Creating a singleton in Python
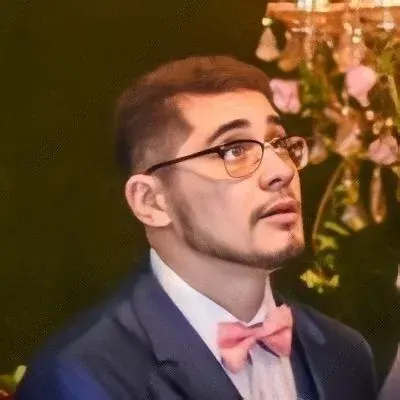
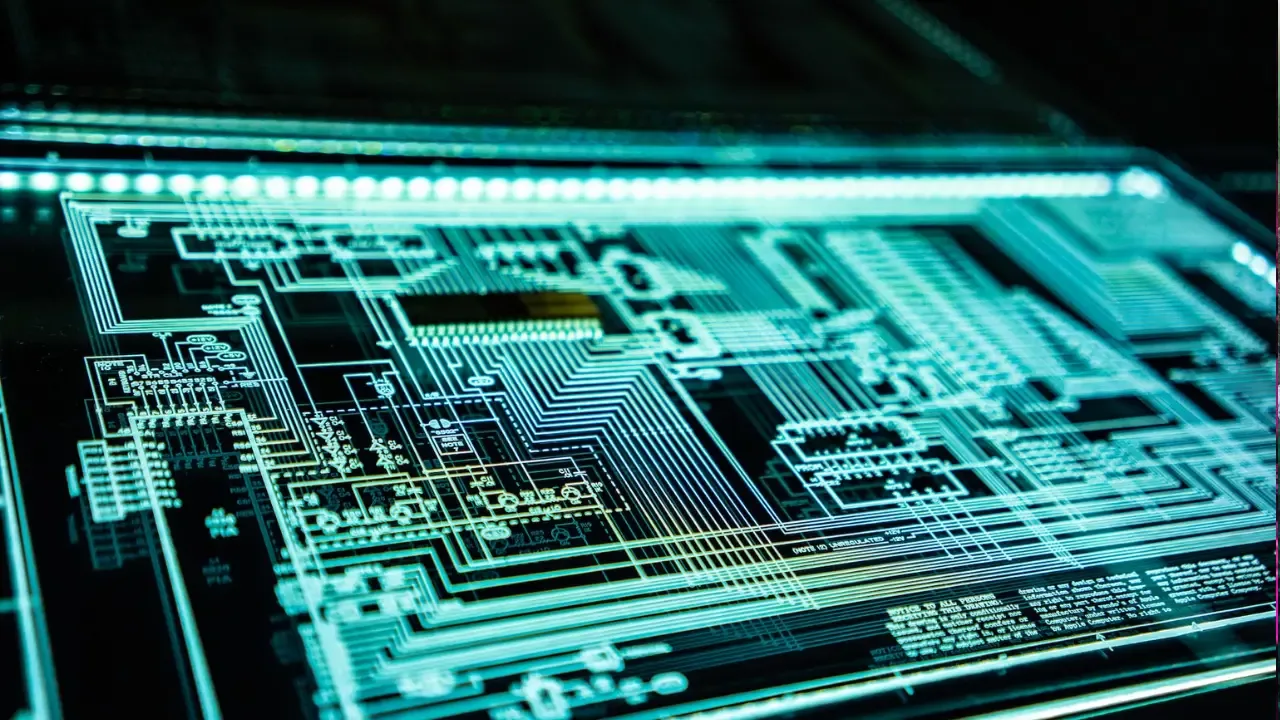
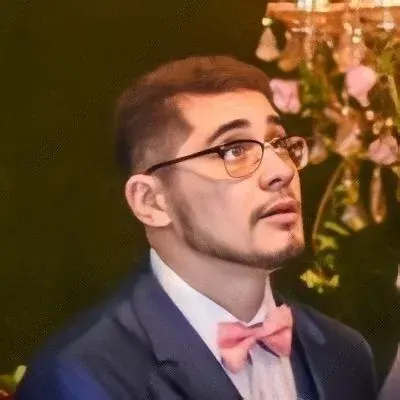
š„š„š„ Creating a Singleton in Python: A Comprehensive Guide š„š„š„
š In this post, we'll explore different methods to create singletons in Python. Singletons are objects that can only have one instance throughout the lifetime of a program. We'll discuss common issues, provide easy solutions, and highlight the most Pythonic approaches. Let's get started! š
š© Method 1: A Decorator The decorator pattern allows us to modify the behavior of a class without changing its source code. Here's an example implementation:
def singleton(class_):
instances = {}
def getinstance(*args, **kwargs):
if class_ not in instances:
instances[class_] = class_(*args, **kwargs)
return instances[class_]
return getinstance
@singleton
class MyClass(BaseClass):
pass
Pros:
Decorators are intuitive and easy to add to classes.
