Convert Python dict into a dataframe
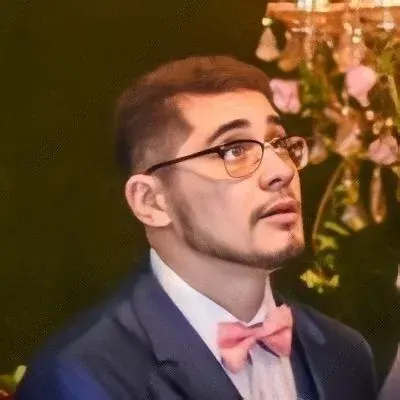
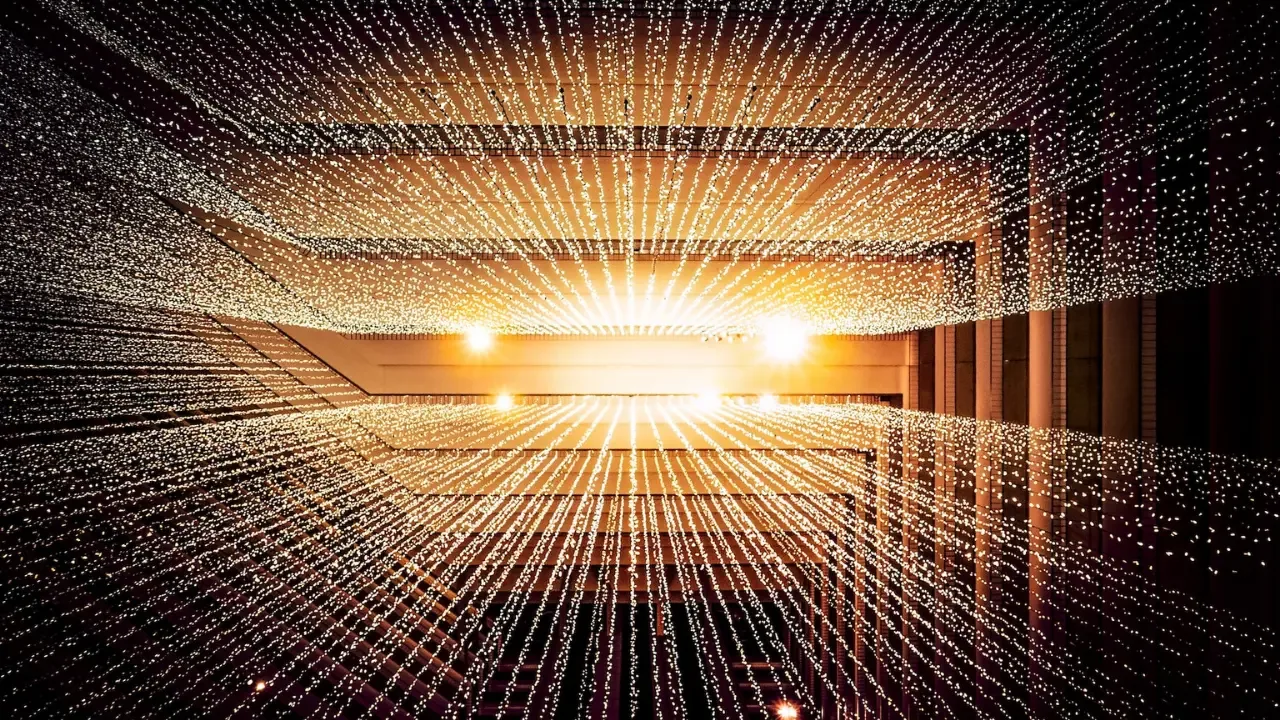
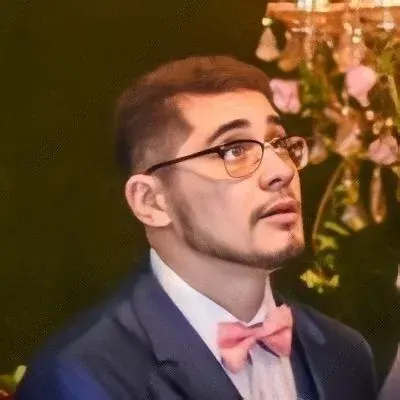
Converting a Python Dict into a DataFrame in Pandas
Are you trying to convert a Python dictionary into a DataFrame in Pandas, but having trouble figuring out the best way to do it? Don't worry, you're not alone! Converting dictionaries into DataFrames can sometimes be a bit tricky, especially when trying to maintain the relationship between the keys and values.
In this blog post, we'll explore some common issues when converting a Python dictionary into a DataFrame and provide easy solutions to help you achieve your goal. So, let's dive in and find the most direct way to solve this problem!
The Problem
One user had a Python dictionary with Unicode dates as keys and integer values. They wanted to convert this dictionary into a Pandas DataFrame, with the dates and their corresponding values as two separate columns.
To better illustrate the problem, here's the example dictionary given:
{u'2012-06-08': 388,
u'2012-06-09': 388,
u'2012-06-10': 388,
u'2012-06-11': 389,
u'2012-06-12': 389,
u'2012-06-13': 389,
u'2012-06-14': 389,
u'2012-06-15': 389,
u'2012-06-16': 389,
u'2012-06-17': 389,
u'2012-06-18': 390,
u'2012-06-19': 390,
u'2012-06-20': 390,
u'2012-06-21': 390,
u'2012-06-22': 390,
u'2012-06-23': 390,
u'2012-06-24': 390,
u'2012-06-25': 391,
u'2012-06-26': 391,
u'2012-06-27': 391,
u'2012-06-28': 391,
u'2012-06-29': 391,
u'2012-06-30': 391,
u'2012-07-01': 391,
u'2012-07-02': 392,
u'2012-07-03': 392,
u'2012-07-04': 392,
u'2012-07-05': 392,
u'2012-07-06': 392}
The Solution
While one possible solution involves converting each key-value pair into a dictionary within the original dictionary structure and then adding each row individually to the DataFrame, it might be inefficient and cumbersome. Luckily, there's a simpler and more direct approach!
Step 1: Import the Necessary Libraries
The first step is to import the required libraries, which in this case are Pandas and NumPy. Open your Python interpreter or script and add the following lines:
import pandas as pd
Step 2: Create a DataFrame from the Dictionary
To convert the dictionary into a DataFrame, we can use the pd.DataFrame.from_dict()
method. This method accepts the dictionary as its first argument and the orientation as the second argument. In our case, we want the dictionary keys to be the column "Date" and the values as the column "DateValue".
Here's the code to convert the dictionary into the desired DataFrame:
my_dict = {
u'2012-06-08': 388,
u'2012-06-09': 388,
u'2012-06-10': 388,
...
}
df = pd.DataFrame.from_dict(my_dict, orient='index', columns=['DateValue'])
df.index.name = 'Date'
Let's break down what's happening in this code snippet:
pd.DataFrame.from_dict(my_dict, orient='index')
creates a DataFrame from the dictionary using the dictionary keys as the DataFrame index.columns=['DateValue']
sets the column name as "DateValue".df.index.name = 'Date'
sets the index name as "Date", which matches the desired output as stated in the question.
Step 3: Check the Result
To verify whether the DataFrame has been created correctly, you can use the df.head()
method to display the first few rows of the DataFrame:
print(df.head())
The output should look like this:
DateValue
Date
2012-06-08 388
2012-06-09 388
2012-06-10 388
2012-06-11 389
2012-06-12 389