Converting dictionary to JSON
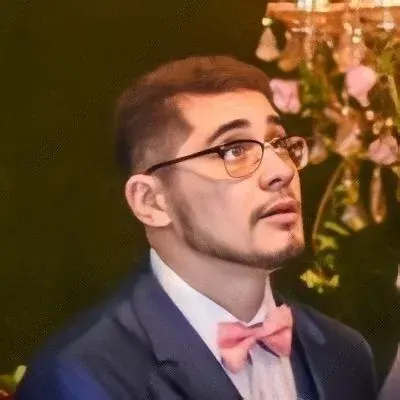
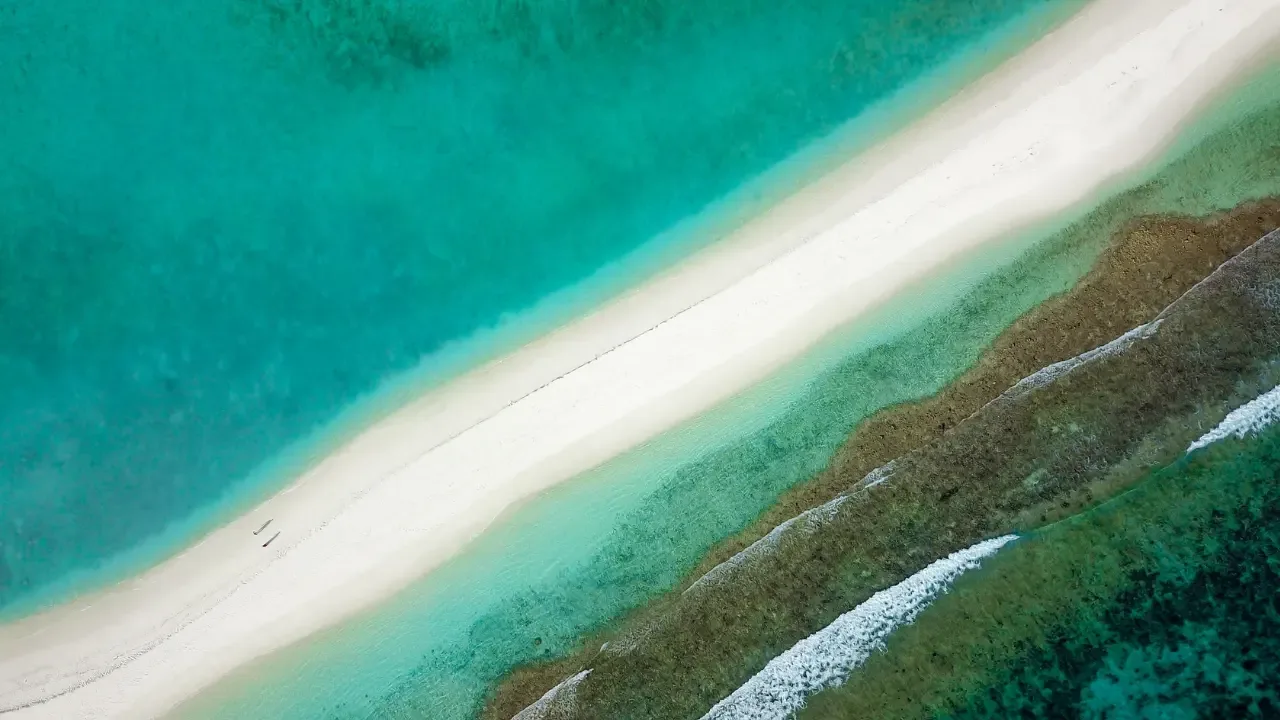
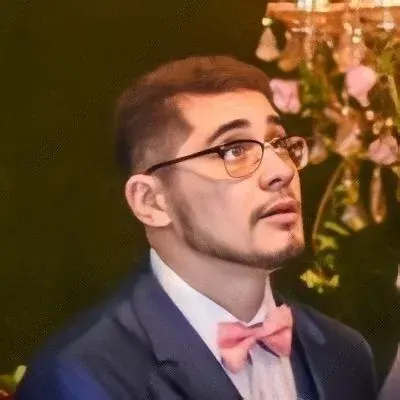
Converting Dictionary to JSON: Easy Solutions for Common Issues
So, you're trying to convert a dictionary to JSON? It sounds like a simple task, but sometimes things can go wrong. Don't worry, we're here to help you understand what might be happening and provide easy solutions to solve these issues. Let's dive in and untangle the problem!
The Problem: "TypeError: string indices must be integers, not str"
You may have encountered the above error message when trying to access your data in the JSON file. The error message is pointing out that you are trying to use a string as an index instead of an integer.
Here's the code snippet that raises the error:
r = {'is_claimed': 'True', 'rating': 3.5}
r = json.dumps(r)
file.write(str(r['rating']))
Understanding the Issue
The problem arises when you convert your dictionary r
to JSON using the json.dumps()
function. This function converts the dictionary into a string representation of JSON, which means you can no longer access it using string keys.
The Solution
To solve this issue, you need to convert your JSON string back to a dictionary and then access the data using the appropriate key. Here's how you can do it:
import json
r = {'is_claimed': 'True', 'rating': 3.5}
r = json.dumps(r)
r = json.loads(r) # Convert JSON string back to dictionary
file.write(str(r['rating']))
With this solution, you first convert your dictionary r
to a JSON string using json.dumps()
. Then, using json.loads()
, you convert the JSON string back to a dictionary, allowing you to access your data using string keys like 'rating'
.
Tip: Simplify Your Code
You might find the code above a bit cumbersome, as it involves multiple conversions. To simplify it, you can directly write your dictionary to a JSON file without converting it back and forth. Here's an example:
import json
r = {'is_claimed': 'True', 'rating': 3.5}
with open('data.json', 'w') as file:
json.dump(r, file)
This code snippet writes the dictionary r
directly to a JSON file named 'data.json'
using json.dump()
. By doing so, you avoid any unnecessary conversions and make your code more concise and efficient.
Get Your Data Safely!
By following the solutions we've provided, you should now be able to convert your dictionary to JSON without encountering any issues. Remember to adapt the solutions to your specific case and use the appropriate functions to access your data in the desired format.
If you found this guide helpful, or if you have any other questions or cool Python tips to share, leave a comment below! Let's keep learning together. 🚀