Create new column based on values from other columns / apply a function of multiple columns, row-wise in Pandas
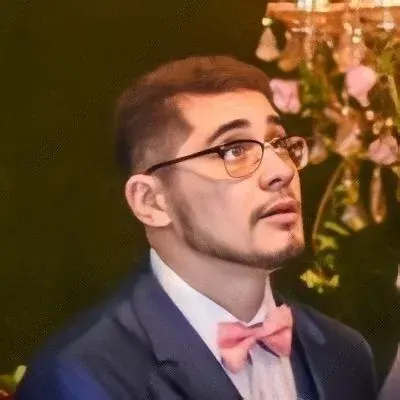
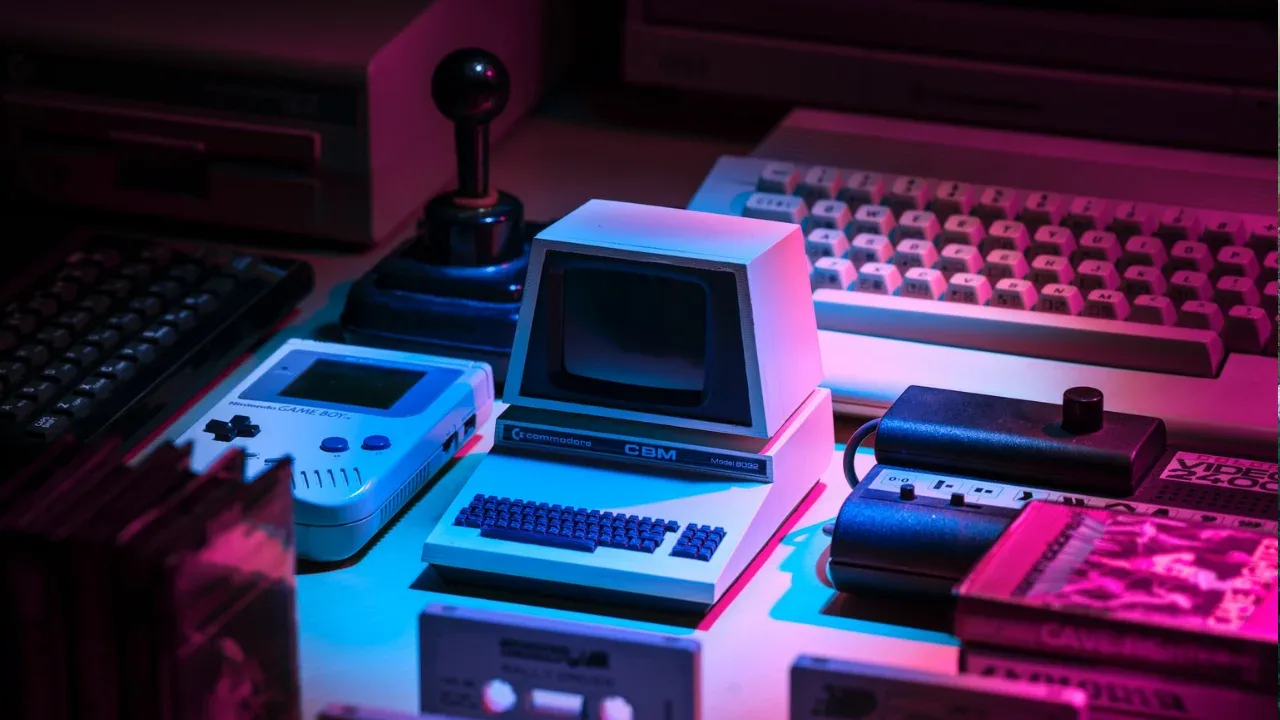
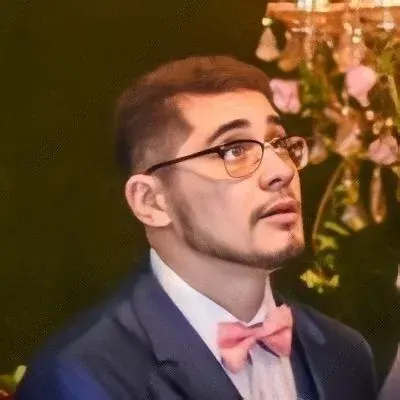
📝 Your Ultimate Guide to Creating a New Column based on Values from Other Columns in Pandas
Are you struggling to create a new column in your Pandas dataframe by applying a function to multiple columns row-wise? Look no further! In this guide, we'll address common issues and provide easy solutions to help you accomplish this task effortlessly. 💪
The Challenge: Applying a Custom Function to Multiple Columns
The context of this question revolves around applying a custom function to six columns in each row of a dataframe. The columns in question are as follows: ERI_Hispanic
, ERI_AmerInd_AKNatv
, ERI_Asian
, ERI_Black_Afr.Amer
, ERI_HI_PacIsl
, and ERI_White
. Sounds tricky, right? But fear not, we've got you covered! 😎
The Critical Criteria
Before we jump into the solutions, let's understand the critical criteria set for creating the new column. Here's a summary:
If the
ERI_Hispanic
column is equal to 1, the person should be classified as "Hispanic" (Even if they have a "1" in another ethnicity column, they are still counted as Hispanic).If the sum of all the non-Hispanic ethnicity columns (i.e.,
ERI_AmerInd_AKNatv
,ERI_Asian
,ERI_Black_Afr.Amer
,ERI_HI_PacIsl
, andERI_White
) is greater than 1, the person should be classified as "Two or More".If any of the non-Hispanic ethnicity columns is equal to 1, the person should be classified accordingly: "A/I AK Native" for
ERI_AmerInd_AKNatv
, "Asian" forERI_Asian
, "Black/AA" forERI_Black_Afr.Amer
, "Haw/Pac Isl." forERI_HI_PacIsl
, and "White" forERI_White
.
Let's Dive into Solutions!
Now that we understand the criteria, let's explore some solutions:
Solution 1: Using Pandas' apply
Function
One way to tackle this problem is by using the apply
function along with a lambda function. Here's an example code snippet that demonstrates this approach:
import pandas as pd
