Converting between datetime, Timestamp and datetime64
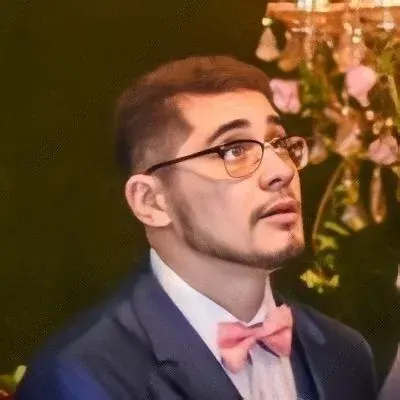
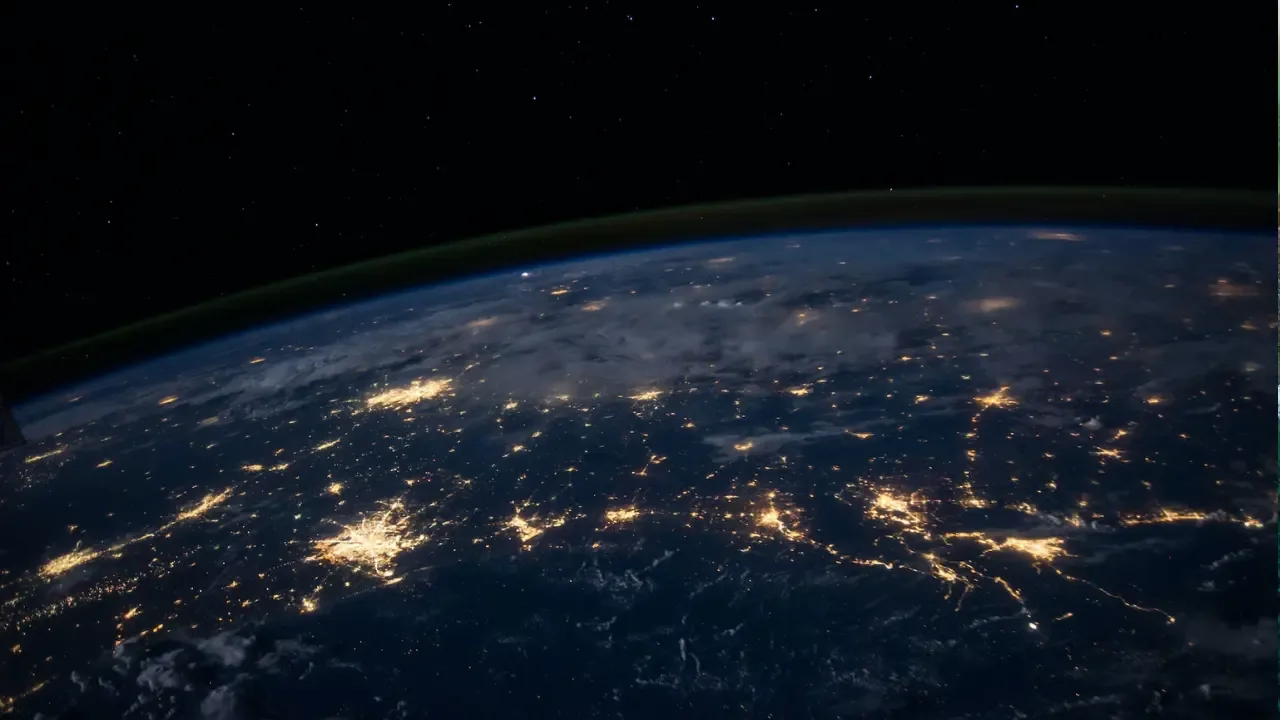
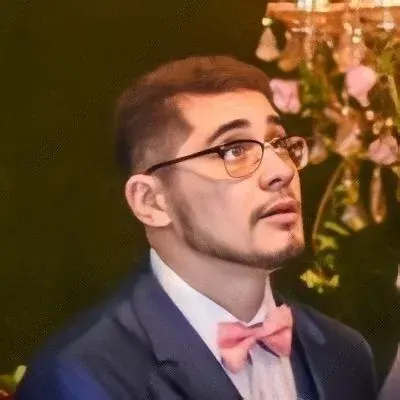
Converting Between DateTime, Timestamp, and DateTime64: A Complete Guide
Are you struggling with converting datetime, Timestamp, and datetime64 objects? Don't worry, we've got you covered! In this guide, we'll address common issues and provide easy solutions to help you with your conversion problems. So let's dive in and unravel the mysteries of datetime conversions!
The Setup
To understand how to convert between datetime, Timestamp, and datetime64 objects, let's first set up the scenario. In the given code snippet, we create three objects:
import datetime
import numpy as np
import pandas as pd
dt = datetime.datetime(2012, 5, 1)
ts = pd.DatetimeIndex([dt])[0]
dt64 = np.datetime64(dt)
dt
: A regular datetime object with the date set to May 1, 2012.ts
: A Timestamp object created from the datetime object.dt64
: A datetime64 object created from the datetime object.
This is our starting point. Now, let's see how we can perform the conversions!
Converting datetime64 to datetime
One common requirement is to convert a numpy.datetime64
object (dt64
) to a datetime.datetime
object. Thankfully, this conversion is relatively straightforward. To convert dt64
to a datetime
object, you can use the to_datetime()
method provided by pandas:
dt_from_dt64 = pd.to_datetime(dt64)
And there you have it! You have successfully converted the dt64
object to a datetime
object.
Converting datetime64 to Timestamp
What if you need to convert dt64
to a Timestamp
object instead? Again, pandas comes to the rescue! You can utilize the Timestamp()
function provided by pandas:
ts_from_dt64 = pd.Timestamp(dt64)
And just like that, your dt64
object is now a Timestamp
object.
Handling Edge Cases
Sometimes, you might encounter unusual examples that require a bit of extra care. Let's consider the following example in the dataset:
dt64 = numpy.datetime64('2002-06-28T01:00:00.000000000+0100')
In this case, you might expect the equivalent datetime object to be datetime.datetime(2002, 6, 28, 1, 0)
. However, the conversion might give you a large integer value.
To handle this, you can still use the same conversion techniques mentioned earlier. Here's an example using the to_datetime()
method:
dt_from_dt64_edge_case = pd.to_datetime(dt64)
By applying this conversion, you can obtain the correct datetime
object for your edge case scenario.
🚀 Take Your Conversions to the Next Level!
Now that you have learned how to convert between datetime, Timestamp, and datetime64 objects, it's time to put your skills into practice! Experiment with various conversions and explore the possibilities.
Remember, practice makes perfect, and the more you work with these conversions, the more confident you'll become in handling datetime objects in your projects.
Let us know in the comments below if you encountered any specific conversion challenges or if you have any other tips to share. We would love to hear from you!