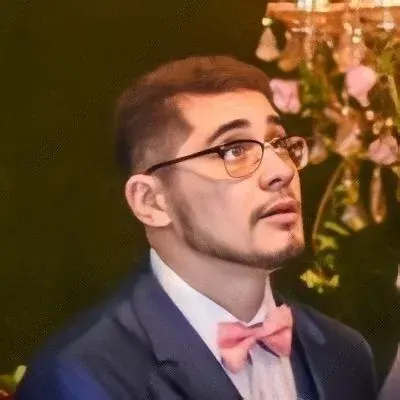
Solving the csv.Error: iterator should return strings, not bytes
So you're working with CSV files in Python and you encounter the dreaded csv.Error: iterator should return strings, not bytes
error. 😱 Don't worry, I've got your back! In this blog post, I'll explain what this error means, why it occurs, and provide you with easy solutions to fix it. Let's dive in! 💪
Understanding the Error
First, let's understand why this error occurs in the first place. When you open a file in Python, you have the option to open it in binary mode ("rb"
) or text mode ("r"
or "rt"
). The csv.reader
function expects the file to be opened in text mode, where the data is treated as strings.
In your code snippet, you opened the CSV file in binary mode using "rb"
. This causes the csv.reader
to interpret each row as bytes instead of strings, leading to the error.
Solution 1: Open the File in Text Mode
The simplest solution is to open the CSV file in text mode ("r"
) instead of binary mode ("rb"
). Update your code as follows:
import csv
with open('sample.csv', "r") as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
By opening the file in text mode, you ensure that the csv.reader
will correctly interpret the data as strings, preventing the error from occurring.
Solution 2: Decode the Bytes
If for some reason you need to open the file in binary mode, you can decode the bytes into strings using the appropriate encoding. Let's say your file is encoded in UTF-8, you can modify your code like this:
import csv
with open('sample.csv', "rb") as csvfile:
reader = csv.reader((line.decode('utf-8') for line in csvfile))
for row in reader:
print(row)
Here, we use a generator expression to decode each line from bytes to strings before passing it to the csv.reader
. This ensures that the data is correctly interpreted as strings, avoiding the error.
Solution 3: Use io.TextIOWrapper
Another alternative is to use Python's io.TextIOWrapper
to wrap your binary file object with a text-mode interface. Here's how you can modify your code:
import csv
import io
with open('sample.csv', "rb") as csvfile:
wrapper = io.TextIOWrapper(csvfile, encoding='utf-8')
reader = csv.reader(wrapper)
for row in reader:
print(row)
The io.TextIOWrapper
handles the decoding for you, providing the csv.reader
with the necessary text-mode interface.
Conclusion
In this guide, we discussed the csv.Error: iterator should return strings, not bytes
error and provided you with three easy solutions to fix it. Whether you choose to open the file in text mode, decode the bytes, or use io.TextIOWrapper
, you can now tackle this error with confidence. Happy coding! 🎉
I hope you found this guide helpful! If you have any questions or other tricky tech problems you'd like me to cover, feel free to leave a comment below. 👇