Converting XML to JSON using Python?
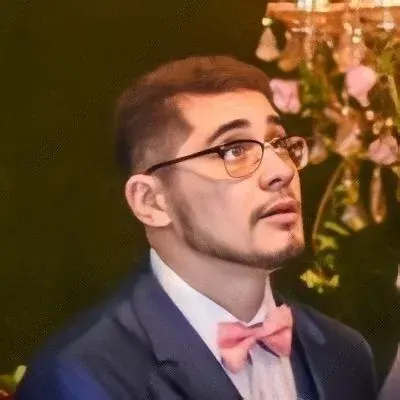
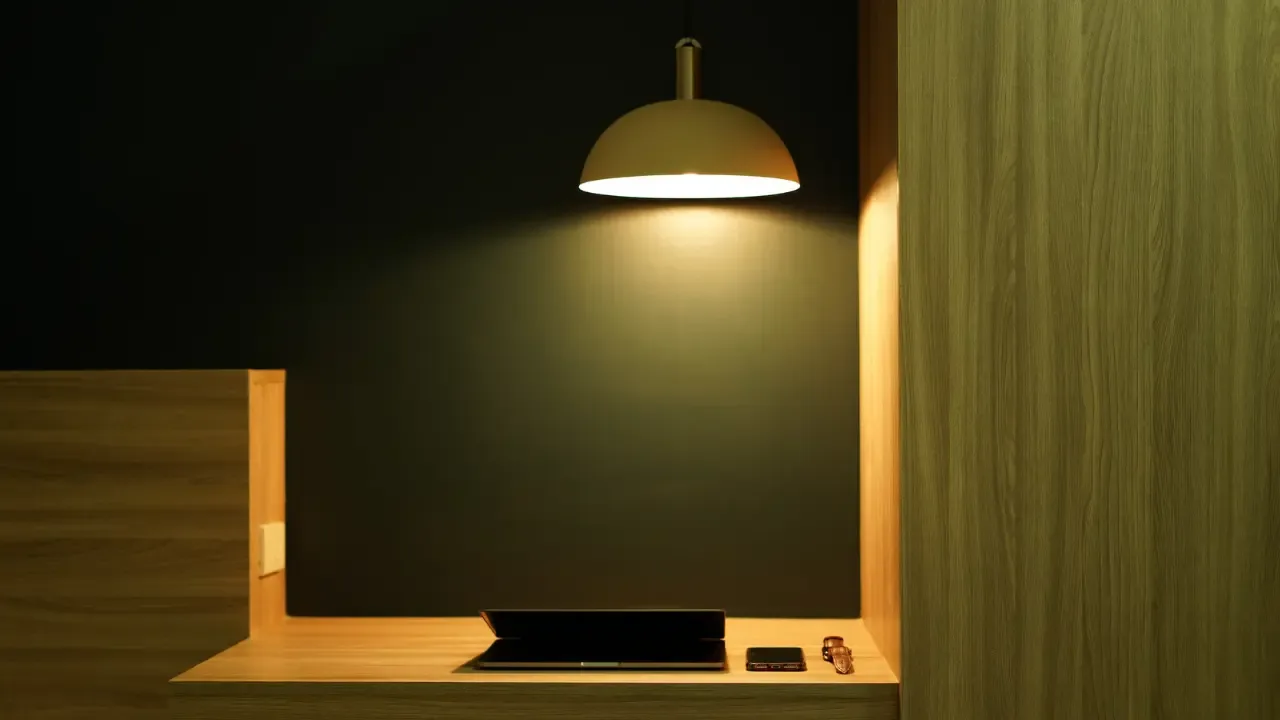
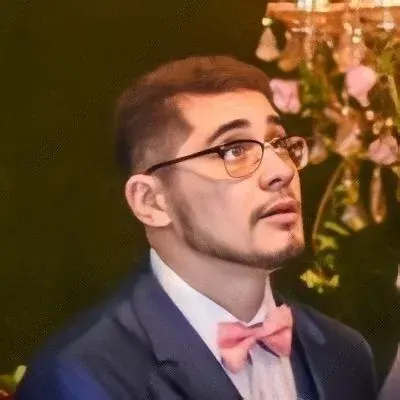
📝 Converting XML to JSON using Python: A Simple Guide
Have you ever struggled with converting XML to JSON using Python? Don't worry, you're not alone! Many developers have found themselves tangled up in ungainly code and inconclusive search results. But fear not, because we're here to help you find an easy and efficient solution.
The Scenario: Imagine you're working on a project that requires you to parse a weather feed and populate weather widgets on multiple websites. You're specifically looking for a Python-based solution to convert XML to JSON seamlessly.
The Challenge: Parsing XML and converting it to JSON can seem like a daunting task, especially if you're not familiar with the intricacies of these data formats. However, with the right tools and techniques, it can actually be a smooth process.
The Solution:
Python offers several libraries that simplify the conversion process. One such library is xmltodict
. With xmltodict
, you can effortlessly convert XML into a Python dictionary and then easily convert it to JSON format. Here's a step-by-step breakdown of how to do it:
Install
xmltodict
: Begin by installing thexmltodict
library using either pip or your preferred package manager. Open your terminal and run the following command:
pip install xmltodict
Import the necessary modules: In your Python script, import the
xmltodict
andjson
modules using the following code:
import xmltodict
import json
Read the XML file: Assuming you have an XML file that needs to be converted, use the
open()
function to read it. Here's an example:
with open('weather_feed.xml', 'r') as file:
xml_data = file.read()
Convert XML to JSON: Use
xmltodict.parse()
to convert the XML data into a Python dictionary. Then, utilizejson.dumps()
to convert the dictionary to a JSON string. Here's an example:
xml_dict = xmltodict.parse(xml_data)
json_data = json.dumps(xml_dict)
Write the JSON data: If you want to save the JSON information to a file, use the
open()
function again, but this time with the'w'
mode. Here's an example:
with open('weather_feed.json', 'w') as file:
file.write(json_data)
And voila! You have successfully converted XML to JSON using Python.
The Call-to-Action: We hope this guide has helped you understand how to convert XML to JSON using Python. If you found it useful, share it with your fellow developers and tech enthusiasts. Let's make XML to JSON conversion a breeze for everyone! 💪🚀
Do you have any other programming challenges or questions? Let us know in the comments below, and we'll be more than happy to help you find a solution! 😊✨