# Define your custom function
def classify_ethnicity(row):
if row['ERI_Hispanic'] == 1:
return 'Hispanic'
elif row[['ERI_AmerInd_AKNatv', 'ERI_Asian', 'ERI_Black_Afr.Amer', 'ERI_HI_PacIsl', 'ERI_White']].sum() > 1:
return 'Two or More'
elif row['ERI_AmerInd_AKNatv'] == 1:
return 'A/I AK Native'
elif row['ERI_Asian'] == 1:
return 'Asian'
elif row['ERI_Black_Afr.Amer'] == 1:
return 'Black/AA'
elif row['ERI_HI_PacIsl'] == 1:
return 'Haw/Pac Isl.'
elif row['ERI_White'] == 1:
return 'White'
# Apply the custom function row-wise to create the new column
df['new_column'] = df.apply(lambda row: classify_ethnicity(row), axis=1)
Solution 2: Utilizing Numpy's select
Function
For a more concise solution, you can make use of Numpy's select
function. Here's an example that demonstrates this approach:
import numpy as np
# Define the column values and conditions for each classification
column_values = ['Hispanic', 'Two or More', 'A/I AK Native', 'Asian', 'Black/AA', 'Haw/Pac Isl.', 'White']
conditions = [
(df['ERI_Hispanic'] == 1),
(df[['ERI_AmerInd_AKNatv', 'ERI_Asian', 'ERI_Black_Afr.Amer', 'ERI_HI_PacIsl', 'ERI_White']].sum(axis=1) > 1),
(df['ERI_AmerInd_AKNatv'] == 1),
(df['ERI_Asian'] == 1),
(df['ERI_Black_Afr.Amer'] == 1),
(df['ERI_HI_PacIsl'] == 1),
(df['ERI_White'] == 1)
]
# Apply the conditions and assign values using Numpy's select function
df['new_column'] = np.select(conditions, column_values, default=np.nan)
Conclusion
There you have it! We've provided two solutions to help you create a new column based on values from other columns in your Pandas dataframe. Now it's your turn to put these solutions to the test and find the one that suits your needs best. Remember, if you encounter any further issues or need clarification, feel free to leave a comment below, and we'll be more than happy to assist you! 💡
📣 Your Turn!
Have you ever faced a similar challenge while working with Pandas? How did you overcome it? Share your experience and any additional insights in the comments section below. Let's learn from each other and grow together! 🌟