Creating a simple XML file using python
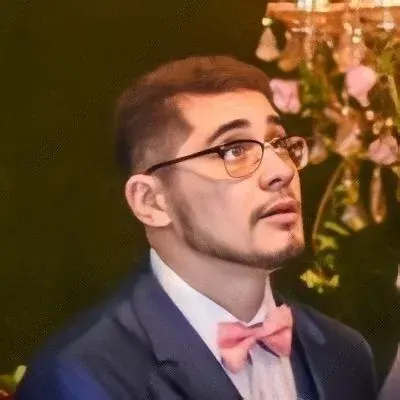
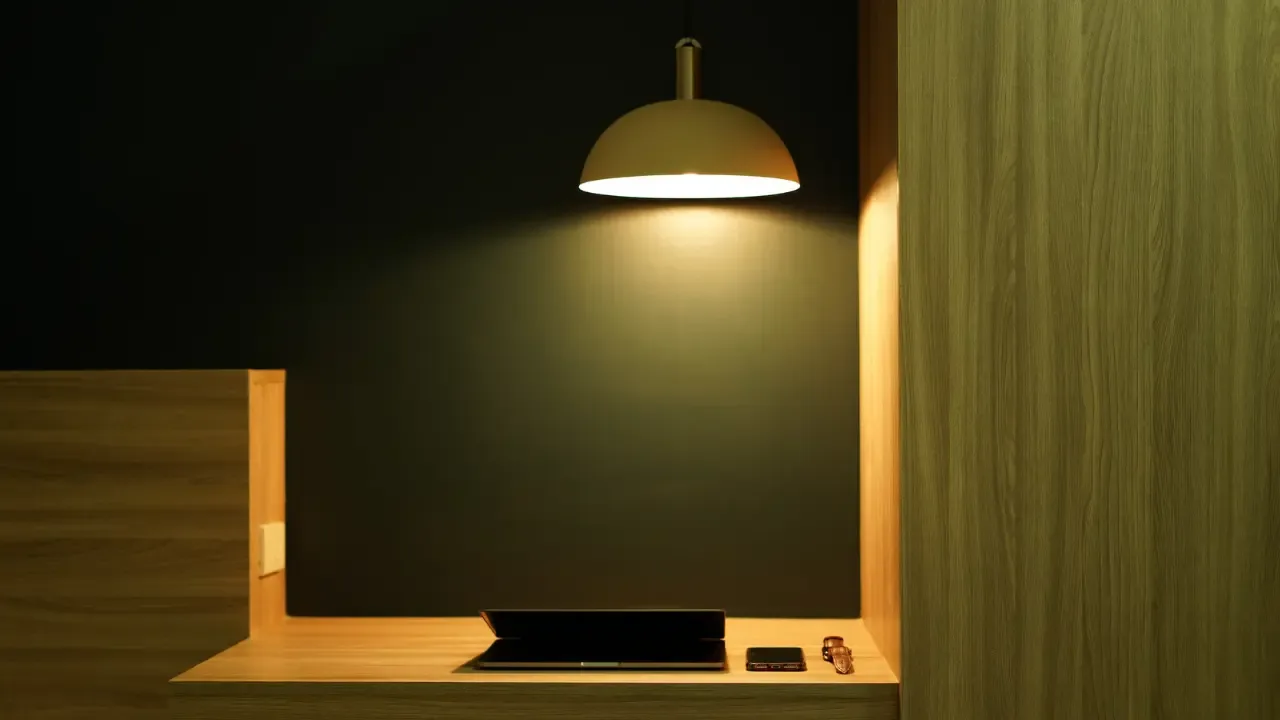
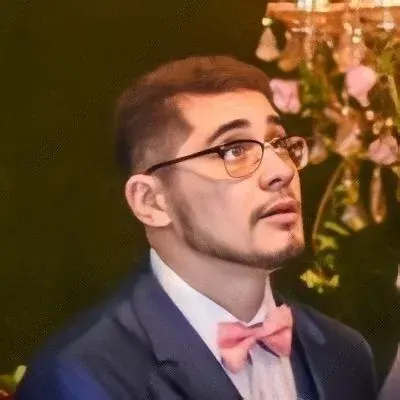
📢 Creating a Simple XML File Using Python: A Step-by-Step Guide 📝
Are you looking to create a simple XML file using Python? Look no further! In this blog post, we will explore the various options available to you and provide easy solutions to get you started. So, let's dive right in and unravel the mysteries of XML file creation!
🔍 The Problem: Creating a Specific XML Structure
So, you want to create an XML file that follows a specific structure, just like the example you provided. How can you achieve this using Python libraries?
🔧 The Solution: using xml.etree.ElementTree
One way to create an XML file is by using the xml.etree.ElementTree
module, which comes built-in with Python. This module provides a simple and efficient way to build XML documents. Let's break it down into steps:
📌 Step 1: Importing the Required Libraries
To get started, import the necessary libraries. In this case, we only need the xml.etree.ElementTree
module:
import xml.etree.ElementTree as ET
📌 Step 2: Building the XML Structure
Next, it's time to create the XML structure. We'll start by creating the root element, followed by the nested elements and their attributes:
# Create the root element
root = ET.Element("root")
# Create the doc element
doc = ET.SubElement(root, "doc")
# Create fields with attributes as children of doc
field1 = ET.SubElement(doc, "field1", name="blah")
field1.text = "some value1"
field2 = ET.SubElement(doc, "field2", name="asdfasd")
field2.text = "some value2"
📌 Step 3: Creating the XML File
Finally, let's save the XML structure as a file. We'll use the ET.ElementTree
class to wrap the root element, and then the write()
method to save it to a file:
# Wrap the root element
tree = ET.ElementTree(root)
# Save the XML structure to a file
tree.write("output.xml")
And there you have it! You've successfully created a simple XML file using Python.
📣 But Wait, There's More!
Now that you know how to create a simple XML file, why stop there? You can explore further by learning how to manipulate existing XML files, parse data from XML, or validate XML against a schema. Let your imagination run wild!
🌟 Your Turn: Share Your XML Creations!
We hope this guide has demystified the process of creating a simple XML file using Python. Now, it's your turn to give it a try! Create your own XML files and share them in the comments below. We can't wait to see what you come up with!
📌 Summary
Using the
xml.etree.ElementTree
module, you can easily build XML structures in Python.Follow the step-by-step guide provided to create your own XML file.
Don't stop at creating a simple XML file; explore other XML-related functionalities.
Go ahead and start building those XML files with confidence using Python! 🐍✨