Creating a Pandas DataFrame from a Numpy array: How do I specify the index column and column headers?
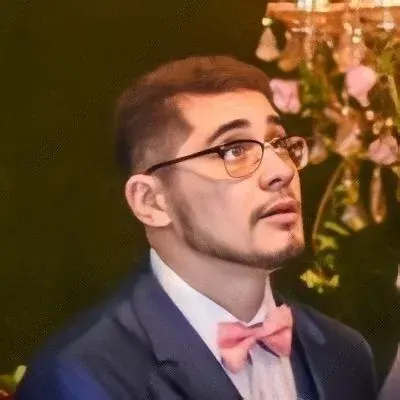
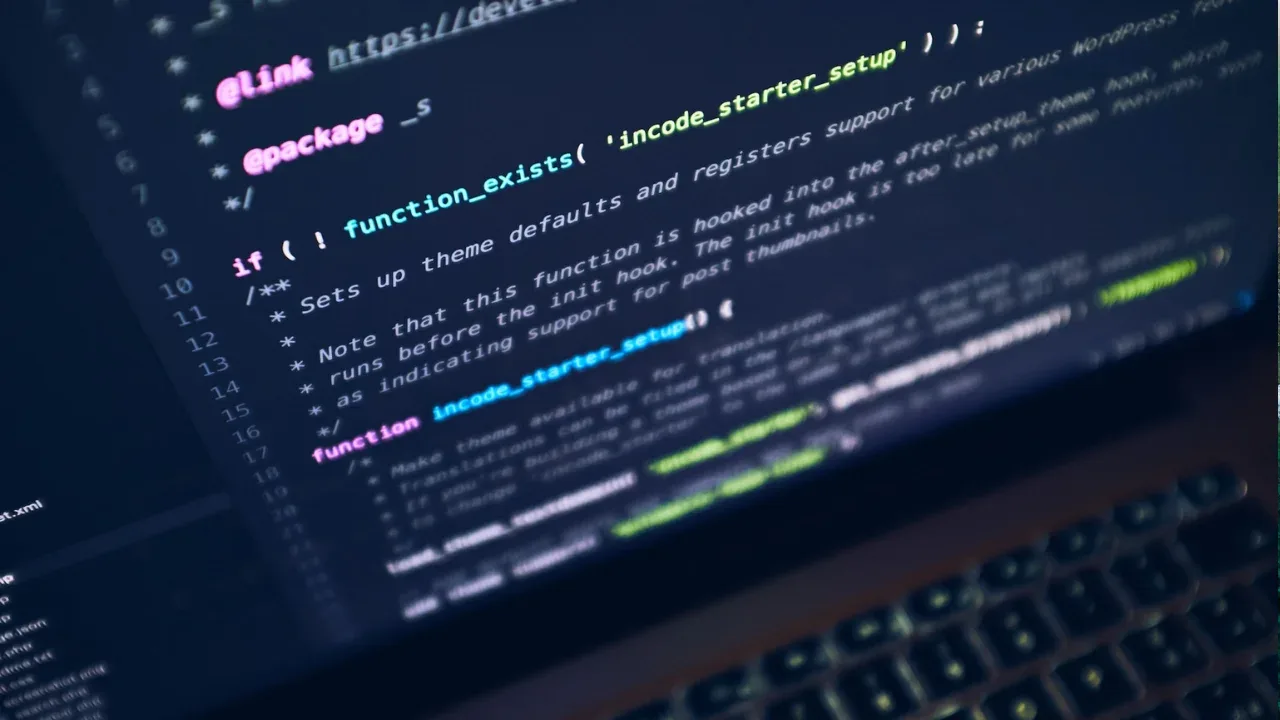
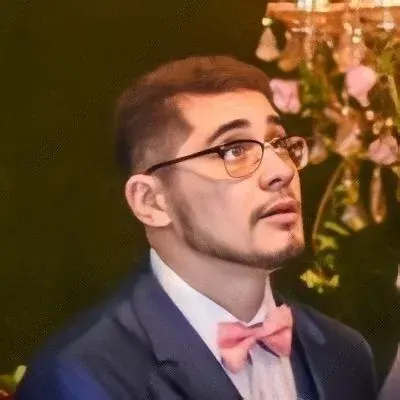
Creating a Pandas DataFrame from a Numpy array: How do I specify the index column and column headers?
Are you struggling to create a Pandas DataFrame from a Numpy array and don't know how to specify the index column and column headers? Don't worry, I've got you covered! In this blog post, I'll walk you through the process step by step and provide you with easy solutions to this common problem.
The Problem
Let's start by understanding the problem at hand. You have a Numpy array that represents a two-dimensional array with row labels and column names. Here's an example:
data = np.array([['','Col1','Col2'],['Row1',1,2],['Row2',3,4]])
You want to create a DataFrame from this array with Row1
and Row2
as index values, and Col1
and Col2
as header values. However, you're not sure how to specify the column headers.
The Solution
To create a DataFrame with the desired index column and column headers, you can use the following code:
df = pd.DataFrame(data, index=data[:,0], columns=data[0,1:])
Let's break down this code to understand how it works:
index=data[:,0]
specifies the index values for the DataFrame. In our example, it assigns the first column of the array (['Row1', 'Row2']
) as the index.columns=data[0,1:]
specifies the column headers for the DataFrame. It assigns the values starting from the second element of the first row of the array (['Col1', 'Col2']
) as the column headers.
By using the index
and columns
parameters of the pd.DataFrame
constructor, we can easily specify the index column and column headers when creating a DataFrame from a Numpy array.
An Example
Let's now apply this solution to our original example:
import numpy as np
import pandas as pd
data = np.array([['','Col1','Col2'],['Row1',1,2],['Row2',3,4]])
df = pd.DataFrame(data, index=data[:,0], columns=data[0,1:])
The resulting DataFrame will look like this:
Col1 Col2
Row1 1 2
Row2 3 4
Conclusion
Creating a Pandas DataFrame from a Numpy array with specified index column and column headers is not as complicated as it may seem. By using the index
and columns
parameters of the pd.DataFrame
constructor, you can achieve this task effortlessly.
So go ahead and give it a try! Start by creating your Numpy array, and then use the provided solution to create your desired DataFrame. And remember, if you encounter any issues or have any questions, feel free to leave a comment below. I'm here to help!
Happy coding! 😄🐼
Have you ever encountered the need to create a Pandas DataFrame from a Numpy array with specified index column and column headers? Share your experience in the comments below!