Creating an empty Pandas DataFrame, and then filling it
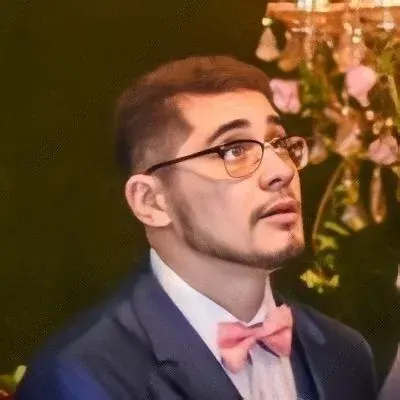
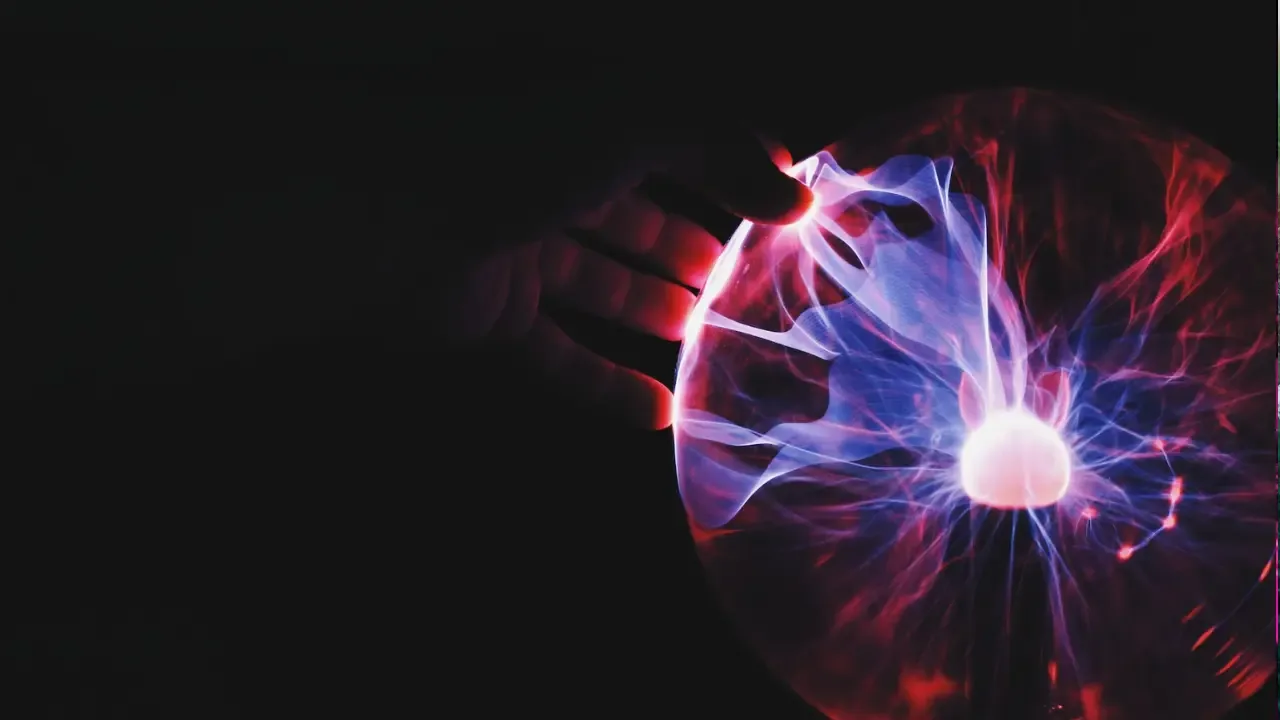
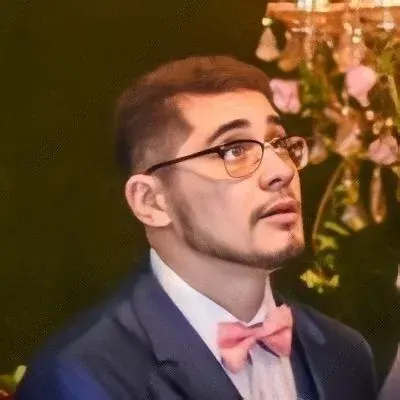
Creating an Empty Pandas DataFrame and Filling it: A Step-by-Step Guide
Introduction
Pandas is an open-source data analysis and manipulation library for Python. It provides a powerful toolset for working with structured data, including the ability to create and manipulate DataFrames.
The Problem
A common task when working with Pandas is to create an empty DataFrame and then fill it with values. This can be useful when you want to perform calculations or analysis on a time series dataset. However, the process of filling an empty DataFrame can sometimes be challenging or inefficient, especially if you're not familiar with the available tools and techniques.
In this blog post, we will address the problem of creating an empty Pandas DataFrame and efficiently filling it with values.
Step 1: Importing the Required Libraries
To get started, we need to import the necessary libraries. In this case, we will import pandas
, datetime
, and scipy
.
import pandas as pd
import datetime as dt
import scipy as s
Step 2: Initializing the DataFrame
Next, let's initialize the empty DataFrame with the desired columns and timestamp rows.
base = dt.datetime.today().date()
dates = [ base - dt.timedelta(days=x) for x in range(9, -1, -1) ]
valdict = {}
symbols = ['A','B', 'C']
for symb in symbols:
valdict[symb] = pd.Series(s.zeros(len(dates)), dates)
In the above code, we first create a base date using datetime.today().date()
. Then, we generate a list of dates by subtracting a certain number of days from the base date using a list comprehension.
We also create an empty dictionary valdict
to hold the columns of our DataFrame. Next, we iterate over the symbols
list and create a Pandas Series
with zeros for each symbol, using the dates as the index. These series are then added to the valdict
dictionary.
At this point, we have an empty DataFrame with columns 'A', 'B', 'C' and rows corresponding to the provided dates, all initialized with zeros.
Step 3: Filling the DataFrame
Finally, let's fill the DataFrame with values using a time series calculation. In this example, we will increment each value in a column by 1 compared to the previous day.
for thedate in dates:
if thedate > dates[0]:
for symb in valdict:
valdict[symb][thedate] = 1 + valdict[symb][thedate - dt.timedelta(days=1)]
In the above code, we iterate over the dates starting from the second date. For each date, we iterate over the symbols in valdict
and calculate the new value by adding 1 to the corresponding value from the previous day. The calculated values are then assigned to the DataFrame using the valdict[symb][thedate]
syntax.
With this approach, we efficiently iterate over the DataFrame and fill it with values using the previous day's data.
Conclusion
Creating an empty Pandas DataFrame and filling it with values can be a common and essential task in data analysis. By following the steps outlined in this guide, you can efficiently initialize an empty DataFrame and populate it with the desired values using time series calculations.
Now that you have the knowledge and tools to tackle this problem, go ahead and experiment with your own datasets. Feel free to share your experiences, ideas, or any additional questions in the comments below. Happy coding! 💻📊
*[Pandas]: Python library for data analysis and manipulation.