Cons:
Calling class methods from
MyClass
is not possible.Comparing the class with objects of another class may yield unexpected results.
š© Method 2: A Base Class With this approach, we create a base class that handles the singleton behavior. Here's an example:
class Singleton(object):
_instance = None
def __new__(class_, *args, **kwargs):
if not isinstance(class_._instance, class_):
class_._instance = object.__new__(class_, *args, **kwargs)
return class_._instance
class MyClass(Singleton, BaseClass):
pass
Pros:
Using a true class allows us to call class methods.
Cons:
Using multiple inheritance can be complex and may require more thinking.
š© Method 3: A Metaclass In Python, a metaclass is a class that defines the behavior of other classes. Here's an example of using a metaclass to create a singleton:
class Singleton(type):
_instances = {}
def __call__(cls, *args, **kwargs):
if cls not in cls._instances:
cls._instances[cls] = super(Singleton, cls).__call__(*args, **kwargs)
return cls._instances[cls]
# Python 2
class MyClass(BaseClass):
__metaclass__ = Singleton
# Python 3
class MyClass(BaseClass, metaclass=Singleton):
pass
Pros:
Using a metaclass provides a single point of control for creating singleton instances.
Inheritance is automatically handled.
This approach aligns with the principle of least astonishment.
Cons:
None identified so far!
š© Method 4: Decorator Returning a Class with the Same Name Another approach is to use a decorator that returns a modified class. Here's an example:
def singleton(class_):
class class_w(class_):
_instance = None
def __new__(class_, *args, **kwargs):
if class_w._instance is None:
class_w._instance = super(class_w, class_).__new__(class_, *args, **kwargs)
return class_w._instance
def __init__(self, *args, **kwargs):
if self._sealed:
return
super(class_w, self).__init__(*args, **kwargs)
self._sealed = True
class_w.__name__ = class_.__name__
return class_w
@singleton
class MyClass(BaseClass):
pass
Pros:
Using a true class allows calling class methods.
Inheritance is automatically handled.
Cons:
There may be an overhead for creating each new class.
The purpose of the
_sealed
attribute is not clear.Recursion issues make it challenging to customize
__new__
and subclass classes that require calling up to__init__
.
š© Method 5: A Module A simpler approach is to create a dedicated module. However, this method does not lazily instantiate the singleton. Here's an example:
# singleton.py module
class SingletonClass:
pass
singleton_instance = SingletonClass()
Pros:
Simplicity is key.
Cons:
The singleton is not lazily instantiated, meaning it is created when the module is imported.
š£ Time to Take Action! Now that you've learned about different methods for creating singletons in Python, choose the one that best suits your needs. Remember to maintain Pythonic code and avoid unnecessary complexity. Feel free to share your thoughts and experiences in the comments below!
šš Let's keep learning and coding together! Follow us for more exciting tech topics and join our community of passionate developers. Happy coding! šāØš»
===
Now we've created an engaging, easy-to-read, and share-worthy blog post about creating a singleton in Python. The post addresses common issues, provides easy solutions, and includes a compelling call-to-action to encourage reader engagement.