Create a Pandas Dataframe by appending one row at a time
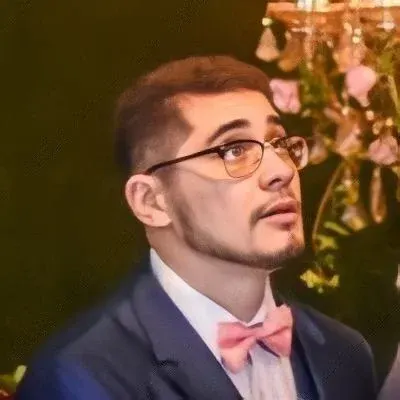
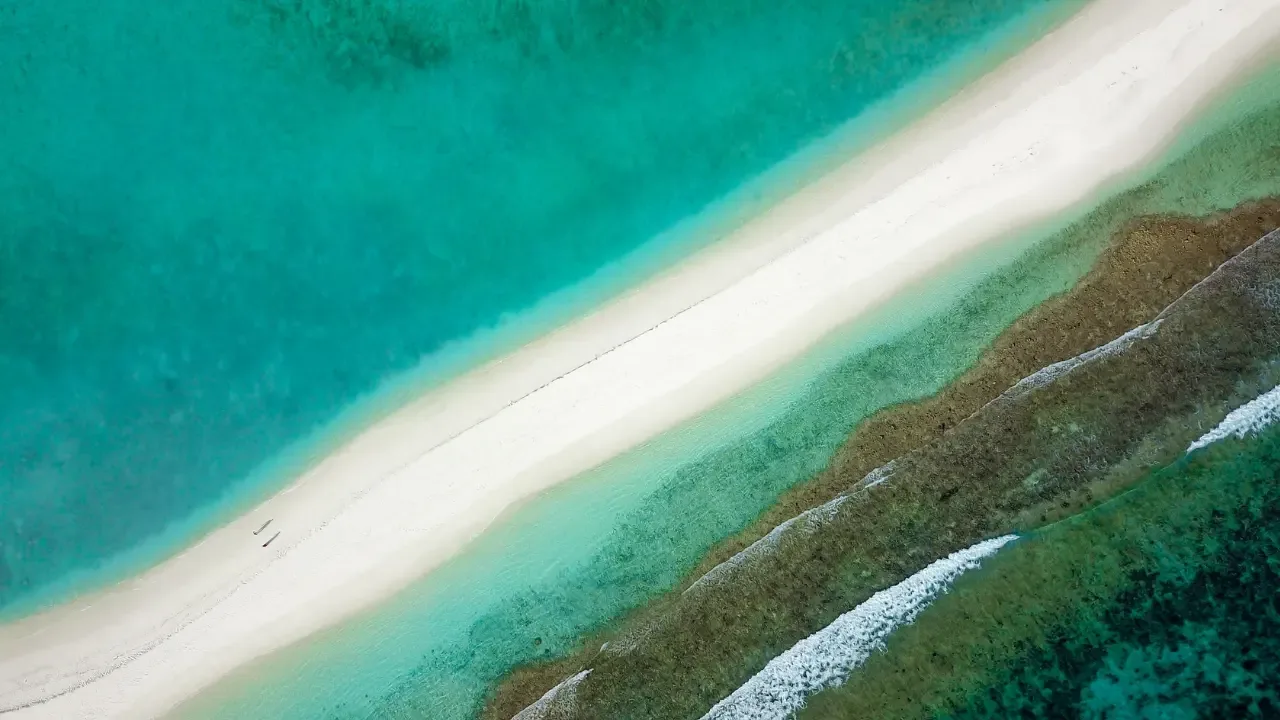
🐼 Creating a Pandas Dataframe by Appending One Row at a Time
Have you ever needed to create an empty Pandas DataFrame and then add rows to it one by one? If so, you're not alone! This is a common task that many data analysts and scientists face when working with pandas.
The Problem: Adding Rows to an Empty DataFrame
Let's say you have created an empty DataFrame like this:
df = pd.DataFrame(columns=('lib', 'qty1', 'qty2'))
And you want to add a new row to the DataFrame and fill in the values for each column. One way of doing this is by using the _set_value
method like so:
df = df._set_value(index=len(df), col='qty1', value=10.0)
This works fine if you only need to add values to one column at a time. But what if you want to add values to multiple columns simultaneously? Is there a better way to accomplish this?
