Converting a Pandas GroupBy output from Series to DataFrame
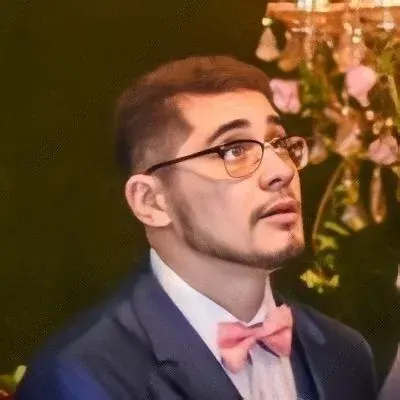
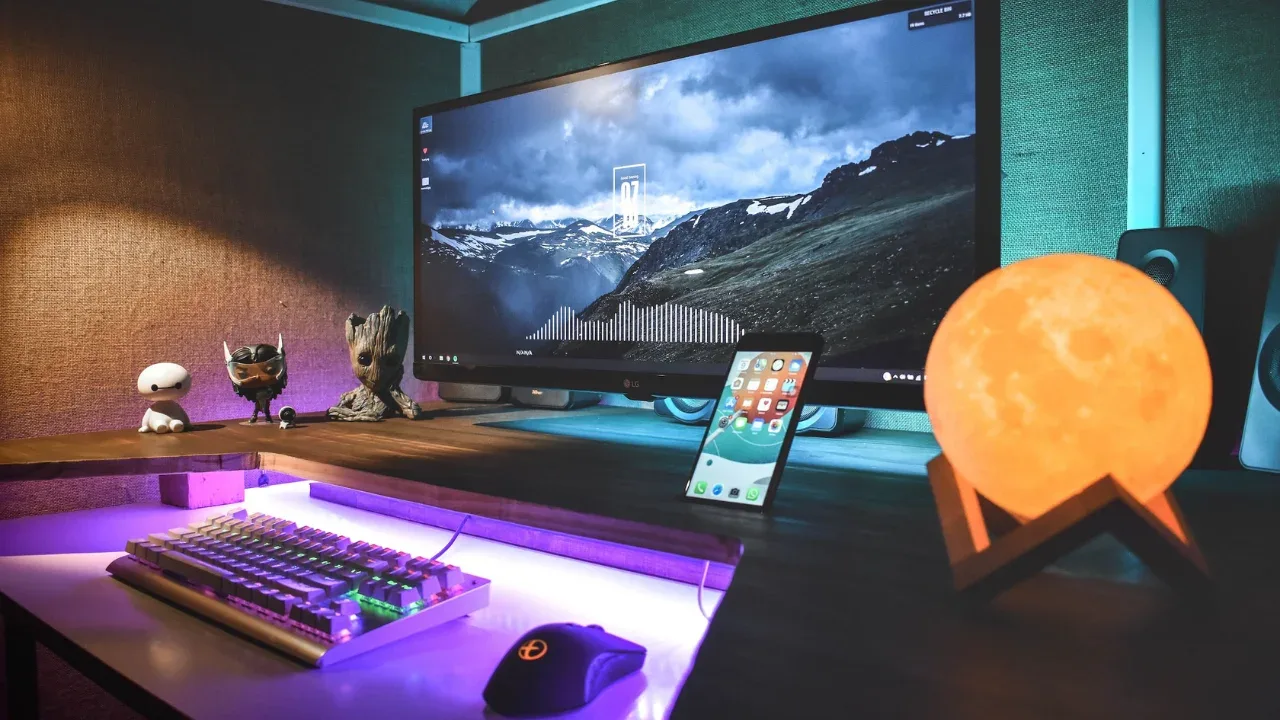
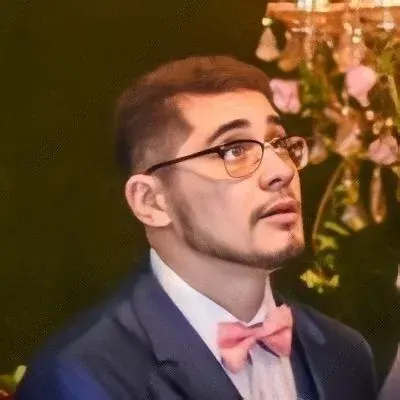
Converting a Pandas GroupBy Output from Series to DataFrame: An Easy Guide
Are you struggling to convert a Pandas GroupBy output from Series to DataFrame? Don't worry, you're not alone! Many data analysts and scientists encounter this issue while working with pandas. In this blog post, we will address this common problem and provide you with easy solutions to get the desired DataFrame result.
Understanding the Problem
Let's start by understanding the problem with a real-world example. Imagine you have a DataFrame df1
with two columns - "Name" and "City" - and you want to group the data based on these columns. Starting with the following input data:
df1 = pandas.DataFrame( {
"Name" : ["Alice", "Bob", "Mallory", "Mallory", "Bob" , "Mallory"] ,
"City" : ["Seattle", "Seattle", "Portland", "Seattle", "Seattle", "Portland"] } )
The dataframe looks like this:
City Name
0 Seattle Alice
1 Seattle Bob
2 Portland Mallory
3 Seattle Mallory
4 Seattle Bob
5 Portland Mallory
To group the data by "Name" and "City," you can use the groupby
function:
g1 = df1.groupby(['Name', 'City']).count()
This groups the data and returns a GroupBy object:
City Name
Name City
Alice Seattle 1 1
Bob Seattle 2 2
Mallory Portland 2 2
Seattle 1 1
However, the goal is to convert this GroupBy object into a DataFrame that includes all the rows in the GroupBy object.
Solution: Resetting the Index
To convert the GroupBy output into a DataFrame and include all the rows, you need to reset the index of the GroupBy object.
g1.reset_index(inplace=True)
This modifies the existing GroupBy object and resets the index, resulting in the desired DataFrame:
Name City City Name
0 Alice Seattle 1 1
1 Bob Seattle 2 2
2 Mallory Portland 2 2
3 Mallory Seattle 1 1
Wrapping Up
Converting a Pandas GroupBy output from Series to DataFrame may initially seem challenging, but with the right approach, it becomes a straightforward task. By resetting the index of the GroupBy object, you can transform it into a DataFrame that includes all the rows.
Next time you come across this issue, remember to use the reset_index
method. It will save you time and frustration. Happy data wrangling!
Do you have any other pandas-related questions or topics you'd like us to cover? Let us know in the comments below! 👇🤔
Example code sourced from the original question on Stack Overflow.