Converting from a string to boolean in Python
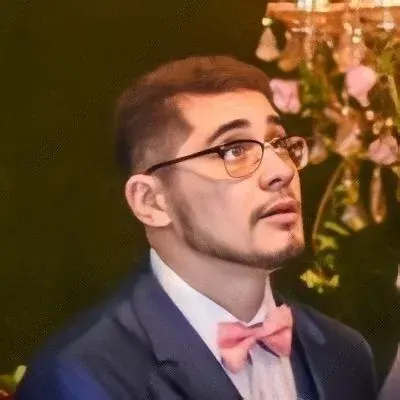
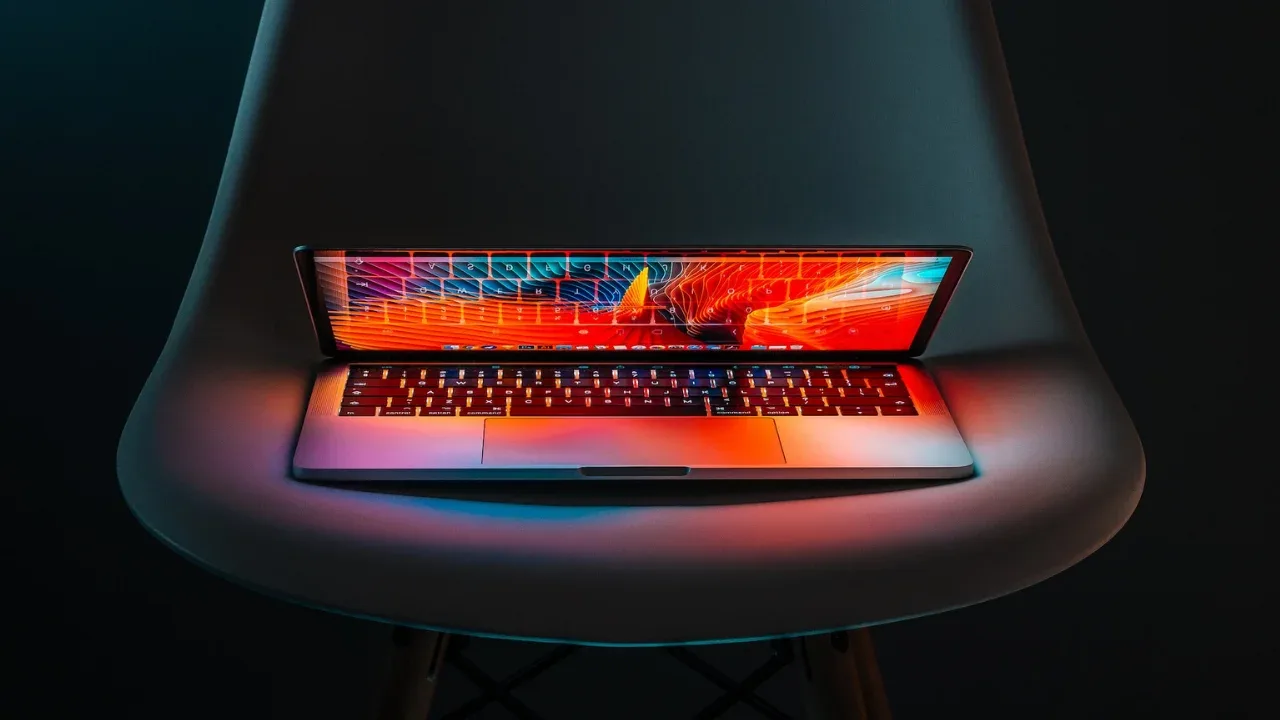
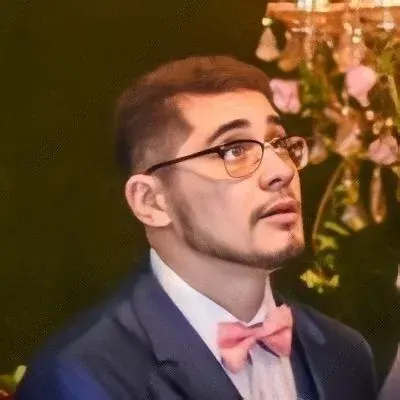
Title: Converting from a string to boolean in Python: Unmasking the Truth! 🎭
Hey there Pythonistas! 👋
Today, we're diving into the fascinating world of string-to-boolean conversion in Python. 🚀 Have you ever encountered a situation where you needed to transform a string into a boolean value, but things didn't quite go as expected? Fear not! We've got your back! Let's untangle this mystery. 🧐
The Problem: 💔
So, we've stumbled upon an intriguing case. Consider the following code snippet:
>>> bool("False")
True
Whoa! That's not what we were expecting, right? 😱 The string "False"
doesn't exactly scream "truth" to us. But Python seems to have other ideas! 😅
Why This Happens: 🤔
The truth is, Python's bool()
function doesn't perform string matching. Instead, it evaluates the non-empty nature of a string. In this case, since "False" is not an empty string, bool("False")
returns True
. Isn't that surprising? 😅
A Simple Solution: ✔️
Thankfully, we have an elegant solution for this conundrum. We can use the ast
(Abstract Syntax Trees) module to evaluate string literals properly. Here's how you can do it:
import ast
def string_to_boolean(string):
return ast.literal_eval(string.lower())
result = string_to_boolean("False")
print(result) # Output: False
Voila! 👏 With the help of ast.literal_eval()
and a lowercase string, you can now convert the string to its boolean counterpart correctly. Our previous code snippet now gives us the desired outcome of False
.
Going Beyond - Handling Strings: 🌟
But wait, what about other strings like "true," "yes," or "no"? Let's expand our solution to handle those as well. Here's an improved version of our string_to_boolean()
function:
def string_to_boolean(string):
if string.lower() in ["true", "yes"]:
return True
elif string.lower() in ["false", "no"]:
return False
else:
raise ValueError(f"Invalid input: {string}")
Using a simple if-else construct, we can now handle a broader range of string inputs. If the input matches any of the recognized string representations of True or False, we return the corresponding boolean value. Otherwise, we raise a ValueError
to handle unexpected inputs.
Contribute and Share Your Wisdom: 📚
Have you encountered any other approaches to this problem? We'd love to hear about them! Share your unique insights, alternate solutions, or even ask questions in the comments section below. Let's brainstorm and learn from each other! 💡🤝
Keep coding, keep exploring! 🚀✨ Happy Pythoning! 🐍💻
P.S. Don't forget to follow our blog for more tech tips and tricks! 😉🔖