Convert string "Jun 1 2005 1:33PM" into datetime
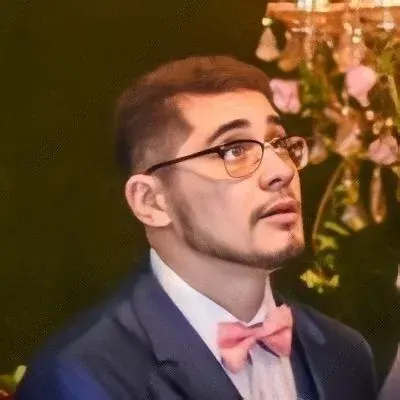
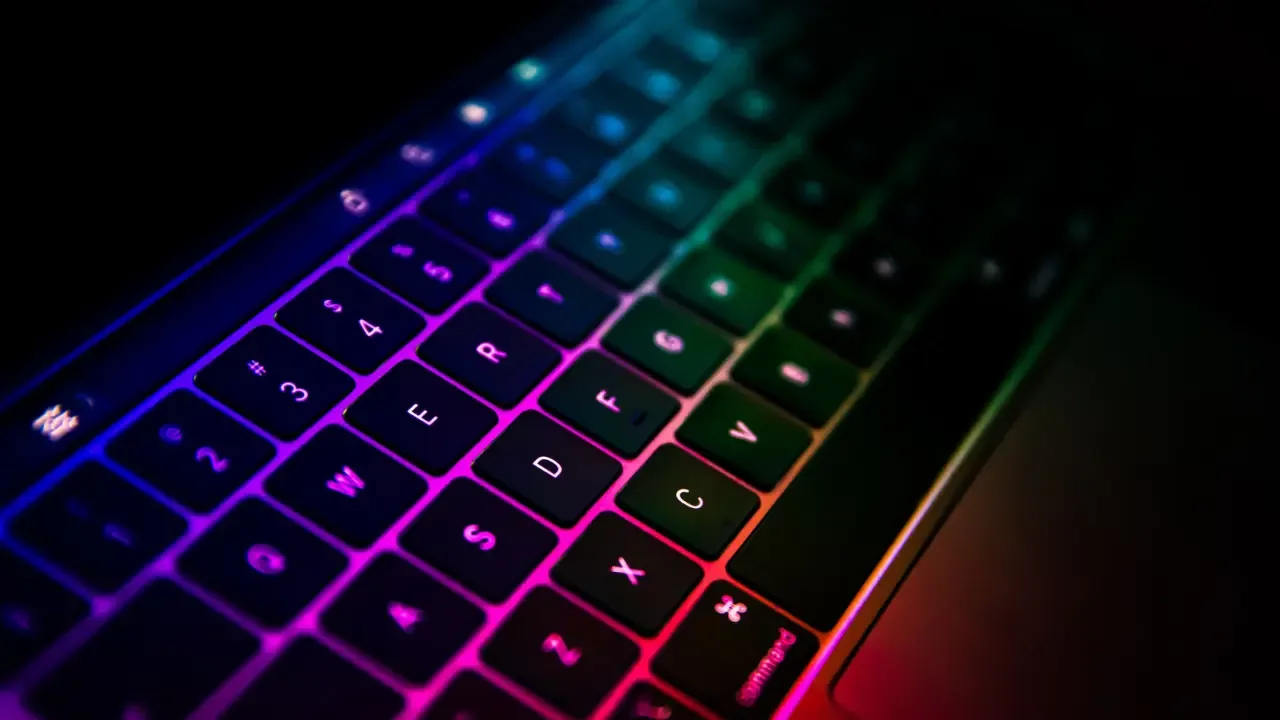
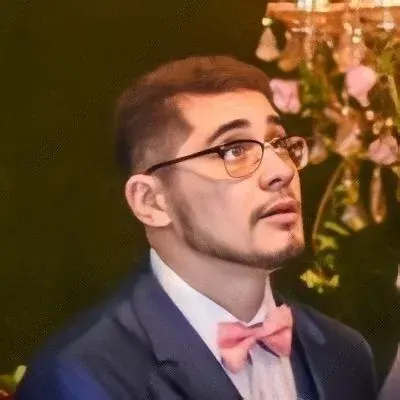
Converting String "Jun 1 2005 1:33PM" into Datetime 💫
Are you struggling to convert datetime strings into datetime objects in Python? Don't worry, you're not alone! Many developers find this a common challenge when dealing with different date and time formats. But fear not, because in this guide, I'll walk you through the process step-by-step and provide you with easy solutions to help you convert the string "Jun 1 2005 1:33PM" into a datetime object. Let's dive right in! 🚀
The Challenge ⚠️
So you have a list of datetime strings, and your goal is to convert them into datetime objects. In our case, the datetime string we want to convert is "Jun 1 2005 1:33PM". The challenge lies in the fact that datetime strings can have various formats, making it tricky to convert them directly into datetime objects.
The Solution 💡
To successfully convert the datetime string into a datetime object, we need to follow these steps:
Import the datetime module from the datetime library in Python.
from datetime import datetime
Use the
strptime
function to parse the datetime string according to its format.datetime_object = datetime.strptime("Jun 1 2005 1:33PM", "%b %d %Y %I:%M%p")
Here, we specify the format of the datetime string using the formatting codes:
%b
represents the abbreviated month name (e.g., Jun)%d
represents the day of the month (e.g., 1)%Y
represents the four-digit year (e.g., 2005)%I
represents the hour in 12-hour format (e.g., 1)%M
represents the minutes (e.g., 33)%p
represents either AM or PM (e.g., PM)
Voila! Now we have successfully converted the datetime string into a datetime object. 🎉
Putting It All Together 🛠️
Let's see how this solution can be applied to your list of datetime strings:
from datetime import datetime
datetime_strings = ["Jun 1 2005 1:33PM", "Aug 28 1999 12:00AM"]
datetime_objects = []
for datetime_str in datetime_strings:
datetime_object = datetime.strptime(datetime_str, "%b %d %Y %I:%M%p")
datetime_objects.append(datetime_object)
print(datetime_objects)
By iterating through each datetime string in the list, applying the conversion, and storing the resulting datetime objects in a new list, we can now access and work with the converted datetime values.
Conclusion 🎯
Converting datetime strings into datetime objects may initially seem daunting due to the variations in date formats. However, by utilizing the strptime
function from the datetime module, we can tackle this challenge head-on. Remember to specify the correct formatting codes to match the structure of your datetime string.
Now that you have the knowledge and tools to convert datetime strings into datetime objects, go ahead and give it a try in your own projects! Happy coding! 😄
Have you ever encountered any struggles with datetime conversions? Share your experiences and tips in the comments below! Let's help each other overcome these challenges! 💪💬