The Solution: Appending Rows to the DataFrame
Yes, there is a better way! Instead of using _set_value
method repeatedly, you can use the append
method to add a new row to the DataFrame.
Here's how you can do it:
new_row = pd.Series({'lib': 'pandas', 'qty1': 10.0, 'qty2': 20.0})
df = df.append(new_row, ignore_index=True)
In this example, we first create a new row as a Pandas Series object. Each key-value pair in the dictionary represents a column name and its corresponding value. Then, we append the new_row to the DataFrame using the append
method.
The ignore_index=True
argument is important here. It ensures that the index of the new row is reset and increments by one with each appended row. Without this argument, the index will retain the values from the original DataFrame, which may lead to unexpected behavior.
Example: Adding Multiple Rows at Once
If you have multiple rows to add to the DataFrame, you can create a list of Series objects representing each row and append them all at once. Here's an example:
new_rows = [
pd.Series({'lib': 'numpy', 'qty1': 15.0, 'qty2': 25.0}),
pd.Series({'lib': 'matplotlib', 'qty1': 20.0, 'qty2': 30.0}),
pd.Series({'lib': 'seaborn', 'qty1': 5.0, 'qty2': 10.0})
]
df = df.append(new_rows, ignore_index=True)
In this case, we create a list of Pandas Series objects new_rows
, each representing a new row to be added. Then, we append the entire list to the DataFrame using the append
method.
Call-to-Action: Share Your Experience!
Creating a Pandas DataFrame by appending one row at a time is a common task that can be made simpler using the append
method. Have you encountered any challenges or found other creative solutions to this problem? Share your thoughts and experiences in the comments section below! Let's learn from each other and make our data analysis journeys more enjoyable.
Remember to follow us on Twitter @TechBlog for more exciting tech tips and tutorials! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
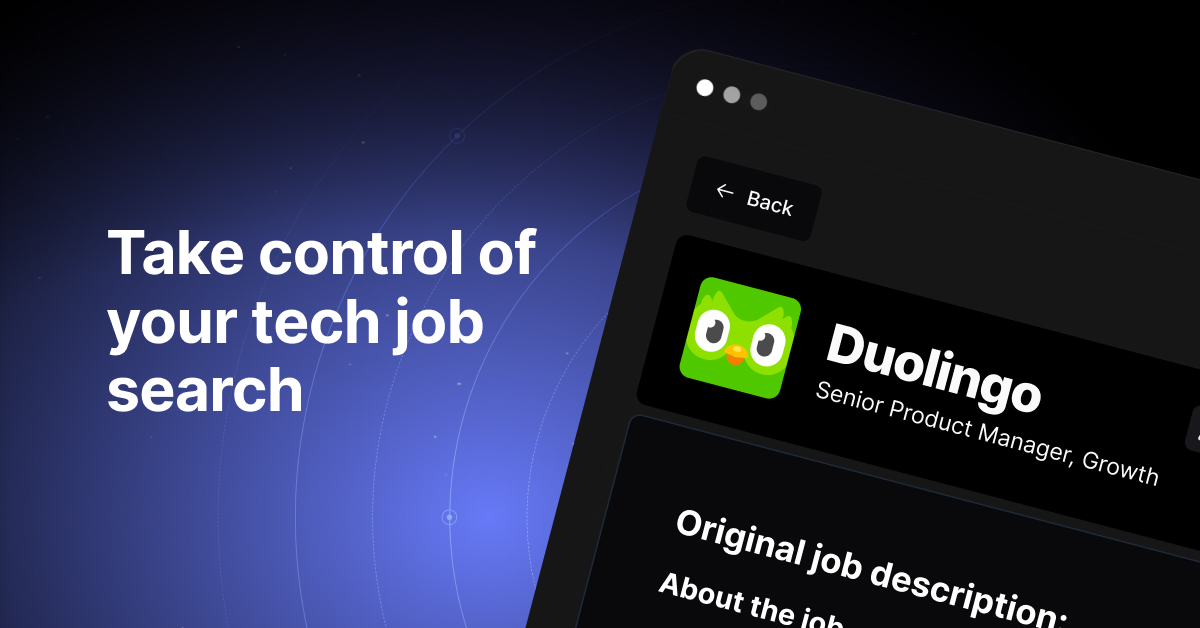