Correct way to write line to file?
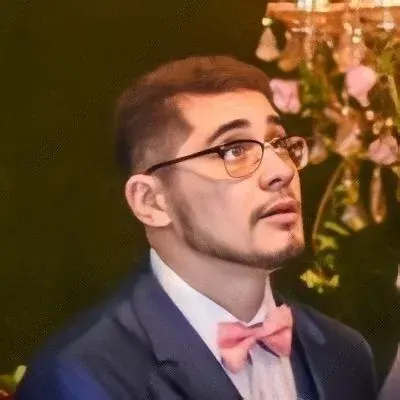
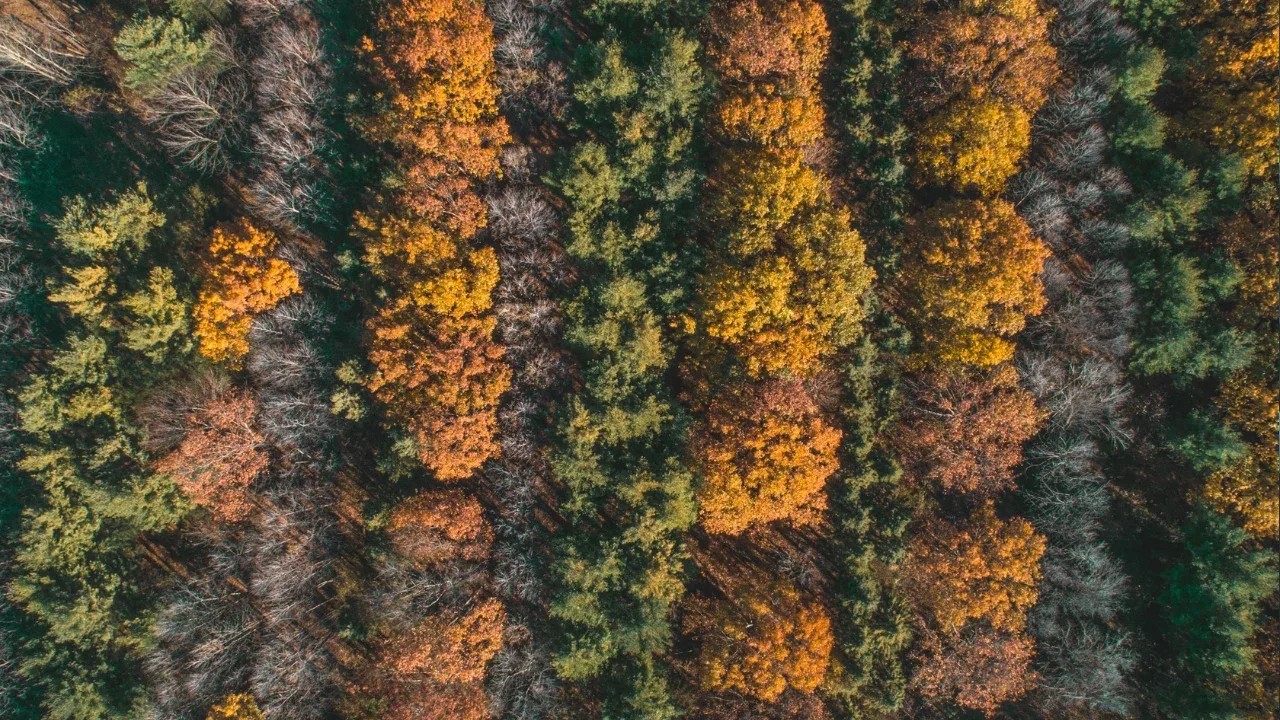
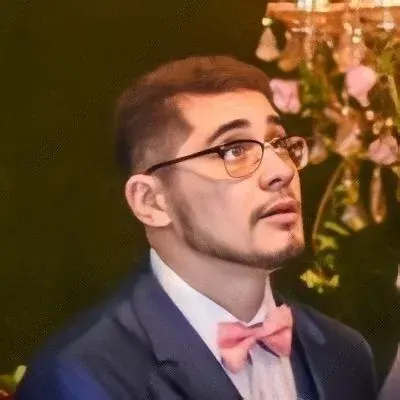
The Correct Way to Write a Line to a File in Python 🖋️📂
So, you want to write a line to a file in Python? You've come to the right place! In this blog post, we will address common issues, provide easy solutions, and guide you on the correct way to accomplish this task. Let's dive in! 💻💡
The Deprecated Way: print >>f, "hi there"
The code you mentioned, print >>f, "hi there"
, is indeed deprecated in modern Python versions. This syntax was commonly used in older versions (Python 2.x). In Python 3.x and beyond, it is no longer supported. Therefore, we need to find an alternative solution. 🚫👴🐍
The Modern Way: Using the write
Method 🆕✍️
To write a line to a file in modern Python, we'll use the write
method provided by the file object. Here's the correct syntax to achieve this:
with open("file.txt", "w") as f:
f.write("hi there\n") # Note the '\n' at the end to create a new line
In the above example, we open a file named file.txt
in write mode using the open
function. The write
method allows us to write content to the file. We simply pass the string we want to write as an argument. It's important to append the '\n'
character at the end if you want to create a new line after writing the desired text. 📝📄👌
Platform Compatibility: '\n'
or '\r\n'
? 🖥️🔄
Now, let's address the concern about line endings and platform compatibility. In Python, using '\n'
for new lines is the standard convention. It works perfectly fine on Unix-based systems, such as Linux and macOS. However, Windows uses '\r\n'
as the line ending format.
Fortunately, Python's file handling takes care of this for us automatically! When you use '\n'
as the line ending in Python, it will be translated to the appropriate format based on the operating system you are using. So, you can safely use '\n'
for line endings in your Python code, and it will work correctly across different platforms. 🌍✅
Summing It Up: The Correct Way to Write a Line to a File in Python ✔️📝
To summarize, the correct way to write a line to a file in modern Python is as follows:
with open("file.txt", "w") as f:
f.write("hi there\n")
Remember to use the write
method of the file object to write the desired content, and append '\n'
at the end if you want to create a new line. Python will automatically handle line endings and ensure platform compatibility for you. It's that simple! 🙌🎉
If you have further questions or need more information, feel free to leave a comment below. Happy coding! 💻💬
Did you find this guide helpful? Share it with your fellow Python enthusiasts and spread the knowledge! 📢🐍💡